
- Entity Framework Tutorial
- Entity Framework - Home
- Entity Framework - Overview
- Entity Framework - Architecture
- Entity F - Environment Setup
- Entity Framework - Database Setup
- Entity Framework - Data Model
- Entity Framework - DbContext
- Entity Framework - Types
- Entity Framework - Relationships
- Entity Framework - Lifecycle
- Entity F - Code First Approach
- Entity F - Model First Approach
- Entity F - Database First Approach
- Entity Framework - DEV Approaches
- Entity F - Database Operations
- Entity Framework - Concurrency
- Entity Framework - Transaction
- Entity Framework - Views
- Entity Framework - Index
- Entity F - Stored Procedures
- Entity F - Disconnected Entities
- Entity F - Table-Valued Function
- Entity Framework - Native SQL
- Entity Framework - Enum Support
- Entity F - Asynchronous Query
- Entity Framework - Persistence
- Entity F - Projection Queries
- Entity F - Command Logging
- Entity F - Command Interception
- Entity Framework - Spatial Data Type
- Entity Framework - Inheritance
- Entity Framework - Migration
- Entity Framework - Eager Loading
- Entity Framework - Lazy Loading
- Entity Framework - Explicit Loading
- Entity Framework - Validation
- Entity Framework - Track Changes
- Entity Framework - Colored Entities
- Entity F - Code First Approach
- Entity Framework - First Example
- Entity Framework - Data Annotations
- Entity Framework - Fluent API
- Entity Framework - Seed Database
- Entity F - Code First Migration
- Entity F - Multiple DbContext
- Entity F - Nested Entity Types
- Entity Framework Resources
- Entity Framework - Quick Guide
- Entity Framework - Useful Resources
- Entity Framework - Discussion
Entity Framework - Types
In Entity Framework, there are two types of entities that allow developers to use their own custom data classes together with data model without making any modifications to the data classes themselves.
- POCO entities
- Dynamic Proxy
POCO Entities
POCO stands for "plain-old" CLR objects which can be used as existing domain objects with your data model.
POCO data classes which are mapped to entities are defined in a data model.
It also supports most of the same query, insert, update, and delete behaviors as entity types that are generated by the Entity Data Model tools.
You can use the POCO template to generate persistence-ignorant entity types from a conceptual model.
Let’s take a look at the following example of Conceptual Entity Data Model.
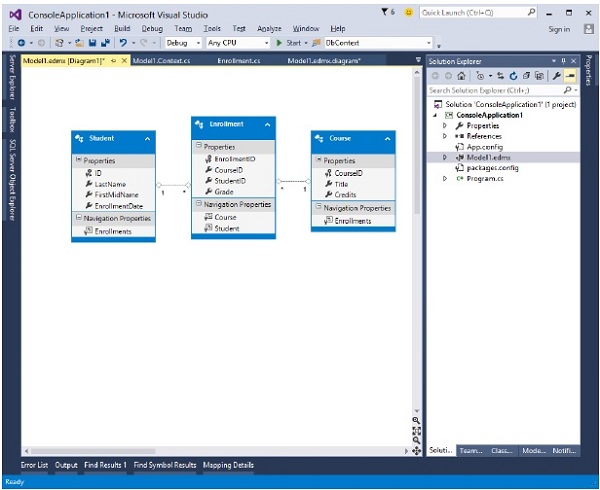
To generate POCO entities for the above Entity model −
Step 1 − Right click on the designer window. It will display the following dialog.
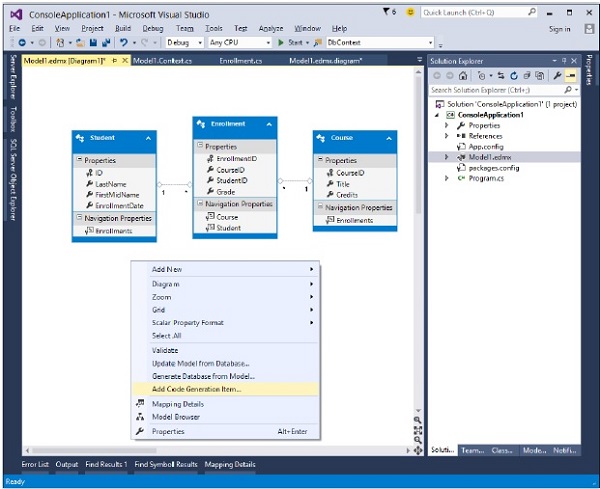
Step 2 − Select the Add Code Generation Item...
