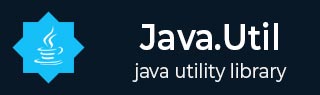
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections reverseOrder() Method
Description
The Java Collections reverseOrder() method is used to get a comparator that imposes the reverse of the natural ordering on a collection of objects that implement the Comparable interface.
Declaration
Following is the declaration for java.util.Collections.reverseOrder() method.
public static <T> Comparator<T> reverseOrder()
Parameters
NA
Return Value
The method call returns a comparator that imposes the reverse of the natural ordering on a collection of objects that implement the Comparable interface.
Exception
NA
Java Collections reverseOrder(Comparator<T>) Method
Description
The reverseOrder(Comparator<T>) method is used to get a comparator that imposes the reverse ordering of the specified comparator.
Declaration
Following is the declaration for java.util.Collections.reverseOrder() method.
public static <T> Comparator<T> reverseOrder(Comparator<T> cmp)
Parameters
cmp − This is the comparator.
Return Value
The method call returns a comparator that imposes the reverse ordering of the specified comparator.
Exception
NA
Getting Reverse Order Comparator for a List of Integers Example
The following example shows the usage of Java Collection reverseOrder() method. We've created a List object with some integers, printed the original list. Using reverseOrder() method, we've retrieved a comparator and then reversed sorted the list and printed it.
package com.tutorialspoint; import java.util.Arrays; import java.util.Collections; import java.util.Comparator; import java.util.LinkedList; import java.util.List; public class CollectionsDemo { public static void main(String args[]) { // create a list object List<Integer> list = new LinkedList<>(Arrays.asList(-28,20,-12,8)); System.out.println(list); // create comparator for reverse order Comparator<Integer> cmp = Collections.reverseOrder(); // sort the list Collections.sort(list, cmp); System.out.println("List sorted in ReverseOrder: "); System.out.println(list); } }
Output
Let us compile and run the above program, this will produce the following result.
[-28, 20, -12, 8] List sorted in ReverseOrder: [20, 8, -12, -28]
Getting Reverse Order Comparator for a List of Strings Example
The following example shows the usage of Java Collection reverseOrder() method. We've created a List object with some strings, printed the original list. Using reverseOrder() method, we've retrieved a comparator and then reversed sorted the list and printed it.
package com.tutorialspoint; import java.util.Arrays; import java.util.Collections; import java.util.Comparator; import java.util.LinkedList; import java.util.List; public class CollectionsDemo { public static void main(String args[]) { // create a list object List<String> list = new LinkedList<>(Arrays.asList("A","B","C","D")); System.out.println(list); // create comparator for reverse order Comparator<String> cmp = Collections.reverseOrder(); // sort the list Collections.sort(list, cmp); System.out.println("List sorted in ReverseOrder: "); System.out.println(list); } }
Output
Let us compile and run the above program, this will produce the following result.
[A, B, C, D] List sorted in ReverseOrder: [D, C, B, A]
Getting Reverse Order Comparator for a List of Objects Example
The following example shows the usage of Java Collection reverseOrder(Comparator) method. We've created a List object with some Student objects, printed the original list. Using reverseOrder(Comparator) method, we've retrieved a comparator and then reversed sorted the list and printed it.
package com.tutorialspoint; import java.util.Arrays; import java.util.Collections; import java.util.Comparator; import java.util.LinkedList; import java.util.List; public class CollectionsDemo { public static void main(String args[]) { // create a list object List<Student> list = new LinkedList<>(Arrays.asList(new Student(1, "Julie"), new Student(3, "Adam"), new Student(2, "Robert"))); System.out.println(list); RollNoComparator comparator = new RollNoComparator(); // create comparator for reverse order Comparator<Student> cmp = Collections.reverseOrder(comparator); // sort the list Collections.sort(list, cmp); System.out.println("List sorted in ReverseOrder: "); System.out.println(list); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } public int getRollNo() { return rollNo; } public void setRollNo(int rollNo) { this.rollNo = rollNo; } public String getName() { return name; } public void setName(String name) { this.name = name; } } class RollNoComparator implements Comparator<Student>{ @Override public int compare(Student o1, Student o2) { return o1.getRollNo()-o2.getRollNo(); } }
Output
Let us compile and run the above program, this will produce the following result.
[[ 1, Julie ], [ 3, Adam ], [ 2, Robert ]] List sorted in ReverseOrder: [[ 3, Adam ], [ 2, Robert ], [ 1, Julie ]]
To Continue Learning Please Login