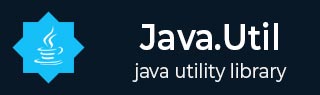
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections emptyEnumeration() Method
Description
The Java Collections emptyEnumeration() method is used to get the empty enumeration. Enumeration is empty and its hasMoreElements method always returns false.
Declaration
Following is the declaration for Java Collections emptyEnumeration() method.
public static final <T> Enumeration<T> emptyEnumeration()
Parameters
NA
Return Value
NA
Exception
NA
Getting Empty Enumeration of Integers Example
The following example shows the usage of Java Collection emptyEnumeration() method to get an empty enumeration of Integers. We've created an empty enumeration using emptyEnumeration() method and then checked if enumeration has elements or not.
package com.tutorialspoint; import java.util.Collections; import java.util.Enumeration; public class CollectionsDemo { public static void main(String args[]) { // create an empty list Enumeration<Integer> emptyEnumeration = Collections.emptyEnumeration(); System.out.println("Created empty enumeration, it has elements: "+emptyEnumeration.hasMoreElements()); } }
Output
Let us compile and run the above program, this will produce the following result.
Created empty enumeration, it has elements: false
Getting Empty Enumeration of Strings Example
The following example shows the usage of Java Collection emptyEnumeration() method to get an empty enumeration of Strings. We've created an empty enumeration using emptyEnumeration() method and then checked if enumeration has elements or not.
package com.tutorialspoint; import java.util.Collections; import java.util.Enumeration; public class CollectionsDemo { public static void main(String args[]) { // create an empty list Enumeration<String> emptyEnumeration = Collections.emptyEnumeration(); System.out.println("Created empty enumeration, it has elements: "+emptyEnumeration.hasMoreElements()); } }
Output
Let us compile and run the above program, this will produce the following result.
Created empty enumeration, it has elements: false
Getting Empty Enumeration of Objects Example
The following example shows the usage of Java Collection emptyEnumeration() method to get an empty enumeration of Student objects. We've created an empty enumeration using emptyEnumeration() method and then checked if enumeration has elements or not.
package com.tutorialspoint; import java.util.Collections; import java.util.Enumeration; public class CollectionsDemo { public static void main(String args[]) { // create an empty list Enumeration<Student> emptyEnumeration = Collections.emptyEnumeration(); System.out.println("Created empty enumeration, it has elements: "+emptyEnumeration.hasMoreElements()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Created empty enumeration, it has elements: false
To Continue Learning Please Login