
- DirectX Tutorial
- DirectX - Home
- 3D MATHS
- 3D Maths - Vector Algebra
- 3D Maths - Matrix Algebra
- 3D Maths - Transformations
- 3D Maths - DirectX Math
- DIRECTX
- DirectX - Overview
- DirectX - Installation
- DirectX - Components
- DirectX - Tools
- DirectX - Creating App
- DirectX - Window Events
- DirectX - App Lifecycle
- DirectX - Direct3D Initialization
- DirectX - First HLSL
- DirectX - Graphics Pipeline
- DirectX - Buffers
- DirectX - 3D Transformation
- DirectX - Drawing
- DirectX - Rendering
- DirectX - Modeling
- DirectX - Lighting
- DirectX - Texturing
- DirectX - Multi Texturing
- DirectX - Blending
- DirectX - Picking
- DirectX - Stenciling
- DirectX - First Shader
- DirectX - Pixel Shader
- DirectX - Geometry Shaders
- DirectX - Compute Shaders
- DirectX - Shader Effects
- DirectX - Quaternion
- DirectX Resources
- DirectX - Quick Guide
- DirectX - Useful Resources
- DirectX - Discussion
DirectX - Window Events
This chapter will focus on the events used in DirectX. It includes various user actions which can be detected by Windows. Whenever a particular event occurs, a notification is sent which describes about the event.
The life cycle of processing the events is mentioned below −
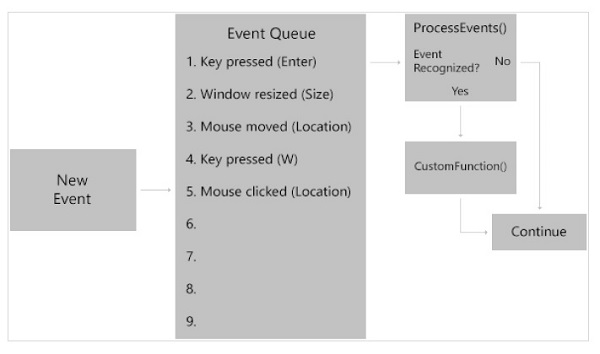
Whenever a new event is created, it is kept in queue. The events are listed priority-wise in the mentioned queue. ProcessEvents() is the function which will verify if the event is recognized or not. If yes it will move to CustomFunction() which focuses on further steps.
Handling events focuses on three basic parts −
- Instructing the window to dispatch the respective events
- Writing the functions to get the desired events
- Giving information to Windows about the list of functions
Now let us focus on each one and understand its importance.
Instruction to dispatch all events
Dispatching events is considered to be very simple. It is done with ProcessEvents() function. The code snippet for understanding this function is mentioned below −
virtual void Run() { // Obtain a pointer to the window CoreWindow^ Window = CoreWindow::GetForCurrentThread(); // Run ProcessEvents() to dispatch events Window->Dispatcher->ProcessEvents(CoreProcessEventsOption::ProcessUntilQuit); }
Run() refers to the initialization of event. GetForCurrentThread() is used to get a pointer to the window. Once the pointer is defined, it is considered to be the member of CoreWindow class. It automatically helps in processing events which is highlighted in the code snippet mentioned above.
Functions for the desired events
DirectX includes lots of events that can occur; but in this section, we will be interested only in specific events.
There is a special event called PointerPressed. It triggers when the user either clicks the mouse or when the touchscreen is touched with a pen or a finger. The event function need two major parameters as defined below −
void PointerPressed(CoreWindow^ Window , PointerEventArgs^ Args) { }
The first parameter is CoreWindow which allows us to access information about the window. The second parameter is considered to be secondary and mentions about the pointer-press such as what type of press should be created i.e., mouse, pen and touch.
Information to Windows about the list of functions
In this section, we will focus on setting the parameters to give information to Windows about the list of functions.
Consider the code snippet mentioned below −
virtual void SetWindow(CoreWindow^ Window) { Window->PointerPressed += ref new TypedEventHandler <CoreWindow^, PointerEventArgs^>(this, &App::PointerPressed); }
The CoreWindow parameter calls for PointerPressed which refers to the TypeEventHandler, thus specifying information to Windows with the list of functions.
To Continue Learning Please Login