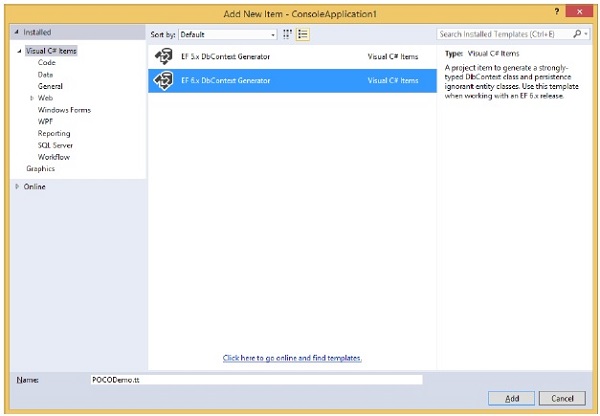
Step 3 − Select the EF 6.x DbContext Generator, write name and then click Add button.
You will see in your solution explorer that POCODemo.Context.tt and POCODemo.tt templates are generated.
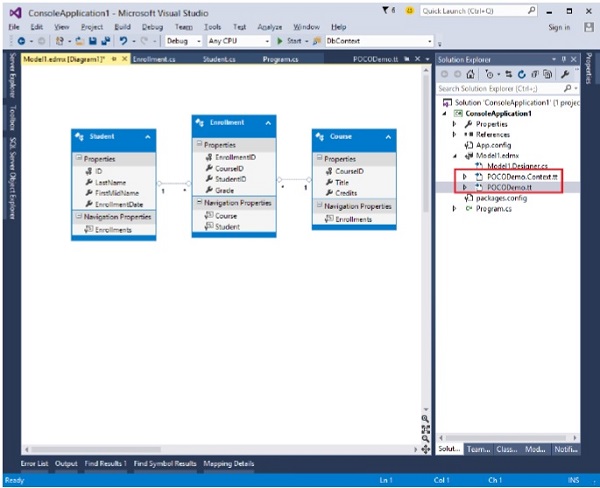
The POCODemo.Context generates the DbContext and the object sets that you can return and use for querying, say for context, Students and Courses, etc.
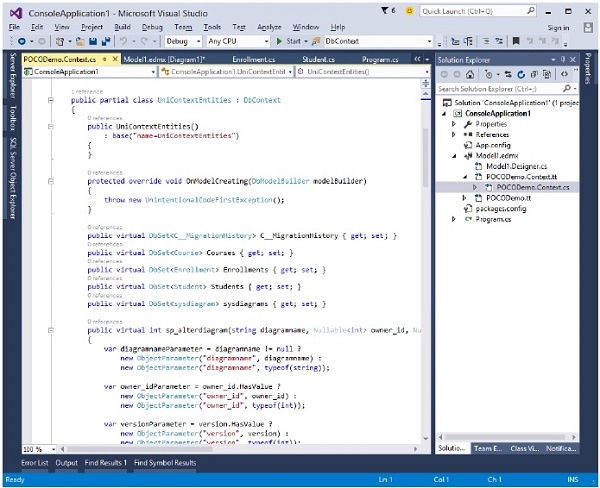
The other template deals with all the types Student, Courses, etc. Following is the code for Student class which is generated automatically from the Entity Model.
namespace ConsoleApplication1 { using System; using System.Collections.Generic; public partial class Student { [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] public Student() { this.Enrollments = new HashSet<Enrollment>(); } public int ID { get; set; } public string LastName { get; set; } public string FirstMidName { get; set; } public System.DateTime EnrollmentDate { get; set; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", CA2227:CollectionPropertiesShouldBeReadOnly")] public virtual ICollection<Enrollment> Enrollments { get; set; } } }
Similar classes are generated for Course and Enrollment tables from the Entity Model.
Dynamic Proxy
When creating instances of POCO entity types, the Entity Framework often creates instances of a dynamically generated derived type that acts as a proxy for the entity. IT can also be said that it is a runtime proxy classes like a wrapper class of POCO entity.
You can override some properties of the entity for performing actions automatically when the property is accessed.
This mechanism is used to support lazy loading of relationships and automatic change tracking.
This technique also applies to those models which are created with Code First and EF Designer.
If you want the Entity Framework to support lazy loading of the related objects and to track changes in POCO classes, then the POCO classes must meet the following requirements −
Custom data class must be declared with public access.
Custom data class must not be sealed.
Custom data class must not be abstract.
Custom data class must have a public or protected constructor that does not have parameters.
Use a protected constructor without parameters if you want the CreateObject method to be used to create a proxy for the POCO entity.
Calling the CreateObject method does not guarantee the creation of the proxy: the POCO class must follow the other requirements that are described in this topic.
The class cannot implement the IEntityWithChangeTracker or IEntityWithRelationships interfaces because the proxy classes implement these interfaces.
The ProxyCreationEnabled option must be set to true.
The following example is of dynamic proxy entity class.
public partial class Course { public Course() { this.Enrollments = new HashSet<Enrollment>(); } public int CourseID { get; set; } public string Title { get; set; } public int Credits { get; set; } public virtual ICollection<Enrollment> Enrollments { get; set; } }
To disable creating proxy objects, set the value of the ProxyCreationEnabled property to false.
To Continue Learning Please Login