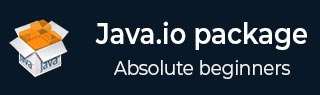
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java.io.StreamTokenizer Class
Introduction
The Java.io.StreamTokenizer class takes an input stream and parses it into "tokens", allowing the tokens to be read one at a time. The stream tokenizer can recognize identifiers, numbers, quoted strings, and various comment styles.
Class declaration
Following is the declaration for Java.io.StreamTokenizer class −
public class StreamTokenizer extends Object
Field
Following are the fields for Java.io.StreamTokenizer class −
double nval − If the current token is a number, this field contains the value of that number.
String sval − If the current token is a word token, this field contains a string giving the characters of the word token.
static int TT_EOF − A constant indicating that the end of the stream has been read.
static int TT_EOL − A constant indicating that the end of the line has been read.
static int TT_NUMBER − A constant indicating that a number token has been read.
static int TT_WORD − A constant indicating that a word token has been read.
int ttype − After a call to the nextToken method, this field contains the type of the token just read.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | StreamTokenizer(Reader r) This creates a tokenizer that parses the given character stream. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | void commentChar(int ch)
Specified that the character argument starts a single-line comment. |
2 | void eolIsSignificant(boolean flag)
This method determines whether or not ends of line are treated as tokens. |
3 | int lineno()
This method returns the current line number. |
4 | void lowerCaseMode(boolean fl)
This method determines whether or not word token are automatically lowercased. |
5 | int nextToken()
This method parses the next token from the input stream of this tokenizer. |
6 | void ordinaryChar(int ch)
This method specifies that the character argument is "ordinary" in this tokenizer. |
7 | void ordinaryChars(int low, int hi)
This method specifies that all characters c in the range low <= c <= high are "ordinary" in this tokenizer. |
8 | void parseNumbers()
This method specifies that numbers should be parsed by this tokenizer. |
9 | void pushBack()
This method causes the next call to the nextToken method of this tokenizer to return the current value in the ttype field, and not to modify the value in the nval or sval field. |
10 | void quoteChar(int ch)
This method specifies that matching pairs of this character delimit string constants in this tokenizer. |
11 | void resetSyntax()
This method resets this tokenizer's syntax table so that all characters are "ordinary." See the ordinaryChar method for more information on a character being ordinary. |
12 | void slashSlashComments(boolean flag)
This method determines whether or not the tokenizer recognizes C++ style comments. |
13 | void slashStarComments(boolean flag)
This method determines whether or not the tokenizer recognizes C style comments. |
14 | String toString()
This method returns the string representation of the current stream token and the line number it occurs on. |
15 | void whitespaceChars(int low, int hi)
This method specifies that all characters c in the range low <= c <= high are white space characters. |
16 | void wordChars(int low, int hi)
This method specifies that all characters c in the range low <= c >= high are word constituents. |
Methods inherited
This class inherits methods from the following classes −
- Java.io.Object