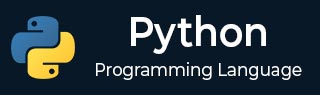
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Sets
A set is one of the built-in data types in Python. In mathematics, set is a collection of distinct objects. Set data type is Python's implementation of a set. Objects in a set can be of any data type.
Set in Python also a collection data type such as list or tuple. However, it is not an ordered collection, i.e., items in a set or not accessible by its positional index. A set object is a collection of one or more immutable objects enclosed within curly brackets {}.
Example 1
Some examples of set objects are given below −
s1 = {"Rohan", "Physics", 21, 69.75} s2 = {1, 2, 3, 4, 5} s3 = {"a", "b", "c", "d"} s4 = {25.50, True, -55, 1+2j} print (s1) print (s2) print (s3) print (s4)
It will produce the following output −
{'Physics', 21, 'Rohan', 69.75} {1, 2, 3, 4, 5} {'a', 'd', 'c', 'b'} {25.5, -55, True, (1+2j)}
The above result shows that the order of objects in the assignment is not necessarily retained in the set object. This is because Python optimizes the structure of set for set operations.
In addition to the literal representation of set (keeping the items inside curly brackets), Python's built-in set() function also constructs set object.
set() Function
set() is one of the built-in functions. It takes any sequence object (list, tuple or string) as argument and returns a set object
Syntax
Obj = set(sequence)
Parameters
sequence − An object of list, tuple or str type
Return value
The set() function returns a set object from the sequence, discarding the repeated elements in it.
Example 2
L1 = ["Rohan", "Physics", 21, 69.75] s1 = set(L1) T1 = (1, 2, 3, 4, 5) s2 = set(T1) string = "TutorialsPoint" s3 = set(string) print (s1) print (s2) print (s3)
It will produce the following output −
{'Rohan', 69.75, 21, 'Physics'} {1, 2, 3, 4, 5} {'u', 'a', 'o', 'n', 'r', 's', 'T', 'P', 'i', 't', 'l'}
Example 3
Set is a collection of distinct objects. Even if you repeat an object in the collection, only one copy is retained in it.
s2 = {1, 2, 3, 4, 5, 3,0, 1, 9} s3 = {"a", "b", "c", "d", "b", "e", "a"} print (s2) print (s3)
It will produce the following output −
{0, 1, 2, 3, 4, 5, 9} {'a', 'b', 'd', 'c', 'e'}
Example 4
Only immutable objects can be used to form a set object. Any number type, string and tuple is allowed, but you cannot put a list or a dictionary in a set.
s1 = {1, 2, [3, 4, 5], 3,0, 1, 9} print (s1) s2 = {"Rohan", {"phy":50}} print (s2)
It will produce the following output −
s1 = {1, 2, [3, 4, 5], 3,0, 1, 9} ^^^^^^^^^^^^^^^^^^^^^^^^^^^^ TypeError: unhashable type: 'list' s2 = {"Rohan", {"phy":50}} ^^^^^^^^^^^^^^^^^^^^^ TypeError: unhashable type: 'dict'
Python raises TypeError with a message unhashable types 'list' or 'dict'. Hashing generates a unique number for an immutable item that enables quick search inside computer's memory. Python has built-in hash() function. This function is not supported by list or dictionary.
Even though mutable objects are not stored in a set, set itself is a mutable object. Python has a special operators to work with sets, and there are different methods in set class to perform add, remove, update operations on elements of a set object.