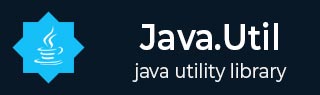
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Date from() Method
Description
The Java Date from(Instant instant) returns a date instance from an Instant object. Instant uses a higher precision of nanoseconds, whereas Date uses a precision of milliseconds. During conversion any excess precision information is truncated. Instant can store points on the time-line further in the future and further in the past than Date and such scenario will throw an exception.
Declaration
Following is the declaration for java.util.Date.from(Instant instant) method
public static Date from​(Instant instant)
Parameters
instant − the instant to convert.
Return Value
This method returns a Date representing the same point on the time-line as the provided instant.
Exception
NullPointerException − if instant is null.
IllegalArgumentException − if the instant is too large to represent as a Date.
Creating Date Instance from Instant.now() Method Example
The following example shows the usage of Java Date from() method. We're creating a Date instance of current time using Instant using from() method.
package com.tutorialspoint; import java.time.Instant; import java.util.Date; public class DateDemo { public static void main(String[] args) { // create a date Date date = Date.from(Instant.now()); System.out.println("Date: " + date.toString()); } }
Output
Let us compile and run the above program, this will produce the following result −
Date: Mon Apr 01 12:10:07 IST 2024
Creating Date Instance from Instant.MAX property Example
The following example shows the usage of Java Date from() method. We're creating a Date instance of current time using Instant.MAX using from() method. Exception will occur as Instant is too large to convert into date.
package com.tutorialspoint; import java.time.Instant; import java.util.Date; public class DateDemo { public static void main(String[] args) { // create a date Date date = Date.from(Instant.MAX); System.out.println("Date: " + date.toString()); } }
Output
Let us compile and run the above program, this will produce the following result −
Exception in thread "main" java.lang.IllegalArgumentException: java.lang.ArithmeticException: long overflow at java.base/java.util.Date.from(Date.java:1362) at com.tutorialspoint.DateDemo.main(DateDemo.java:10) Caused by: java.lang.ArithmeticException: long overflow at java.base/java.lang.Math.multiplyExact(Math.java:946) at java.base/java.lang.Math.multiplyExact(Math.java:922) at java.base/java.time.Instant.toEpochMilli(Instant.java:1236) at java.base/java.util.Date.from(Date.java:1360) ... 1 more
Comparing Date Instance with Instant.now() time Example
The following example shows the usage of Java Date from() method. We're creating a Date instance of current time using Instant using from() method. Here date object truncates the nanoseconds.
package com.tutorialspoint; import java.time.Instant; import java.util.Date; public class DateDemo { public static void main(String[] args) { // create a date Date date = Date.from(Instant.now()); System.out.println(Instant.now()); System.out.println("Date: " + date.toString()); } }
Output
Let us compile and run the above program, this will produce the following result −
2024-04-01T06:39:42.885311400Z Date: Mon Apr 01 12:09:42 IST 2024
To Continue Learning Please Login