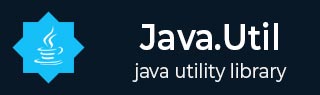
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Scanner findAll() Method
Description
The Java Scanner findAll(Pattern pattern) method returns a stream of match results from this scanner. The stream contains the same results in the same order that would be returned by calling findWithinHorizon(pattern, 0) and then match() successively as long as findWithinHorizon() finds matches. The resulting stream is sequential and ordered. All stream elements are non-null.
Declaration
Following is the declaration for java.util.Scanner.findAll() method
public Stream<MatchResult> findAll(Pattern pattern)
Parameters
pattern − the pattern to be matched
Return Value
This method returns a sequential stream of match results.
Exception
NullPointerException − if pattern is null
IllegalStateException − if this scanner is closed
Java Scanner findInLine(String pattern) Method
Description
The java Scanner findAll(String pattern) method returns a stream of match results that match the provided pattern string.
Declaration
Following is the declaration for java.util.Scanner.findAll(String pattern) method
public Stream<MatchResult> findAll(String pattern)
Parameters
pattern − a string specifying the pattern to search for
Return Value
This method returns the text that matched the specified pattern.
Exception
NullPointerException − if patString is null
IllegalStateException − if this scanner is closed
PatternSyntaxException − if the regular expression's syntax is invalid
Finding multiple patterns Using Scanner on a String Example
The following example shows the usage of Java Scanner findAll(Pattern pattern) method to get a stream of matched result of pattern in a given string. We've created a scanner object using a given string. We've retrieved a stream using findAll(Pattern) method. A stream is then iterated to print the result. Then we printed the string using scanner.nextLine() method.
package com.tutorialspoint; import java.util.Scanner; import java.util.regex.MatchResult; import java.util.regex.Pattern; import java.util.stream.Stream; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); Stream<MatchResult> stream = scanner.findAll(Pattern.compile(".ello")); stream.forEach(i -> System.out.println(i.group())); // print the next line of the string System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
Hello World! 3 + 3.0 = 6
Finding multiple String patterns Using Scanner on a String Example
The following example shows the usage of Java Scanner findAll(String pattern) method to get a stream of matched result of pattern in a given string. We've created a scanner object using a given string. We've retrieved a stream using findAll(String) method. A stream is then iterated to print the result. Then we printed the string using scanner.nextLine() method.
package com.tutorialspoint; import java.util.Scanner; import java.util.regex.MatchResult; import java.util.stream.Stream; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); Stream<MatchResult> stream = scanner.findAll("World"); stream.forEach(i -> System.out.println(i.group())); // print the next line of the string System.out.println(scanner.nextLine()); // close the scanner scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
World ! 3 + 3.0 = 6
Finding multiple patterns Using Scanner on User Input Example
The following example shows the usage of Java Scanner findAll(String pattern) method to get a stream of matched result of pattern in a given string. We've created a scanner object using System.in class. We've retrieved a stream using findAll(String) method. A stream is then iterated to print the result. Then we printed the string using scanner.nextLine() method.
package com.tutorialspoint; import java.util.Scanner; import java.util.regex.MatchResult; import java.util.stream.Stream; public class ScannerDemo { public static void main(String[] args) { // create a new scanner with the System input Scanner scanner = new Scanner(System.in); Stream<MatchResult> stream = scanner.findAll("World"); stream.forEach(i -> System.out.println(i.group())); // close the scanner scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result − (where we've entered Hello World and pressed enter key.)
Hello World World
To Continue Learning Please Login