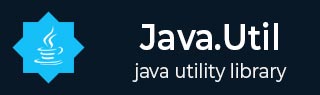
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java UUID version() Method
Description
The Java UUID version() method is used to return the version number associated with this UUID. The version number describes how this UUID was generated.
Declaration
Following is the declaration for java.util.UUID.version() method.
public int version()
Parameters
NA
Return Value
The method call returns the version number of this UUID.
Exception
NA
Getting Version of a UUID generated using Standard Formatted String Example
The following example shows the usage of Java UUID version() method to get the version number of this UUID. We've created a UUID object using a given string. Then we've printed the version number of this UUID object using version() method.
package com.tutorialspoint; import java.util.UUID; public class UUIDDemo { public static void main(String[] args) { // creating UUID UUID x = UUID.fromString("38400000-8cf0-11bd-b23e-10b96e4ef00d"); // getting version number System.out.println("version number: "+x.version()); } }
Output
Let us compile and run the above program, this will produce the following result.
version number: 1
Getting Version of a Random UUID generated Example
The following example shows usage of Java UUID version() method to get the version number of this UUID. We've created a UUID object using randomUUID() method. Then we've printed the version number of this UUID object using version() method.
package com.tutorialspoint; import java.util.UUID; public class UUIDDemo { public static void main(String[] args) { // creating UUID UUID x = UUID.randomUUID(); // getting version number System.out.println("version number: "+x.version()); } }
Output
Let us compile and run the above program, this will produce the following result.
version number: 4
Getting Version of a UUID generated using Bytes Example
The following example shows usage of Java UUID version() method to get the version number of this UUID. We've created a UUID object using nameUUIDFromBytes() method. Then we've printed the version number of this UUID object using version() method.
package com.tutorialspoint; import java.util.UUID; public class UUIDDemo { public static void main(String[] args) { // creating byte array byte[] nbyte = {10,20,30}; // creating UUID from byte UUID uid = UUID.nameUUIDFromBytes(nbyte); // getting version number System.out.println("version number: "+uid.version()); } }
Output
Let us compile and run the above program, this will produce the following result.
version number: 3
To Continue Learning Please Login