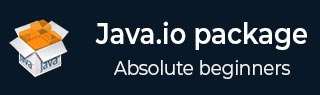
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java.io.DataOutputStream Class
Introduction
The Java.io.DataOutputStream class lets an application write primitive Java data types to an output stream in a portable way. An application can then use a data input stream to read the data back in.
Class declaration
Following is the declaration for Java.io.DataOutputStream class −
public class DataOutputStream extends FilterOutputStream implements DataOutput
Field
Following are the fields for Java.io.DataOutputStream class −
protected int written − This is the number of bytes written to the data output stream so far.
protected OutputStream out − This is the underlying output stream to be filtered.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | DataOutputStream(OutputStream out) This creates a new data output stream to write data to the specified underlying output stream. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | void flush()
This method flushes this data output stream. |
2 | int size()
This method returns the current value of the counter written, the number of bytes written to this data output stream so far. |
3 | void write(byte[] b, int off, int len)
This method writes len bytes from the specified byte array starting at offset off to the underlying output stream. |
4 | void write(int b)
This method writes the specified byte (the low eight bits of the argument b) to the underlying output stream. |
5 | void writeBoolean(boolean v)
This method writes a boolean to the underlying output stream as a 1-byte value. |
6 | void writeByte(int v)
This method writes out a byte to the underlying output stream as a 1-byte value. |
7 | void writeBytes(String s)
This method writes out the string to the underlying output stream as a sequence of bytes. |
8 | void writeChar(int v)
This method writes a char to the underlying output stream as a 2-byte value, high byte first. |
9 | void writeChars(String s)
This method writes a string to the underlying output stream as a sequence of characters. |
10 | void writeDouble(double v)
This method converts the double argument to a long using the doubleToLongBits method in class Double, and then writes that long value to the underlying output stream as an 8-byte quantity, high byte first. |
11 | void writeFloat(float v)
This method converts the float argument to an int using the floatToIntBits method in class Float, and then writes that int value to the underlying output stream as a 4-byte quantity, high byte first. |
12 | void writeInt(int v)
This method writes an int to the underlying output stream as four bytes, high byte first. |
13 | void writeLong(long v)
This method writes a long to the underlying output stream as eight bytes, high byte first. |
14 | void writeShort(int v)
This method writes a short to the underlying output stream as two bytes, high byte first. |
15 | void writeUTF(String str)
This method writes a string to the underlying output stream using modified UTF-8 encoding in a machine-independent manner. |
Methods inherited
This class inherits methods from the following classes −
- Java.io.FilterOutputStream
- Java.io.Object
- Java.io.DataOutput