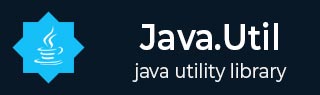
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java TreeSet spliterator() Method
Description
The Java TreeSet spliterator() method is used to get a late-binding and fail-fast Spliterator over the elements in this set.
Declaration
Following is the declaration for java.util.TreeSet.spliterator() method.
public Splspliterator<E> splspliterator()
Parameters
NA
Return Value
The method call returns an Spliterator over the elements in this set.
Exception
NA
Getting Spliterator of a TreeSet of Integer Example
The following example shows the usage of Java TreeSet spliterator() method to get a spliterator of elements of the treeset. We've created a TreeSet object of Integer. Few entries are added using add() method and spliterator is retrieved using spliterator() method and then spliterator is iterated to print the elements.
package com.tutorialspoint; import java.util.Spliterator; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet<Integer> treeset = new TreeSet<>(); // adding in the tree set treeset.add(1); treeset.add(13); treeset.add(17); treeset.add(2); // create ascending spliterator Spliterator<Integer> spliterator = treeset.spliterator(); // displaying the Tree set data System.out.println("Tree set data: "); spliterator.forEachRemaining( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
Tree set data: 1 2 13 17
Getting Spliterator of a TreeSet of String Example
The following example shows the usage of Java TreeSet spliterator() method to get a spliterator of elements of the treeset. We've created a TreeSet object of String. Few entries are added using add() method and spliterator is retrieved using spliterator() method and then spliterator is iterated to print the elements.
package com.tutorialspoint; import java.util.Spliterator; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet<String> treeset = new TreeSet<>(); // adding in the tree set treeset.add("D"); treeset.add("B"); treeset.add("C"); treeset.add("A"); // create ascending spliterator Spliterator<String> spliterator = treeset.spliterator(); // displaying the Tree set data System.out.println("Tree set data: "); spliterator.forEachRemaining( i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result.
Tree set data in ascending order: A B C D
Getting Spliterator of a TreeSet of Object Example
The following example shows the usage of Java TreeSet spliterator() method to get a spliterator of elements of the treeset. We've created a TreeSet object of Student. Few entries are added using add() method and spliterator is retrieved using spliterator() method and then spliterator is iterated to print the elements.
package com.tutorialspoint; import java.util.Spliterator; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet<Student>treeset = new TreeSet<>(); // adding in the tree set treeset.add(new Student(2, "Julie")); treeset.add(new Student(1, "Robert")); treeset.add(new Student(3, "Adam")); treeset.add(new Student(4, "Julia")); // create ascending spliterator Spliterator<Student> spliterator = treeset.spliterator(); // displaying the Tree set data System.out.println("Tree set data: "); spliterator.forEachRemaining( i -> System.out.println(i)); } } class Student implements Comparable<Student> { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int compareTo(Student student) { return this.rollNo - student.rollNo; } }
Output
Let us compile and run the above program, this will produce the following result.
Tree set data: [ 1, Robert ] [ 2, Julie ] [ 3, Adam ] [ 4, Julia ]
To Continue Learning Please Login