
- DirectX Tutorial
- DirectX - Home
- 3D MATHS
- 3D Maths - Vector Algebra
- 3D Maths - Matrix Algebra
- 3D Maths - Transformations
- 3D Maths - DirectX Math
- DIRECTX
- DirectX - Overview
- DirectX - Installation
- DirectX - Components
- DirectX - Tools
- DirectX - Creating App
- DirectX - Window Events
- DirectX - App Lifecycle
- DirectX - Direct3D Initialization
- DirectX - First HLSL
- DirectX - Graphics Pipeline
- DirectX - Buffers
- DirectX - 3D Transformation
- DirectX - Drawing
- DirectX - Rendering
- DirectX - Modeling
- DirectX - Lighting
- DirectX - Texturing
- DirectX - Multi Texturing
- DirectX - Blending
- DirectX - Picking
- DirectX - Stenciling
- DirectX - First Shader
- DirectX - Pixel Shader
- DirectX - Geometry Shaders
- DirectX - Compute Shaders
- DirectX - Shader Effects
- DirectX - Quaternion
- DirectX Resources
- DirectX - Quick Guide
- DirectX - Useful Resources
- DirectX - Discussion
DirectX - Lighting
DirectX is known for creating lights which is similar to real world lighting. It includes a system of varied calculation which is less time consuming and demonstrates the real world system. Lighting is considered as an important part of 3D game programming. Lighting helps user to bring the realism out and make it as a real time experience. The DirectX system includes built up of various set of types like lightning and types of light sources. Now, it is important to focus on three types of lightning as mentioned below −
- Diffuse Lighting
- Ambient Lighting
- Specular Lighting
Diffuse Lighting
Diffuse light is a dim light which falls on the surface. The best demonstration is a user holding up hand with this light source. It will be visible that one side of hand is lit while the other side is not. The side which is lit is called as diffuse light and the procedure is called diffuse lighting.
Ambient Lighting
Ambient light refers to the light which spreads everywhere. As per the previous example, holding up the arm with one light source, the dark side is called as lightly lit or ambient lit. The light which is reflected is considered as ambient light and the process is called as ambient lighting.
Specular Light
Specular light is also called as specular highlight or even more commonly as reflection. When light reflects off the required object, usually most of the light will go in one specific direction.
Creating Lights
This section includes the process of creating lights which is often used for creating lights. Following are the important steps needed for creating lights −
- Starting with new flexible format
- Process of turning light on
- Ambient light setup
- Diffuse light setup
- Setting the material standards
The first three are considered as simple processes, but the last two will require a whole function to themselves.
Starting with new flexible format
Once again, we will focus on the FVF code and the vertex format will change. This time we are focusing on the diffuse color and add a new property in the vertex normal. The new FVF code and vertex format is mentioned below −
struct CUSTOMVERTEX {FLOAT X, Y, Z; D3DVECTOR NORMAL;}; #define CUSTOMFVF (D3DFVF_XYZ | D3DFVF_NORMAL) D3DVECTOR NORMAL; #definition of the structure typedef struct D3DVECTOR { float x, y, z; } D3DVECTOR, *LPD3DVECTOR;
Process of turning light on
The function call is already discussed in the previous sections. The process of activating the lights on is given in the following code −
d3ddev->SetRenderState(D3DRS_LIGHTING, TRUE);
Ambient Light setup
This step uses the same particular function, SetRenderState which is considered as the first parameter that changes to a different flag, the second parameter is D3DRS_AMBIENT which is color of the ambient light.
d3ddev->SetRenderState(D3DRS_AMBIENT, D3DCOLOR_XRGB(50, 50, 50)); // ambient light
Diffusion of light setup
This step is longer than normal. It is important for the properties of the size and flexibility of this step, with its own function called own_light(). It is given below −
this is the function that sets up the lights and materials void init_light(void){ D3DLIGHT9 light; // creating the structure of light ZeroMemory(&light, sizeof(light)); // following zero memory pattern light.Type = D3DLIGHT_DIRECTIONAL; // creating the light type light.Diffuse = D3DXCOLOR(0.5f, 0.5f, 0.5f, 1.0f); // setting up the color light.Direction = D3DXVECTOR3(-1.0f, -0.3f, -1.0f); d3ddev->SetLight(0, &light); // send the light struct properties to light #0 d3ddev->LightEnable(0, TRUE); // turn on light #0 }
D3DLIGHT9
D3DLIGHT9 is a struct data which includes all the information about a light, no matter what its type is.
The demonstration of the lit cube is mentioned below −
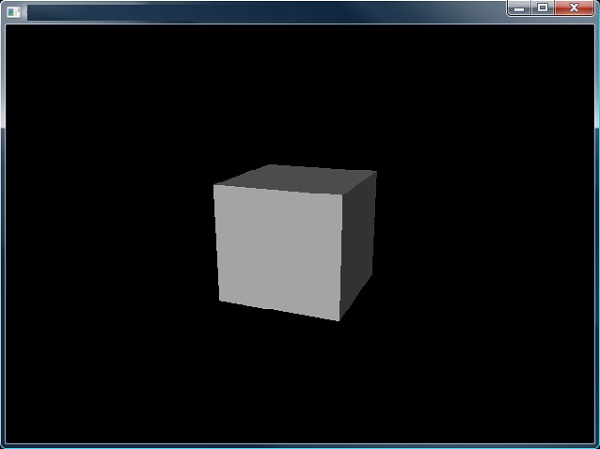
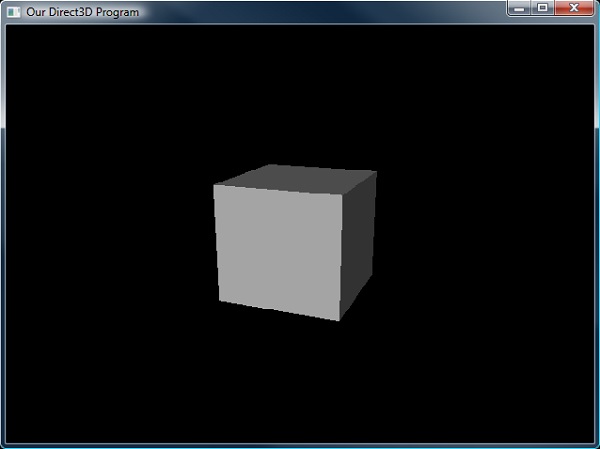
To Continue Learning Please Login