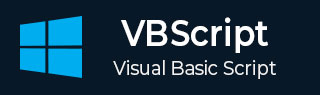
- VBScript Tutorial
- VBScript - Home
- VBScript - Overview
- VBScript - Syntax
- VBScript - Enabling
- VBScript - Placement
- VBScript - Variables
- VBScript - Constants
- VBScript - Operators
- VBScript - Decisions
- VBScript - Loops
- VBScript - Events
- VBScript - Cookies
- VBScript - Numbers
- VBScript - Strings
- VBScript - Arrays
- VBScript - Date
- VBScript Advanced
- VBScript - Procedures
- VBScript - Dialog Boxes
- VBScript - Object Oriented
- VBScript - Reg Expressions
- VBScript - Error Handling
- VBScript - Misc Statements
- VBScript Useful Resources
- VBScript - Questions and Answers
- VBScript - Quick Guide
- VBScript - Useful Resources
- VBScript - Discussion
VBScript - Quick Guide
VBScript - Overview
VBScript stands for Visual Basic Scripting that forms a subset of Visual Basic for Applications (VBA). VBA is a product of Microsoft which is included NOT only in other Microsoft products such as MS Project and MS Office but also in Third Party tools such as AUTO CAD.
Features of VBScript
VBScript is a lightweight scripting language, which has a lightning fast interpreter.
VBScript, for the most part, is case insensitive. It has a very simple syntax, easy to learn and to implement.
Unlike C++ or Java, VBScript is an object-based scripting language and NOT an Object-Oriented Programming language.
It uses Component Object Model (COM) in order to access the elements of the environment in which it is executing.
Successful execution of VBScript can happen only if it is executed in Host Environment such as Internet Explorer (IE), Internet Information Services (IIS) and Windows Scripting Host (WSH)
VBscript – Version History and Uses
VBScript was introduced by Microsoft way back in 1996 and its first version was 1.0. The current stable version of VBScript is 5.8, which is available as part of IE8 or Windows 7. The VBScript usage areas are aplenty and not restricted to the below list.
VBScript is used as a scripting language in one of the popular Automation testing tools – Quick Test Professional abbreviated as QTP
Windows Scripting Host, which is used mostly by Windows System administrators for automating the Windows Desktop.
Active Server Pages (ASP), a server side scripting environment for creating dynamic webpages which uses VBScript or Java Script.
VBScript is used for Client side scripting in Microsoft Internet Explorer.
Microsoft Outlook Forms usually runs on VBScript; however, the application level programming relies on VBA (Outlook 2000 onwards).
Disadvantages
VBscript is used only by IE Browsers. Other browsers such as Chrome, Firefox DONOT Support VBScript. Hence, JavaScript is preferred over VBScript.
VBScript has a Limited command line support.
Since there is no development environment available by default, debugging is difficult.
Where VBScript is Today ?
The current version of VBScript is 5.8, and with the recent development of .NET framework, Microsoft has decided to provide future support of VBScript within ASP.NET for web development. Hence, there will NOT be any more new versions of VBScript engine but the entire defect fixes and security issues are being addressed by the Microsoft sustaining Engineering Team. However, VBScript engine would be shipped as part of all Microsoft Windows and IIS by default.
VBScript - Syntax
Your First VBScript
Let us write a VBScript to print out "Hello World".
<html> <body> <script language = "vbscript" type = "text/vbscript"> document.write("Hello World!") </script> </body> </html>
In the above example, we called a function document.write, which writes a string into the HTML document. This function can be used to write text, HTML or both. So, above code will display following result −
Hello World!
Whitespace and Line Breaks
VBScript ignores spaces, tabs, and newlines that appear within VBScript programs. One can use spaces, tabs, and newlines freely within the program, so you are free to format and indent your programs in a neat and consistent way that makes the code easy to read and understand.
Formatting
VBScript is based on Microsoft's Visual Basic. Unlike JavaScript, no statement terminators such as semicolon is used to terminate a particular statement.
Single Line Syntax
Colons are used when two or more lines of VBScript ought to be written in a single line. Hence, in VBScript, Colons act as a line separator.
<script language = "vbscript" type = "text/vbscript"> var1 = 10 : var2 = 20 </script>
Multiple Line Syntax
When a statement in VBScript is lengthy and if user wishes to break it into multiple lines, then the user has to use underscore "_". This improves the readability of the code. The following example illustrates how to work with multiple lines.
