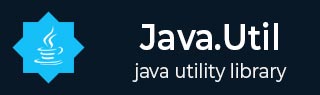
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java EnumSet of() Method
Description
The Java EnumSet of(E e) method creates an enum set initially containing the specified element.
Declaration
Following is the declaration for java.util.EnumSet.of() method
public static <E extends Enum<E>> EnumSet<E> of(E e)
Parameters
e − the element that this set is to contain initially.
Return Value
This method returns an enum set initially containing the specified element.
Exception
NullPointerException − if e is null
Java EnumSet of(E first, E... rest) Method
Description
The java.util.EnumSet.of(E first, E... rest) method creates an enum set initially containing the specified elements. This factory, whose parameter list uses the varargs feature, may be used to create an enum set initially containing an arbitrary number of elements, but it is likely to run slower than the overloadings that do not use varargs.
Declaration
Following is the declaration for java.util.EnumSet.of(E first, E... rest) method
public static <E extends Enum<E>> EnumSet<E> of(E first, E... rest)
Parameters
first − an element that the set is to contain initially.
rest − the remaining elements the set is to contain initially.
Return Value
This method returns an enum set initially containing the specified elements.
Exception
NullPointerException − if e is null
Java EnumSet of(E e1, E e2) Method
Description
The java.util.EnumSet.of(E e1,E e2) method creates an enum set initially containing the two specified elements.
Declaration
Following is the declaration for java.util.EnumSet.of(E e1,E e2) method
public static <E extends Enum<E>> EnumSet<E> of(E e1,E e2)
Parameters
e1 − the element that this set is to contain initially.
e2 − another element that this set is to contain initially.
Return Value
This method returns an enum set initially containing the specified elements.
Exception
NullPointerException − if any of parameters is null
Java EnumSet of(E e1, E e2, E e3) Method
Description
The java.util.EnumSet.of(E e1,E e2,E e3) method creates an enum set initially containing the three specified elements.
Declaration
Following is the declaration for java.util.EnumSet.of(E e1,E e2,E e3) method
public static <E extends Enum<E>> EnumSet<E> of(E e1,E e2,E e3)
Parameters
e1 − the element that this set is to contain initially.
e2 − another element that this set is to contain initially.
e3 − another element that this set is to contain initially.
Return Value
This method returns an enum set initially containing the specified elements.
Exception
NullPointerException − if any of parameters is null
Java EnumSet of(E e1, E e2, E e3, E e4) Method
Description
The java.util.EnumSet.of(E e1,E e2,E e3,E e4) method creates an enum set initially containing the four specified elements.
Declaration
Following is the declaration for java.util.EnumSet.of(E e1,E e2,E e3,E e4) method
public static <E extends Enum<E>> EnumSet<E> of(E e1,E e2,E e3,E e4)
Parameters
e1 − the element that this set is to contain initially.
e2 − another element that this set is to contain initially.
e3 − another element that this set is to contain initially.
e4 − another element that this set is to contain initially.
Return Value
This method returns an enum set initially containing the specified elements.
Exception
NullPointerException − if any of parameters is null
Java EnumSet of(E e1, E e2, E e3, E e4, E e5) Method
Description
The java.util.EnumSet.of(E e1,E e2,E e3,E e4,E e5) method creates an enum set initially containing the five specified elements.
Declaration
Following is the declaration for java.util.EnumSet.of(E e1,E e2,E e3,E e4,E e5) method
public static <E extends Enum<E>> EnumSet<E> of(E e1,E e2,E e3,E e4,E e5)
Parameters
e1 − the element that this set is to contain initially.
e2 − another element that this set is to contain initially.
e3 − another element that this set is to contain initially.
e4 − another element that this set is to contain initially.
e5 − another element that this set is to contain initially.
Return Value
This method returns an enum set initially containing the specified elements.
Exception
NullPointerException − if any of parameters is null
Creating EnumSet using One Enum Value Example
The following example shows the usage of Java EnumSet of(E) method to populate the EnumSet instance. We've created a enum Numbers. Then a EnumSet instance is created using a enum value and resulted enumSet is printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create a set that contains an enum EnumSet<Numbers> set = EnumSet.of(Numbers.ONE); // print set System.out.println("Set:" + set); } }
Output
Let us compile and run the above program, this will produce the following result −
Set:[ONE]
Creating EnumSet using Multiple Enum Values Example
The following example shows the usage of Java EnumSet of(E, E...) method to populate the EnumSet instance. We've created a enum Numbers. Then a EnumSet instance is created using a enum value and an array of enums and resulted enumSet is printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create an array Numbers[] numbers = {Numbers.TWO, Numbers.THREE}; // create a set that contains all of enum and array of enums EnumSet<Numbers> set = EnumSet.of(Numbers.ONE, numbers); // print set System.out.println("Set:" + set); } }
Output
Let us compile and run the above program, this will produce the following result −
Set:[ONE, TWO, THREE]
Creating EnumSet using Two Enum Values Example
The following example shows the usage of Java EnumSet of(E, E) method to populate the EnumSet instance using two enum values. We've created a enum Numbers. Then a EnumSet instance is created using two enums and resulted enumSet is printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create a set that contains both enums EnumSet<Numbers> set = EnumSet.of(Numbers.ONE, Numbers.TWO); // print set System.out.println("Set:" + set); } }
Output
Let us compile and run the above program, this will produce the following result −
Set:[ONE, TWO]
Creating EnumSet using Three Enum Values Example
The following example shows the usage of Java EnumSet of(E, E, E) method to populate the EnumSet instance using three enum values. We've created a enum Numbers. Then a EnumSet instance is created using three enums and resulted enumSet is printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create a set that contains three enums EnumSet<Numbers> set = EnumSet.of(Numbers.ONE, Numbers.TWO, Numbers.THREE ); // print set System.out.println("Set:" + set); } }
Output
Let us compile and run the above program, this will produce the following result −
Set:[ONE, TWO, THREE]
Creating EnumSet using Four Enum Values Example
The following example shows the usage of Java EnumSet of(E, E, E, E) method to populate the EnumSet instance using four enum values. We've created a enum Numbers. Then a EnumSet instance is created using four enums and resulted enumSet is printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create a set that contains four enums EnumSet<Numbers> set = EnumSet.of(Numbers.ONE, Numbers.TWO, Numbers.THREE, Numbers.FOUR ); // print set System.out.println("Set:" + set); } }
Output
Let us compile and run the above program, this will produce the following result −
Set:[ONE, TWO, THREE, FOUR]
Creating EnumSet using Five Enum Values Example
The following example shows the usage of Java EnumSet of(E, E, E, E, E) method to populate the EnumSet instance using five enum values. We've created a enum Numbers. Then a EnumSet instance is created using five enums and resulted enumSet is printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create a set that contains five enums EnumSet<Numbers> set = EnumSet.of(Numbers.ONE, Numbers.TWO, Numbers.THREE, Numbers.FOUR, Numbers.FIVE); // print set System.out.println("Set:" + set); } }
Output
Let us compile and run the above program, this will produce the following result −
Set:[ONE, TWO, THREE, FOUR, FIVE]
To Continue Learning Please Login