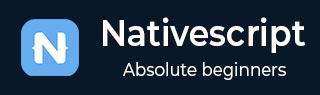
- NativeScript Tutorial
- NativeScript - Home
- NativeScript - Introduction
- NativeScript - Installation
- NativeScript - Architecture
- NativeScript - Angular Application
- NativeScript - Templates
- NativeScript - Widgets
- NativeScript - Layout Containers
- NativeScript - Navigation
- NativeScript - Events Handling
- NativeScript - Data Binding
- NativeScript - Modules
- NativeScript - Plugins
- NativeScript - Native APIs Using JavaScript
- NativeScript - Creating an Application in Android
- NativeScript - Creating an Application in iOS
- NativeScript - Testing
- NativeScript Useful Resources
- NativeScript - Quick Guide
- NativeScript - Useful Resources
- NativeScript - Discussion
NativeScript - Native APIs Using JavaScript
This section explains about the overview of accessing Native APIs using JavaScript.
Marshalling
The NativeScript Runtime provides implicit type conversion for both android and iOS platforms. This concept is known as marshalling. For example, NativeScript- iOS paltform can implicitly convert JavaScript and Objective-C data types similarly, Java/Kotlin can easily be mapped to JavaScript project types and values. Let us understand how to perform marshalling in each type one by one briefly.
Numeric Values
We can easily convert iOS and android numeric data types into JavaScript numbers. Simple numeric conversion for iOS into JavaScript is defined below −
console.log(`max(7,9) = ${max(7,9)}`);
Here,
The native max() function is converted into JavaScript number.
Android Environment
Java supports different numeric types such as byte, short, int, float, double and long. JavaScript has only number type.
Consider a simple Java class shown below −
class Demo extends java.lang.Object { public int maxMethod(int a,int b) { if(a>b) { return a; } else { return b; } } }
Here,
The above code contains two integer arguments. We can call the above code object using JavaScript as shown below −
//Create an instance for Demo class var obj = new Demo(); //implicit integer conversion for calling the above method obj.maxMethod(7,9);
Strings
Android strings are defined in java.lang.string and iOS strings are defined in NSSring. Let us see how to perform marshalling in both platforms.
Android
Strings are immutable but String buffers support mutable strings.
Below code is an example for simple mapping −
//Create android label widget var label = new android.widget.Label(); //Create JavaScript string var str = "Label1"; //Convert JavaScript string into java label.setText(str); // text is converted to java.lang.String
Boolean class is defined in java.lang.Boolean. This class wraps a value of boolean in an object. We can easily convert boolean to String and vice-versa. Simple example is defined as given below −
//create java string let data = new java.lang.String('NativeScript'); //map java String to JavaScript string, let result = data.startsWith('N'); //return result console.log(result);// true
iOS environment
NSString class is immutable but its subclass NSMutableString is immutable. This class contains a collection of methods for working with strings. It is declared as below −
class NSString : NSObject
Consider a simple objective-c declaration as shown below −
NSString *str = @"nativescript"; //convert the string to uppercase NSString *str1; str1 = [str uppercaseString]; NSLog(@"Uppercase String : %@\n", str1 );
NSStrings can easily be mapped to JavaScript strings.
Array
This section explains about how to perform marshalling in arrays. Let’s take an example of iOS environment first.
Array Declaration
class NSArray : NSObject
Here,
NSArray is used to manage ordered collection of objects called arrays. It is used to create static array. Its sub class NSMutableArray is used to create dynamic arrays.
Consider NSArray objects can be created using array literals as shown below −
let array: NSArray = ["React","Vue","TypeScript"]
Now, we can map this array into JavaScript as shown below −
//create native array let nsArr = NSArray.arrayWithArray("React","Vue","TypeScript"]); //create simple javascript array let jsArr = ["Hello,World","NativeScript"]; //Now compare the two arrays, let compare = nsArr.isEqual(jsArr); console.log(comapre);
This will return the output as false.
Android array declaration
Java arrays are defined in java.util.Arrays. This class contains various methods for manipulating arrays. An example is shown below −
//javascript array let data = [12,45,23,56,34,78,50]; //create java array let result = ns.example.Math.maxElement(data); console.log(result);
Classes and Objects
Classes and Objects are basic concepts of Object Oriented Programming. Class is a user defined prototype. Object is an instance of class. Class represents the set of properties or methods that are common to all objects of one type. Let us understand native classes and objects for both mobile development environments.
Android Environment
Java and Kotlin classes have unique identifiers denoted by the full package name.
For example,
android.view.View − It is a basic user interface class for screen layout and interaction with the user. We can access this class in JavaScript as shown below −
const View = android.view.View;
First, we import the class using the below statement −
import android.view.View;
Next create a class as given below −
public class MyClass { public static void staticMethod(context) { //create view instance android.view.View myview = new android.view.View(context); } }
In the above same class, we can access JavaScript function using the below code −
const myview = new android.view.View(context);
Similarly, we can access interfaces, constants and enumerations within java.lang packages.
iOS Environment
Objective-C classes are defined in two sections @interface and @implementation. Class definition starts with the keyword @interface followed by the interface(class) name. In Objective-C, all classes are derived from the base class called NSObject.
It is the superclass of all Objective-C classes. Simple Circle class is defined as shown below −
@interface Circle:NSObject { //Instance variable int radius; } @end
Consider a class with one method as shown below −
@interface MyClass : NSObject + (void)baseStaticMethod; @end
This class can be converted to javascript using the below code −
function MyClass() { /* native call */ }; Object.setPrototypeOf(MyClass, NSObject); BaseClass.baseStaticMethod = function () { /* native call */ };
JavaScript instanceof operator is used to verify, if an object inherits from a given class. This can be defined as −
var obj = MyClass.alloc().init(); // object creation console.log(obj instanceof NSObject); //return true
Here,
Objective-C instances are created using alloc, init or new methods. In the above example, we can easily create object initialization using new method as below −
var obj = MyClass.new();
Similarly, you can access static methods and properties.