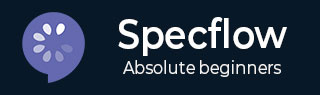
- SpecFlow Tutorial
- SpecFlow - Home
- SpecFlow - Introduction
- Test Driven Development
- Behaviour Driven Development
- SpecFlow - Visual Studio Installation
- Visual Studio Extension Installation
- SpecFlow - Project Set Up
- Other Project Dependencies
- SpecFlow - Runner Activation
- SpecFlow - HTML Reports
- SpecFlow - Binding Test Steps
- SpecFlow - Creating First Test
- Configure Selenium Webdriver
- SpecFlow - Gherkin
- SpecFlow - Gherkin Keywords
- SpecFlow - Feature File
- SpecFlow - Step Definition File
- SpecFlow - Hooks
- SpecFlow - Background Illustration
- Data Driven Testing with Examples
- Data Driven Testing without Examples
- Table conversion to Data Table
- Table conversion to Dictionary
- Table with CreateInstance
- SpecFlow - Table with CreateSet
- SpecFlow Useful Resources
- SpecFlow - Quick Guide
- SpecFlow - Useful Resources
- SpecFlow - Discussion
SpecFlow - Table Conversion to Dictionary
Tables can hold data in a horizontal and vertical direction in the Feature File. With a Dictionary object, we shall see how to access data in the Feature File vertically in a key-value pair.
Step 1: Create a Feature File
The details of how to create a Feature File is discussed in detail in the Chapter − Feature File.
Feature: User credential Scenario: Login module When User types details | KY | Val | | username | tutorialspoint | | password | pwd1 | Then user should be able to login
Step 2: Create C# File to Access Table Data
We must convert a Table to a Dictionary via System.Collections.Generic package. We shall create a new folder within the project and have a C# file in it. Right-click on the SpecFlow Project, then click on Add.
Select the option New Folder.
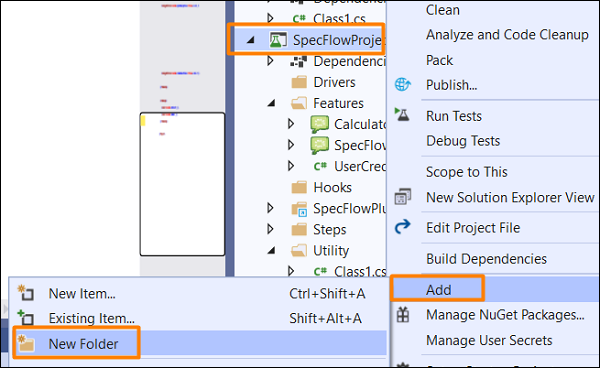
Give the name Utils to the folder.
Right-click on the new Folder created, then select the option Add. Click on Class.
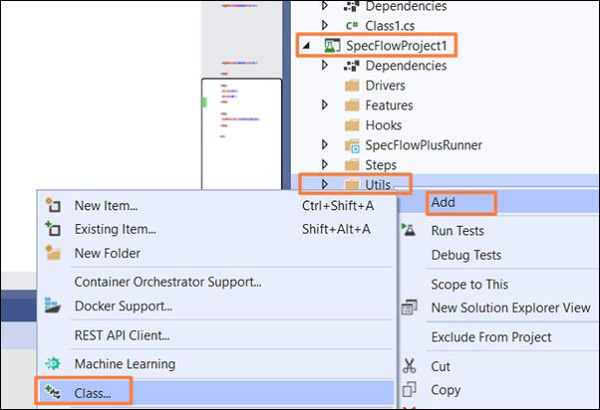
Project Folder Structure
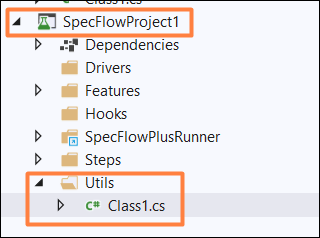
C# Class Implementation
using System; using System.Collections.Generic; using System.Text; using System.Data; using TechTalk.SpecFlow; namespace SpecFlowProject1.Utils { class Class1 { public static Dictionary<string, string> ToDT(Table t) { var dT = new Dictionary<string, string>(); // iterating through rows foreach (var r in t.Rows) { dT.Add(r[0], r[1]); } return dT; } } }
Step 3: Create a Step Definition File
The details of how to create a Step Definition File is discussed in detail in the Chapter − Step Definition File.
using System; using TechTalk.SpecFlow; namespace SpecFlowProject1.Features { [Binding] public class UserCredentialSteps { [When(@"User types details")] public void WhenUserTypesDetails(Table t) { //Accessing C# class method from Step Definition var dict = Utils.Class1.ToDT(t); Console.WriteLine(dict["username"]); Console.WriteLine(dict["password"]); } [Then(@"user should be able to login")] public void ThenUserShouldBeAbleToLogin() { Console.WriteLine("User should be able to login"); } } }
Step 4: Execution & Results
Select User credential(1) Feature, then click on Run All Tests in View.

Select Login Module Scenario, then click on Open additional output for this result link.

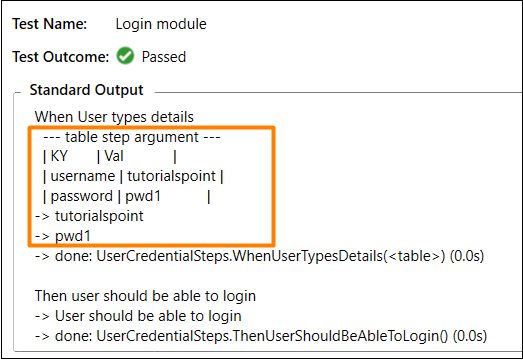
The Scenario got executed with data passed from a Table (converted to a Dictionary) in the Feature File within the When step.