<script language = "vbscript" type = "text/vbscript"> var1 = 10 var2 = 20 Sum = var1 + var2 document.write("The Sum of two numbers"&_"var1 and var2 is " & Sum) </script>
Reserved Words
The following list shows the reserved words in VBScript. These reserved words SHOULD NOT be used as a constant or variable or any other identifier names.
Loop | LSet | Me |
Mod | New | Next |
Not | Nothing | Null |
On | Option | Optional |
Or | ParamArray | Preserve |
Private | Public | RaiseEvent |
ReDim | Rem | Resume |
RSet | Select | Set |
Shared | Single | Static |
Stop | Sub | Then |
To | True | Type |
And | As | Boolean |
ByRef | Byte | ByVal |
Call | Case | Class |
Const | Currency | Debug |
Dim | Do | Double |
Each | Else | ElseIf |
Empty | End | EndIf |
Enum | Eqv | Event |
Exit | False | For |
Function | Get | GoTo |
If | Imp | Implements |
In | Integer | Is |
Let | Like | Long |
TypeOf | Until | Variant |
Wend | While | With |
Xor | Eval | Execute |
Msgbox | Erase | ExecuteGlobal |
Option Explicit | Randomize | SendKeys |
Case Sensitivity
VBScript is a case-insensitive language. This means that language keywords, variables, function names and any other identifiers need NOT be typed with a consistent capitalization of letters. So identifiers int_counter, INT_Counter and INT_COUNTER have the same meaning within VBScript.
Comments in VBScript
Comments are used to document the program logic and the user information with which other programmers can seamlessly work on the same code in future. It can include information such as developed by, modified by and it can also include incorporated logic. Comments are ignored by the interpreter while execution. Comments in VBScript are denoted by two methods.
1. Any statement that starts with a Single Quote (‘) is treated as comment.
Following is the example −
<script language = "vbscript" type = "text/vbscript"> <!— ' This Script is invoked after successful login ' Written by : TutorialsPoint ' Return Value : True / False //- > </script>
2. Any statement that starts with the keyword “REM”.
Following is the example −
<script language = "vbscript" type = "text/vbscript"> <!— REM This Script is written to Validate the Entered Input REM Modified by : Tutorials point/user2 //- > </script>
Enabling VBScript in Browsers
Not all the modern browsers support VBScript. VBScript is supported just by Microsoft's Internet Explorer while other browsers (Firefox and Chrome) support just JavaScript. Hence, developers normally prefer JavaScript over VBScript.
Though Internet Explorer (IE) supports VBScript, you may need to enable or disable this feature manually. This tutorial will make you aware of the procedure of enabling and disabling VBScript support in Internet Explorer.
VBScript in Internet Explorer
Here are simple steps to turn on or turn off VBScript in your Internet Explorer −
Follow Tools → Internet Options from the menu
Select Security tab from the dialog box
Click the Custom Level button
Scroll down till you find Scripting option
Select Enable radio button under Active scripting
Finally click OK and come out
To disable VBScript support in your Internet Explorer, you need to select Disable radio button under Active scripting.
VBScript - Placements
VBScript Placement in HTML File
There is a flexibility given to include VBScript code anywhere in an HTML document. But the most preferred way to include VBScript in your HTML file is as follows −
Script in <head>...</head> section.
Script in <body>...</body> section.
Script in <body>...</body> and <head>...</head> sections.
Script in an external file and then include in <head>...</head> section.
In the following section, we will see how we can put VBScript in different ways −
VBScript in <head>...</head> section
If you want to have a script run on some event, such as when a user clicks somewhere, then you will place that script in the head as follows −
<html> <head> <script type = "text/Vbscript"> <!-- Function sayHello() Msgbox("Hello World") End Function //--> </script> </head> <body> <input type = "button" onclick = "sayHello()" value = "Say Hello" /> </body> </html>
It will produce the following result − A button with the name SayHello. Upon clicking on the Button, the message box is displayed to the user with the message "Hello World".
VBScript in <body>...</body> section
If you need a script to run as the page loads so that the script generates content in the page, the script goes in the <body> portion of the document. In this case, you would not have any function defined using VBScript −
<html> <head> </head> <body> <script type = "text/vbscript"> <!-- document.write("Hello World") //--> </script> <p>This is web page body </p> </body> </html>
This will produce the following result −
Hello World This is web page body
VBScript in <body> and <head> sections
You can put your VBScript code in <head> and <body> section altogether as follows −
<html> <head> <script type = "text/vbscript"> <!-- Function sayHello() msgbox("Hello World") End Function //--> </script> </head> <body> <script type = "text/vbscript"> <!-- document.write("Hello World") //--> </script> <input type = "button" onclick = "sayHello()" value = "Say Hello" /> </body> </html>
It will produce the following result − Hello World message with a 'Say Hello' button. Upon Clicking on the button a message box with a message "Hello World" is displayed to the user.
