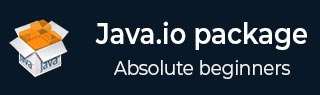
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java - File delete() Method
Description
The Java File delete() method deletes the file or directory defined by the abstract path name. To delete a directory, the directory must be empty.
Declaration
Following is the declaration for java.io.File.delete() method −
public boolean delete()
Parameters
NA
Return Value
This method returns true if the file is successfully deleted, else false.
Exception
SecurityException − If SecurityManager.checkWrite(java.lang.String) method denies delete access to the file
Example 1
The following example shows the usage of Java File delete() method. We've created a File reference and then we've initialized it with File object using "test.txt" file in the current directory. Here this file is not present in the current directory. Thus using delete() method, we're getting result as false. Then we've created the file first using createNewFile() and then using delete() method, we're deleting the file and result returned is true.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new file f = new File("test.txt"); // tries to delete a non-existing file bool = f.delete(); // prints the status System.out.println("File deleted: "+bool); // creates file in the system f.createNewFile(); // createNewFile() is invoked System.out.println("createNewFile() method is invoked"); // tries to delete the newly created file bool = f.delete(); // print the status System.out.println("File deleted: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
File deleted: false createNewFile() method is invoked File deleted: true
Example 2
The following example shows the usage of Java File delete() method. We've created a File reference and then we've initialized it with File object using "test" path in the current directory. Here this directory is not present in the current directory. Thus using delete() method, we're getting result as false. Then we've created the directory first using mkdir() and then using delete() method, we're deleting the directory and result returned is true.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new file for test directory f = new File("test"); // tries to delete a non-existing directory bool = f.delete(); // prints the status System.out.println("Directory deleted: "+bool); // creates directory in the system f.mkdir(); // createNewFile() is invoked System.out.println("mkdir() method is invoked"); // tries to delete the newly created directory bool = f.delete(); // print the status System.out.println("Directory deleted: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
Directory deleted: false mkdir() method is invoked Directory deleted: true
Example 3
The following example shows the usage of Java File delete() method. We've created a File reference and then we've initialized it with File object using "F:/Test/Test2" path. Here this directory is not empty and contains a sample file. Thus using delete() method, we're getting result as false.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new file for test directory f = new File("F:/Test/Test2"); // tries to delete a non-empty directory bool = f.delete(); // prints the status System.out.println("Directory deleted: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
Directory deleted: false