
- Entity Framework Tutorial
- Entity Framework - Home
- Entity Framework - Overview
- Entity Framework - Architecture
- Entity F - Environment Setup
- Entity Framework - Database Setup
- Entity Framework - Data Model
- Entity Framework - DbContext
- Entity Framework - Types
- Entity Framework - Relationships
- Entity Framework - Lifecycle
- Entity F - Code First Approach
- Entity F - Model First Approach
- Entity F - Database First Approach
- Entity Framework - DEV Approaches
- Entity F - Database Operations
- Entity Framework - Concurrency
- Entity Framework - Transaction
- Entity Framework - Views
- Entity Framework - Index
- Entity F - Stored Procedures
- Entity F - Disconnected Entities
- Entity F - Table-Valued Function
- Entity Framework - Native SQL
- Entity Framework - Enum Support
- Entity F - Asynchronous Query
- Entity Framework - Persistence
- Entity F - Projection Queries
- Entity F - Command Logging
- Entity F - Command Interception
- Entity Framework - Spatial Data Type
- Entity Framework - Inheritance
- Entity Framework - Migration
- Entity Framework - Eager Loading
- Entity Framework - Lazy Loading
- Entity Framework - Explicit Loading
- Entity Framework - Validation
- Entity Framework - Track Changes
- Entity Framework - Colored Entities
- Entity F - Code First Approach
- Entity Framework - First Example
- Entity Framework - Data Annotations
- Entity Framework - Fluent API
- Entity Framework - Seed Database
- Entity F - Code First Migration
- Entity F - Multiple DbContext
- Entity F - Nested Entity Types
- Entity Framework Resources
- Entity Framework - Quick Guide
- Entity Framework - Useful Resources
- Entity Framework - Discussion
Entity Framework - Migration
In Entity Framework 5 and previous versions of Entity Framework, the code was split between core libraries (primarily System.Data.Entity.dll) shipped as part of the .NET Framework, and the additional libraries (primarily EntityFramework.dll) was distributed and shipped using NuGet as shown in the following diagram.
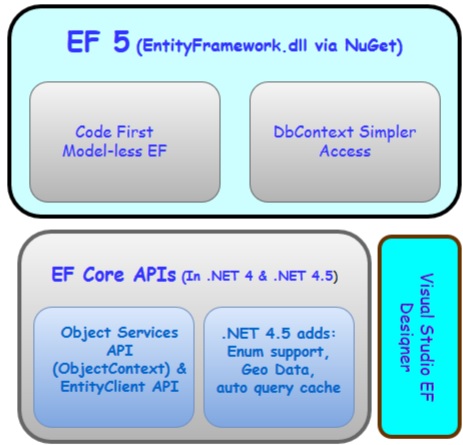
In Entity Framework 6, the core APIs which were previously part of .NET framework are also shipped and distributed as a part of NuGet package.
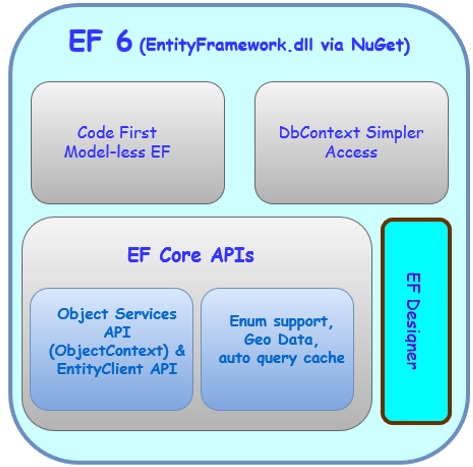
This was necessary to allow Entity Framework to be made open source. However, as a consequence applications will need to be rebuilt whenever there is a need to migrate or upgrade your application from older versions of Entity Framework to EF 6.
The migration process is straightforward if your application uses DbContext, which was shipped in EF 4.1 and later. But if your application is ObjectContext then little more work is required.
Let’s take a look at the following steps you need to go through to upgrade an existing application to EF6.
Step 1 − The first step is to target .NET Framework 4.5.2 and later right click on your project and select properties.
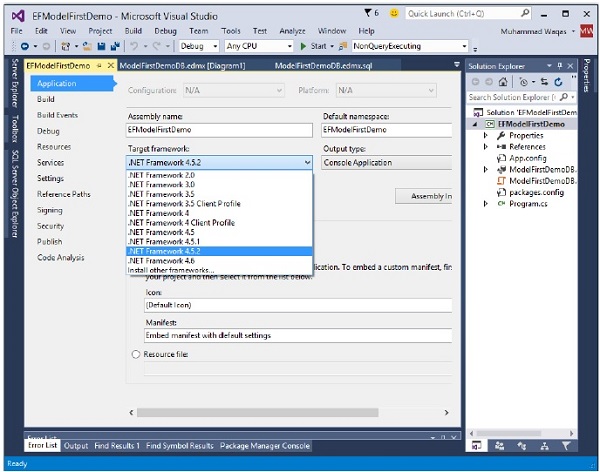
Step 2 − Right click on your project again and select Manage NuGet Packages...
