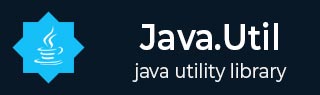
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java BitSet set(int index) Method
Description
The Java BitSet set(int bitIndex) method sets the bit at the specified index to true.
Declaration
Following is the declaration for java.util.BitSet.set() method
public void set(int bitIndex)
Parameters
bitIndex − a bit index.
Return Value
This method does not return a value.
Exception
IndexOutOfBoundsException − if the specified index is negative.
Java BitSet set(int fromIndex, int toIndex) Method
Description
The Java BitSet set(int fromIndex,int toIndex) method sets the bits from the specified fromIndex (inclusive) to the specified toIndex (exclusive) to true.
Declaration
Following is the declaration for java.util.BitSet.set() method
public void set(int fromIndex,int toIndex)
Parameters
fromIndex − index of the first bit to be set.
toIndex − index after the last bit to be set.
Return Value
This method does not return a value.
Exception
IndexOutOfBoundsException − if fromIndex is negative, or toIndex is negative, or fromIndex is larger than toIndex.
Java BitSet set(int bitIndex, boolean value) Method
Description
The java.util.BitSet.set(int bitIndex,boolean value) method sets the bit at the specified index to the specified value.
Declaration
Following is the declaration for java.util.BitSet.set() method
public void set(int bitIndex,boolean value)
Parameters
bitIndex − a bit index.
value − a boolean value to set.
Return Value
This method does not return a value.
Exception
IndexOutOfBoundsException − if the specified index is negative.
Java BitSet set(int fromIndex,int toIndex,boolean value) Method
Description
The Java BitSet set(int fromIndex,int toIndex,boolean value) method sets the bits from the specified fromIndex (inclusive) to the specified toIndex (exclusive) to the specified value.
Declaration
Following is the declaration for java.util.BitSet.set() method
public void set(int fromIndex,int toIndex,boolean value)
Parameters
fromIndex − index of the first bit to be set.
toIndex − index after the last bit to be set.
value − value to set the selected bits to
Return Value
This method does not return a value.
Exception
IndexOutOfBoundsException − if fromIndex is negative, or toIndex is negative, or fromIndex is larger than toIndex.
Setting values of BitSet elements Example
The following example shows the usage of Java BitSet set() methods. We're creating two BitSets. We're setting true values in a BitSet object using set() method call and then use other variants to set values.
package com.tutorialspoint; import java.util.BitSet; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = new BitSet(); BitSet bitset2 = new BitSet(); // assign true values to bitset1 using set(fromIndex, toIndex) bitset1.set(0, 6); // assign false values to bitset1 using set(bitIndex, value) bitset1.set(2, false); // assign true value to bitset2 using set(index) bitset2.set(1); // assign value to bitset2 using set(fromIndex, toIndex, value) bitset2.set(2,5,true); // print the sets System.out.println("Bitset1:" + bitset1); System.out.println("Bitset2:" + bitset2); } }
Output
Let us compile and run the above program, this will produce the following result −
Bitset1:{0, 1, 3, 4, 5} Bitset2:{1, 2, 3, 4}
Setting values of elements of BitSet of Bytes Example
The following example shows the usage of Java BitSet set() methods. We're creating two BitSets using byte[] and using various set() methods, we're setting values in the bitsets.
package com.tutorialspoint; import java.util.BitSet; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = BitSet.valueOf(new byte[] { 0, 1, 2, 3, 4, 5 }); BitSet bitset2 = BitSet.valueOf(new byte[] { 6, 7, 8, 9 }); // assign true values to bitset1 using set(fromIndex, toIndex) bitset1.set(9, 10); // assign false values to bitset1 using set(bitIndex, value) bitset1.set(24, false); // assign true value to bitset2 using set(index) bitset2.set(9); // assign value to bitset2 using set(fromIndex, toIndex, value) bitset2.set(20,24,true); // print the sets System.out.println("Bitset1:" + bitset1); System.out.println("Bitset2:" + bitset2); } }
Output
Let us compile and run the above program, this will produce the following result −
Bitset1:{8, 9, 17, 25, 34, 40, 42} Bitset2:{1, 2, 8, 9, 10, 19, 20, 21, 22, 23, 24, 27}
Setting values of elements of BitSet of Longs Example
The following example shows the usage of Java BitSet set() methods. We're creating two BitSets using long[] and using various set() methods, we're setting values in the bitsets.
package com.tutorialspoint; import java.util.BitSet; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = BitSet.valueOf(new long[] { 0, 1, 2, 3, 4, 5 }); BitSet bitset2 = BitSet.valueOf(new long[] { 6, 7, 8, 9 }); // assign true values to bitset1 using set(fromIndex, toIndex) bitset1.set(9, 10); // assign false values to bitset1 using set(bitIndex, value) bitset1.set(24, false); // assign true value to bitset2 using set(index) bitset2.set(9); // assign value to bitset2 using set(fromIndex, toIndex, value) bitset2.set(20,24,true); // print the sets System.out.println("Bitset1:" + bitset1); System.out.println("Bitset2:" + bitset2); } }
Output
Let us compile and run the above program, this will produce the following result −
Bitset1:{9, 64, 129, 192, 193, 258, 320, 322} Bitset2:{1, 2, 9, 20, 21, 22, 23, 64, 65, 66, 131, 192, 195}
To Continue Learning Please Login