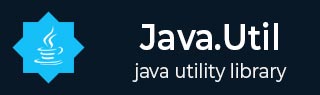
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java.util.BitSet.set() Method
Description
The java.util.BitSet.set(int bitIndex) method sets the bit at the specified index to true.
Declaration
Following is the declaration for java.util.BitSet.set() method
public void set(int bitIndex)
Parameters
bitIndex − a bit index.
Return Value
This method does not return a value.
Exception
IndexOutOfBoundsException − if the specified index is negative.
Example
The following example shows the usage of java.util.BitSet.set() method.
package com.tutorialspoint; import java.util.*; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = new BitSet(8); BitSet bitset2 = new BitSet(8); // assign values to bitset1 bitset1.set(0); bitset1.set(1); bitset1.set(2); bitset1.set(3); bitset1.set(4); bitset1.set(5); // assign values to bitset2 bitset2.set(2); bitset2.set(4); bitset2.set(6); bitset2.set(8); bitset2.set(10); // print the sets System.out.println("Bitset1:" + bitset1); System.out.println("Bitset2:" + bitset2); // set more values bitset1.set(10); bitset1.set(15); bitset2.set(20); bitset2.set(50); // print the sets System.out.println("Bitset1:" + bitset1); System.out.println("Bitset2:" + bitset2); } }
Let us compile and run the above program, this will produce the following result −
Bitset1:{0, 1, 2, 3, 4, 5} Bitset2:{2, 4, 6, 8, 10} Bitset1:{0, 1, 2, 3, 4, 5, 10, 15} Bitset2:{2, 4, 6, 8, 10, 20, 50}
java_util_bitset.htm
Advertisements
To Continue Learning Please Login