Hello World
VBScript in External File
As you begin to work more extensively with VBScript, you will likely find that there are cases, where you are reusing identical VBScript code on multiple pages of a site. You are not restricted to be maintaining identical code in multiple HTML files.
The script tag provides a mechanism to allow you to store VBScript in an external file and then include it into your HTML files. Here is an example to show how you can include an external VBScript file in your HTML code using script tag and its src attribute −
<html> <head> <script type = "text/vbscript" src = "filename.vbs" ></script> </head> <body> ....... </body> </html>
To use VBScript from an external file source, you need to write your all VBScript source code in a simple text file with extension ".vbs" and then include that file as shown above. For example, you can keep the following content in filename.vbs file and then you can use sayHello function in your HTML file after including filename.vbs file.
Function sayHello() Msgbox "Hello World" End Function
VBScript Placement in QTP
VBScript is placed in QTP (Quick Test Professional) tool but it is NOT enclosed within HTML Tags. The Script File is saved with the extension .vbs and it is executed by Quick Test Professional execution engine.
VBScript - Variables
VBScript Variables
A variable is a named memory location used to hold a value that can be changed during the script execution. VBScript has only ONE fundamental data type, Variant.
Rules for Declaring Variables −
Variable Name must begin with an alphabet.
Variable names cannot exceed 255 characters.
Variables Should NOT contain a period (.)
Variable Names should be unique in the declared context.
Declaring Variables
Variables are declared using “dim” keyword. Since there is only ONE fundamental data type, all the declared variables are variant by default. Hence, a user NEED NOT mention the type of data during declaration.
Example 1 − In this Example, IntValue can be used as a String, Integer or even arrays.
Dim Var
Example 2 − Two or more declarations are separated by comma(,)
Dim Variable1,Variable2
Assigning Values to the Variables
Values are assigned similar to an algebraic expression. The variable name on the left hand side followed by an equal to (=) symbol and then its value on the right hand side.
Rules
The numeric values should be declared without double quotes.
The String values should be enclosed within double quotes(")
Date and Time variables should be enclosed within hash symbol(#)
Examples
' Below Example, The value 25 is assigned to the variable. Value1 = 25 ' A String Value ‘VBScript’ is assigned to the variable StrValue. StrValue = “VBScript” ' The date 01/01/2020 is assigned to the variable DToday. Date1 = #01/01/2020# ' A Specific Time Stamp is assigned to a variable in the below example. Time1 = #12:30:44 PM#
Scope of the Variables
Variables can be declared using the following statements that determines the scope of the variable. The scope of the variable plays a crucial role when used within a procedure or classes.
- Dim
- Public
- Private
Dim
Variables declared using “Dim” keyword at a Procedure level are available only within the same procedure. Variables declared using “Dim” Keyword at script level are available to all the procedures within the same script.
Example − In the below example, the value of Var1 and Var2 are declared at script level while Var3 is declared at procedure level.
Note − The scope of this chapter is to understand Variables. Functions would be dealt in detail in the upcoming chapters.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim Var1 Dim Var2 Call add() Function add() Var1 = 10 Var2 = 15 Dim Var3 Var3 = Var1 + Var2 Msgbox Var3 'Displays 25, the sum of two values. End Function Msgbox Var1 ' Displays 10 as Var1 is declared at Script level Msgbox Var2 ' Displays 15 as Var2 is declared at Script level Msgbox Var3 ' Var3 has No Scope outside the procedure. Prints Empty </script> </body> </html>
Public
Variables declared using "Public" Keyword are available to all the procedures across all the associated scripts. When declaring a variable of type "public", Dim keyword is replaced by "Public".
Example − In the following example, Var1 and Var2 are available at script level while Var3 is available across the associated scripts and procedures as it is declared as Public.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim Var1 Dim Var2 Public Var3 Call add() Function add() Var1 = 10 Var2 = 15 Var3 = Var1+Var2 Msgbox Var3 'Displays 25, the sum of two values. End Function Msgbox Var1 ' Displays 10 as Var1 is declared at Script level Msgbox Var2 ' Displays 15 as Var2 is declared at Script level Msgbox Var3 ' Displays 25 as Var3 is declared as Public </script> </body> </html>
Private
Variables that are declared as "Private" have scope only within that script in which they are declared. When declaring a variable of type "Private", Dim keyword is replaced by "Private".
