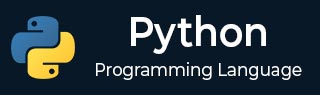
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Exceptions Handling
An exception is defined as an event that occurs during the execution of a program and disrupts the normal flow of program instructions. The reason for event like exception could be attempting to divide by zero, accessing a non-existent file, or trying to convert a string value to an integer where it's not possible.
Python provides two very important features to handle any unexpected error in your Python programs and to add debugging capabilities in them −
Exception Handling − This would be covered in this tutorial. Here is a list of standard Exceptions available in Python: Standard Exceptions.
Assertions − This would be covered in Assertions in Python tutorial.
List of Standard Exceptions −
Sr.No. | Exception Name & Description |
---|---|
1 | Exception Base class for all exceptions |
2 | StopIteration Raised when the next() method of an iterator does not point to any object. |
3 | SystemExit Raised by the sys.exit() function. |
4 | StandardError Base class for all built-in exceptions except StopIteration and SystemExit. |
5 | ArithmeticError Base class for all errors that occur for numeric calculation. |
6 | OverflowError Raised when a calculation exceeds maximum limit for a numeric type. |
7 | FloatingPointError Raised when a floating point calculation fails. |
8 | ZeroDivisionError Raised when division or modulo by zero takes place for all numeric types. |
9 | AssertionError Raised in case of failure of the Assert statement. |
10 | AttributeError Raised in case of failure of attribute reference or assignment. |
11 | EOFError Raised when there is no input from either the raw_input() or input() function and the end of file is reached. |
12 | ImportError Raised when an import statement fails. |
13 | KeyboardInterrupt Raised when the user interrupts program execution, usually by pressing Ctrl+c. |
14 | LookupError Base class for all lookup errors. |
15 | IndexError Raised when an index is not found in a sequence. |
16 | KeyError Raised when the specified key is not found in the dictionary. |
17 | NameError Raised when an identifier is not found in the local or global namespace. |
18 | UnboundLocalError Raised when trying to access a local variable in a function or method but no value has been assigned to it. |
19 | EnvironmentError Base class for all exceptions that occur outside the Python environment. |
20 | IOError Raised when an input/ output operation fails, such as the print statement or the open() function when trying to open a file that does not exist. |
21 | IOError Raised for operating system-related errors. |
22 | SyntaxError Raised when there is an error in Python syntax. |
23 | IndentationError Raised when indentation is not specified properly. |
24 | SystemError Raised when the interpreter finds an internal problem, but when this error is encountered the Python interpreter does not exit. |
25 | SystemExit Raised when Python interpreter is quit by using the sys.exit() function. If not handled in the code, causes the interpreter to exit. |
26 | TypeError Raised when an operation or function is attempted that is invalid for the specified data type. |
27 | ValueError Raised when the built-in function for a data type has the valid type of arguments, but the arguments have invalid values specified. |
28 | RuntimeError Raised when a generated error does not fall into any category. |
29 | NotImplementedError Raised when an abstract method that needs to be implemented in an inherited class is not actually implemented. |
Assertions in Python
An assertion is a sanity-check that you can turn on or turn off when you are done with your testing of the program.
The easiest way to think of an assertion is to liken it to a raise-if statement (or to be more accurate, a raise-if-not statement). An expression is tested, and if the result comes up false, an exception is raised.
Assertions are carried out by the assert statement, the newest keyword to Python, introduced in version 1.5.
Programmers often place assertions at the start of a function to check for valid input, and after a function call to check for valid output.
The assert Statement
When it encounters an assert statement, Python evaluates the accompanying expression, which is hopefully true. If the expression is false, Python raises an AssertionError exception.
The syntax for assert is −
assert Expression[, Arguments]
If the assertion fails, Python uses ArgumentExpression as the argument for the AssertionError. AssertionError exceptions can be caught and handled like any other exception using the try-except statement, but if not handled, they will terminate the program and produce a trace back.
Example
Here is a function that converts a temperature from degrees Kelvin to degrees Fahrenheit. Since zero degrees Kelvin is as cold as it gets, the function bails out if it sees a negative temperature −
def KelvinToFahrenheit(Temperature): assert (Temperature >= 0),"Colder than absolute zero!" return ((Temperature-273)*1.8)+32 print (KelvinToFahrenheit(273)) print (int(KelvinToFahrenheit(505.78))) print (KelvinToFahrenheit(-5))
When the above code is executed, it produces the following result −
32.0 451 Traceback (most recent call last): File "test.py", line 9, in <module> print (KelvinToFahrenheit(-5)) File "test.py", line 4, in KelvinToFahrenheit assert (Temperature >= 0),"Colder than absolute zero!" AssertionError: Colder than absolute zero!
What is Exception?
An exception is an event, which occurs during the execution of a program that disrupts the normal flow of the program's instructions. In general, when a Python script encounters a situation that it cannot cope with, it raises an exception. An exception is a Python object that represents an error.
When a Python script raises an exception, it must either handle the exception immediately otherwise it terminates and quits.
Handling an Exception in Python
If you have some suspicious code that may raise an exception, you can defend your program by placing the suspicious code in a try: block. After the try: block, include an except: statement, followed by a block of code which handles the problem as elegantly as possible.
The try: block contains statements which are susceptible for exception
If exception occurs, the program jumps to the except: block.
If no exception in the try: block, the except: block is skipped.
