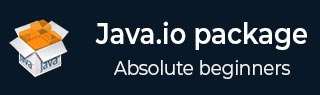
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java - DataOutputStream size()
Description
The Java DataOutputStream size() method returns the current value of the counter written, the number of bytes written to this data output stream so far. If the counter overflows, it will be wrapped to Integer.MAX_VALUE..
Declaration
Following is the declaration for java.io.DataOutputStream.size() method −
public int size()
Parameters
NA
Return Value
The method returns the value of the written field.
Exception
NA
Example 1
The following example shows the usage of Java DataOutputStream size() method. We've created FileOutputStream and DataOutputStream reference. A byte[] buf is initialized with some byte values. A FileOutputStream object is created with a File. Then DataOutputStream is initialized with FileOutputStream object created before. Then byte array is iterated to write byte values to the dataoutputstream. During iteration, we're printing the size of the output stream. Finally we're closing all the streams.
import java.io.DataOutputStream; import java.io.FileOutputStream; import java.io.IOException; public class DataOutputStreamDemo { public static void main(String[] args) throws IOException { FileOutputStream fos = null; DataOutputStream dos = null; byte[] buf = {87,64,72,31,90}; try { // create file output stream fos = new FileOutputStream("F:/test.txt"); // create data output stream dos = new DataOutputStream(fos); int size = 0; // for each byte in the buffer for (byte b:buf) { dos.write(b); // current value of the counter written size = dos.size(); // print System.out.print("Size: "+size + "; "); } } catch(Exception e) { // if any error occurs e.printStackTrace(); } finally { // releases all system resources from the streams if(fos!=null) fos.close(); if(dos!=null) dos.close(); } } }
Output
Let us compile and run the above program, this will produce the following result −
Size: 1; Size: 2; Size: 3; Size: 4; Size: 5;
Example 2
The following example shows the usage of Java DataOutputStream size() method. We've created FileOutputStream and DataOutputStream reference. A byte[] buf is initialized with some byte values. A FileOutputStream object is created with a File. Then DataOutputStream is initialized with FileOutputStream object created before. Then byte array is iterated to write byte values to the dataoutputstream. Once all bytes are written, we're printing the size of the stream. Then as a special case, we're closing the stream and then check the size. It still works and prints the size of the stream. Finally we're closing all the streams.
import java.io.DataOutputStream; import java.io.FileOutputStream; import java.io.IOException; public class DataOutputStreamDemo { public static void main(String[] args) throws IOException { FileOutputStream fos = null; DataOutputStream dos = null; byte[] buf = {87,64,72,31,90}; try { // create file output stream fos = new FileOutputStream("F:/test.txt"); // create data output stream dos = new DataOutputStream(fos); int size = 0; // for each byte in the buffer for (byte b:buf) { dos.write(b); } // current value of the counter written size = dos.size(); // print the size System.out.print("Size: "+size + "; "); // close the stream. dos.close(); // print the size again. System.out.print("Size after closing: "+size + "; "); } catch(Exception e) { // if any error occurs e.printStackTrace(); } finally { // releases all system resources from the streams if(fos!=null) fos.close(); if(dos!=null) dos.close(); } } }
Output
Let us compile and run the above program, this will produce the following result −
Size: 5; Size after closing: 5;