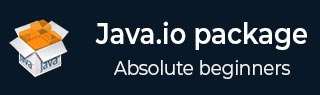
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java - Files Class
Introduction
The Java File class is an abstract representation of file and directory pathnames. Following are the important points about File −
Instances may or may not denote an actual file-system object such as a file or a directory. If it does denote such an object then that object resides in a partition. A partition is an operating system-specific portion of storage for a file system.
A file system may implement restrictions to certain operations on the actual file-system object, such as reading, writing, and executing. These restrictions are collectively known as access permissions.
Instances of the File class are immutable; that is, once created, the abstract pathname represented by a File object will never change.
Class declaration
Following is the declaration for Java.io.File class −
public class File extends Object implements Serializable, Comparable<File>
Field
Following are the fields for Java.io.File class −
static String pathSeparator − This is the system-dependent path-separator character, represented as a string for convenience.
static char pathSeparatorChar − This is the system-dependent path-separator character.
static String separator − This is the system-dependent default name-separator character, represented as a string for convenience.
static char separatorChar − This is the system-dependent default name-separator character.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | File(File parent, String child) This method creates a new File instance from a parent abstract pathname and a child pathname string. |
2 | File(String pathname) This method creates a new File instance by converting the given pathname string into an abstract pathname. |
3 | File(String parent, String child) This method creates a new File instance from a parent pathname string and a child pathname string. |
4 | File(URI uri) This method Creates a new File instance by converting the given file : URI into an abstract pathname. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean canExecute()
This method tests whether the application can execute the file denoted by this abstract pathname. |
2 | boolean canRead()
This method tests whether the application can read the file denoted by this abstract pathname. |
3 | boolean canWrite()
This method tests whether the application can modify the file denoted by this abstract pathname. |
4 | int compareTo(File pathname)
This method compares two abstract pathnames lexicographically. |
5 | boolean createNewFile()
This method atomically creates a new, empty file named by this abstract pathname if and only if a file with this name does not yet exist. |
6 | static File createTempFile(String prefix, String suffix)
This method creates an empty file in the default temporary-file directory, using the given prefix and suffix to generate its name. |
7 | static File createTempFile(String prefix, String suffix, File directory)
This method Creates a new empty file in the specified directory, using the given prefix and suffix strings to generate its name. |
8 | boolean delete()
This method deletes the file or directory denoted by this abstract pathname. |
9 | void deleteOnExit()
This method requests that the file or directory denoted by this abstract pathname be deleted when the virtual machine terminates. |
10 | boolean equals(Object obj)
This method tests this abstract pathname for equality with the given object. |
11 | boolean exists()
This method tests whether the file or directory denoted by this abstract pathname exists. |
12 | File getAbsoluteFile()
This method returns the absolute form of this abstract pathname. |
13 | String getAbsolutePath()
This method returns the absolute pathname string of this abstract pathname. |
14 | File getCanonicalFile()
This method returns the canonical form of this abstract pathname. |
15 | String getCanonicalPath()
This method returns the canonical pathname string of this abstract pathname. |
16 | long getFreeSpace()
This method returns the number of unallocated bytes in the partition named by this abstract path name. |
17 | String getName()
This method returns the name of the file or directory denoted by this abstract pathname. |
18 | String getParent()
This method returns the pathname string of this abstract pathname's parent, or null if this pathname does not name a parent directory. |
19 | File getParentFile()
This method returns the abstract pathname of this abstract pathname's parent, or null if this pathname does not name a parent directory. |
20 | String getPath()
This method converts this abstract pathname into a pathname string. |
21 | long getTotalSpace()
This method returns the size of the partition named by this abstract pathname. |
22 | long getUsableSpace()
This method returns the number of bytes available to this virtual machine on the partition named by this abstract pathname. |
23 | int hashCode()
This method computes a hash code for this abstract pathname. |
24 | boolean isAbsolute()
This method tests whether this abstract pathname is absolute. |
25 | boolean isDirectory()
This method tests whether the file denoted by this abstract pathname is a directory. |
26 | boolean isFile()
This method tests whether the file denoted by this abstract pathname is a normal file. |
27 | boolean isHidden()
This method tests whether the file named by this abstract pathname is a hidden file. |
28 | long lastModified()
This method returns the time that the file denoted by this abstract pathname was last modified. |
29 | long length()
This method returns the length of the file denoted by this abstract pathname. |
30 | String[] list()
This method returns an array of strings naming the files and directories in the directory denoted by this abstract pathname. |
31 | String[] list(FilenameFilter filter)
This method returns an array of strings naming the files and directories in the directory denoted by this abstract pathname that satisfy the specified filter. |
32 | File[] listFiles()
This method returns an array of abstract pathnames denoting the files in the directory denoted by this abstract pathname. |
33 | File[] listFiles(FileFilter filter)
This method returns an array of abstract pathnames denoting the files and directories in the directory denoted by this abstract pathname that satisfy the specified filter. |
34 | File[] listFiles(FilenameFilter filter)
This method returns an array of abstract pathnames denoting the files and directories in the directory denoted by this abstract pathname that satisfy the specified filter. |
35 | static File[] listRoots()
This method lists the available filesystem roots. |
36 | boolean mkdir()
This method creates the directory named by this abstract pathname. |
37 | boolean mkdirs()
This method creates the directory named by this abstract pathname, including any necessary but non existent parent directories. |
38 | boolean renameTo(File dest)
This method renames the file denoted by this abstract pathname. |
39 | boolean setExecutable(boolean executable)
This is a convenience method to set the owner's execute permission for this abstract pathname. |
40 | boolean setExecutable(boolean executable, boolean ownerOnly)
This method Sets the owner's or everybody's execute permission for this abstract pathname. |
41 | boolean setLastModified(long time)
This method sets the last-modified time of the file or directory named by this abstract pathname. |
42 | boolean setReadable(boolean readable)
This is a convenience method to set the owner's read permission for this abstract pathname. |
43 | boolean setReadable(boolean readable, boolean ownerOnly)
This method sets the owner's or everybody's read permission for this abstract pathname. |
44 | boolean setReadOnly()
This method marks the file or directory named by this abstract pathname so that only read operations are allowed. |
45 | boolean setWritable(boolean writable)
This is a convenience method to set the owner's write permission for this abstract pathname. |
46 | boolean setWritable(boolean writable, boolean ownerOnly)
This method sets the owner's or everybody's write permission for this abstract pathname. |
47 | String toString()
This method returns the pathname string of this abstract pathname. |
48 | URI toURI()
This method constructs a file : URI that represents this abstract pathname. |
Methods inherited
This class inherits methods from the following classes −
- Java.io.Object
Example
Following is an example to demonstrate File object − We've create File reference and String array of two strings representing file name. Then we've created a file object and using File.canExecute() and File.getAbsolutePath() and printed the corresponding values.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; String[] strs = {"test1.txt", "test2.txt"}; try { // for each string in string array for(String s:strs ) { // create new file f = new File(s); // true if the file is executable boolean bool = f.canExecute(); // find the absolute path String a = f.getAbsolutePath(); // prints absolute path System.out.print(a); // prints System.out.println(" is executable: "+ bool); } } catch (Exception e) { // if any I/O error occurs e.printStackTrace(); } } }
Consider there is an executable file test1.txt and another file test2.txt is non executable in the current directory. Let us compile and run the above program, This will produce the following result −
Output
F:\Workspace\Tester\test1.txt is executable: true F:\Workspace\Tester\test2.txt is executable: false