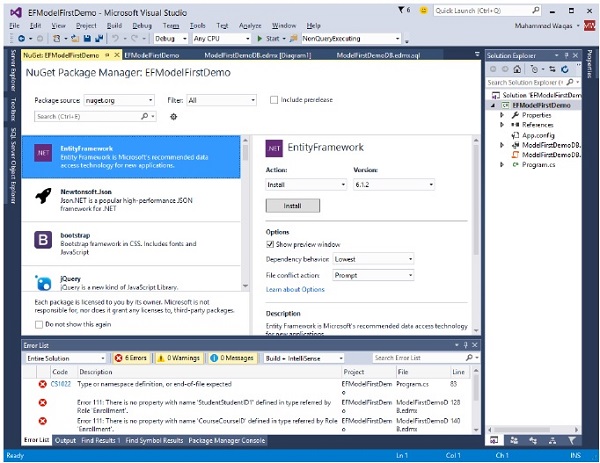
Step 3 − Under the Online tab select EntityFramework and click Install. Make sure that assembly references to System.Data.Entity.dll are removed.
When you install EF6 NuGet package it should automatically remove any references to System.Data.Entity from your project for you.
Step 4 − If you have any model which is created with the EF Designer, then you will also need to update the code generation templates to generate EF6 compatible code.
Step 5 − In your Solution Explorer under your edmx file, delete existing code-generation templates which typically will be named <edmx_file_name>.tt and <edmx_file_name>.Context.tt.
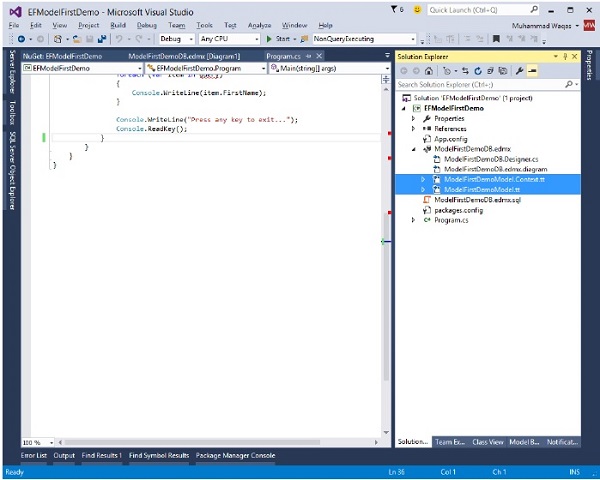
Step 6 − Open your model in the EF Designer, right click on the design surface and select Add Code Generation Item...
Step 7 − Add the appropriate EF 6.x code generation template.
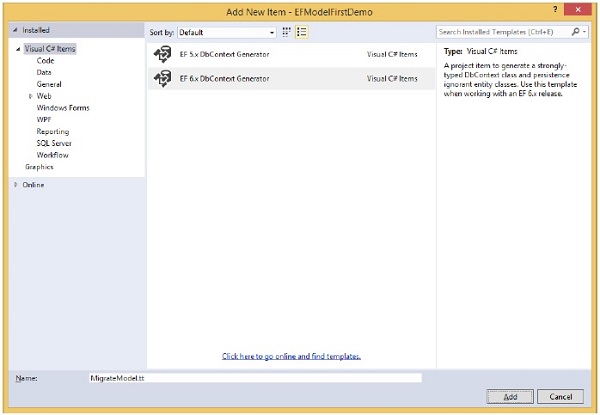
It will also generate EF6 compatible code automatically.
If your applications use EF 4.1 or later you will not need to change anything in the code, because the namespaces for DbContext and Code First types have not changed.
But if your application is using older version of Entity Framework then types like ObjectContext that were previously in System.Data.Entity.dll have been moved to new namespaces.
Step 8 − You will need to update your using or Import directives to build against EF6.
The general rule for namespace changes is that any type in System.Data.* is moved to System.Data.Entity.Core.*. Following are some of them −
- System.Data.EntityException ⇒ System.Data.Entity.Core.EntityException
- System.Data.Objects.ObjectContext ⇒ System.Data.Entity.Core.Objects.ObjectContext;
- System.Data.Objects.DataClasses.RelationshipManager ⇒ System.Data.Entity.Core.Objects.DataClasses.RelationshipManager;
Some types are in the Core namespaces because they are not used directly for most DbContext-based applications.
- System.Data.EntityState ⇒ System.Data.Entity.EntityState
- System.Data.Objects.DataClasses.EdmFunctionAttribute ⇒ System.Data.Entity.DbFunctionAttribute
Your existing Entity Framework project will work in Entity Framework 6.0 without any major changes.
To Continue Learning Please Login