Syntax
Here is the simple syntax of try...except...else blocks −
try: You do your operations here ...................... except ExceptionI: If there is ExceptionI, then execute this block. except ExceptionII: If there is ExceptionII, then execute this block. ...................... else: If there is no exception then execute this block.
Here are few important points about the above-mentioned syntax −
A single try statement can have multiple except statements. This is useful when the try block contains statements that may throw different types of exceptions.
You can also provide a generic except clause, which handles any exception.
After the except clause(s), you can include an else clause. The code in the else block executes if the code in the try: block does not raise an exception.
The else block is a good place for code that does not need the try: block's protection.
Example
This example opens a file, writes content in the file and comes out gracefully because there is no problem at all.
try: fh = open("testfile", "w") fh.write("This is my test file for exception handling!!") except IOError: print ("Error: can\'t find file or read data") else: print ("Written content in the file successfully") fh.close()
It will produce the following output −
Written content in the file successfully
However, change the mode parameter in open() function to "w". If the testfile is not already present, the program encounters IOError in except block, and prints following error message −
Error: can't find file or read data
Example
This example tries to open a file where you do not have write permission, so it raises an exception −
try: fh = open("testfile", "r") fh.write("This is my test file for exception handling!!") except IOError: print ("Error: can\'t find file or read data") else: print ("Written content in the file successfully")
This produces the following result −
Error: can't find file or read data
The except Clause with No Exceptions
You can also use the except statement with no exceptions defined as follows −
try: You do your operations here; ...................... except: If there is any exception, then execute this block. ...................... else: If there is no exception then execute this block.
This kind of a try-except statement catches all the exceptions that occur. Using this kind of try-except statement is not considered a good programming practice though, because it catches all exceptions but does not make the programmer identify the root cause of the problem that may occur.
The except Clause with Multiple Exceptions
You can also use the same except statement to handle multiple exceptions as follows −
try: You do your operations here; ...................... except(Exception1[, Exception2[,...ExceptionN]]]): If there is any exception from the given exception list, then execute this block. ...................... else: If there is no exception then execute this block.
The try-finally Clause
You can use a finally: block along with a try: block. The finally block is a place to put any code that must execute, whether the try-block raised an exception or not. The syntax of the try-finally statement is this −
try: You do your operations here; ...................... Due to any exception, this may be skipped. finally: This would always be executed. ......................
You cannot use else clause as well along with a finally clause.
Example
try: fh = open("testfile", "w") fh.write("This is my test file for exception handling!!") finally: print ("Error: can\'t find file or read data")
If you do not have permission to open the file in writing mode, then this will produce the following result −
Error: can't find file or read data
Same example can be written more cleanly as follows −
try: fh = open("testfile", "w") try: fh.write("This is my test file for exception handling!!") finally: print ("Going to close the file") fh.close() except IOError: print ("Error: can\'t find file or read data")
When an exception is thrown in the try block, the execution immediately passes to the finally block. After all the statements in the finally block are executed, the exception is raised again and is handled in the except statements if present in the next higher layer of the try-except statement.
Argument of an Exception
An exception can have an argument, which is a value that gives additional information about the problem. The contents of the argument vary by exception. You capture an exception's argument by supplying a variable in the except clause as follows −
try: You do your operations here; ...................... except ExceptionType, Argument: You can print value of Argument here...
If you write the code to handle a single exception, you can have a variable follow the name of the exception in the except statement. If you are trapping multiple exceptions, you can have a variable follow the tuple of the exception.
This variable receives the value of the exception mostly containing the cause of the exception. The variable can receive a single value or multiple values in the form of a tuple. This tuple usually contains the error string, the error number, and an error location.
Example
Following is an example for a single exception −
# Define a function here. def temp_convert(var): try: return int(var) except ValueError as Argument: print ("The argument does not contain numbers\n", Argument) # Call above function here. temp_convert("xyz")
This produces the following result −
The argument does not contain numbers invalid literal for int() with base 10: 'xyz'
Raising an Exceptions
You can raise exceptions in several ways by using the raise statement. The general syntax for the raise statement is as follows.
Syntax
raise [Exception [, args [, traceback]]]
Here, Exception is the type of exception (for example, NameError) and argument is a value for the exception argument. The argument is optional; if not supplied, the exception argument is None.
The final argument, trace back, is also optional (and rarely used in practice), and if present, is the traceback object used for the exception.
Example
An exception can be a string, a class or an object. Most of the exceptions that the Python core raises are classes, with an argument that is an instance of the class. Defining new exceptions is quite easy and can be done as follows −
def functionName( level ): if level < 1: raise "Invalid level!", level # The code below to this would not be executed # if we raise the exception
Note: In order to catch an exception, an "except" clause must refer to the same exception thrown either class object or simple string. For example, to capture above exception, we must write the except clause as follows −
try: Business Logic here... except "Invalid level!": Exception handling here... else: Rest of the code here...
User-Defined Exceptions
Python also allows you to create your own exceptions by deriving classes from the standard built-in exceptions.
Here is an example related to RuntimeError. Here, a class is created that is subclassed from RuntimeError. This is useful when you need to display more specific information when an exception is caught.
In the try block, the user-defined exception is raised and caught in the except block. The variable e is used to create an instance of the class Networkerror.
class Networkerror(RuntimeError): def __init__(self, arg): self.args = arg
So once you defined above class, you can raise the exception as follows −
try: raise Networkerror("Bad hostname") except Networkerror,e: print (e.args)