Example − In the following example, Var1 and Var2 are available at Script Level. Var3 is declared as Private and it is available only for this particular script. Use of "Private" Variables is more pronounced within the Class.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim Var1 Dim Var2 Private Var3 Call add() Function add() Var1 = 10 Var2 = 15 Var3 = Var1+Var2 Msgbox Var3 'Displays the sum of two values. End Function Msgbox Var1 ' Displays 10 as Var1 is declared at Script level Msgbox Var2 ' Displays 15 as Var2 is declared at Script level Msgbox Var3 ' Displays 25 but Var3 is available only for this script. </script> </body> </html>
VBScript - Constants
Constant is a named memory location used to hold a value that CANNOT be changed during the script execution. If a user tries to change a Constant Value, the Script execution ends up with an error. Constants are declared the same way the variables are declared.
Declaring Constants
Syntax
[Public | Private] Const Constant_Name = Value
The Constant can be of type Public or Private. The Use of Public or Private is Optional. The Public constants are available for all the scripts and procedures while the Private Constants are available within the procedure or Class. One can assign any value such as number, String or Date to the declared Constant.
Example 1
In this example, the value of pi is 3.4 and it displays the area of the circle in a message box.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim intRadius intRadius = 20 const pi = 3.14 Area = pi*intRadius*intRadius Msgbox Area </script> </body> </html>
Example 2
The below example illustrates how to assign a String and Date Value to a Constant.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Const myString = "VBScript" Const myDate = #01/01/2050# Msgbox myString Msgbox myDate </script> </body> </html>
Example 3
In the below example, the user tries to change the Constant Value; hence, it will end up with an Execution Error.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim intRadius intRadius = 20 const pi = 3.14 pi = pi*pi 'pi VALUE CANNOT BE CHANGED.THROWS ERROR' Area = pi*intRadius*intRadius Msgbox Area </script> </body> </html>
VBScript - Operators
What is an operator?
Let’s take an expression 4 + 5 is equal to 9. Here, 4 and 5 are called operands and + is called the operator. VBScript language supports following types of operators −
- Arithmetic Operators
- Comparison Operators
- Logical (or Relational) Operators
- Concatenation Operators
The Arithmetic Operators
VBScript supports the following arithmetic operators −
Assume variable A holds 5 and variable B holds 10, then −
Operator | Description | Example |
---|---|---|
+ | Adds two operands | A + B will give 15 |
- | Subtracts second operand from the first | A - B will give -5 |
* | Multiply both operands | A * B will give 50 |
/ | Divide numerator by denumerator | B / A will give 2 |
% | Modulus Operator and remainder of after an integer division | B MOD A will give 0 |
^ | Exponentiation Operator | B ^ A will give 100000 |
To understand these operators in a better way, you can Try it yourself.
The Comparison Operators
There are following comparison operators supported by VBScript language −
Assume variable A holds 10 and variable B holds 20, then −
Operator | Description | Example |
---|---|---|
= | Checks if the value of two operands are equal or not, if yes then condition becomes true. | (A == B) is False. |
<> | Checks if the value of two operands are equal or not, if values are not equal then condition becomes true. | (A <> B) is True. |
> | Checks if the value of left operand is greater than the value of right operand, if yes then condition becomes true. | (A > B) is False. |
< | Checks if the value of left operand is less than the value of right operand, if yes then condition becomes true. | (A < B) is True. |
>= | Checks if the value of left operand is greater than or equal to the value of right operand, if yes then condition becomes true. | (A >= B) is False. |
<= | Checks if the value of left operand is less than or equal to the value of right operand, if yes then condition becomes true. | (A <= B) is True. |
To understand these operators in a better way, you can Try it yourself.
The Logical Operators
There are following logical operators supported by VBScript language −
Assume variable A holds 10 and variable B holds 0, then −
Operator | Description | Example |
---|---|---|
AND | Called Logical AND operator. If both the conditions are True, then Expression becomes True. | a<>0 AND b<>0 is False. |
OR | Called Logical OR Operator. If any of the two conditions is True, then condition becomes True. | a<>0 OR b<>0 is true. |
NOT | Called Logical NOT Operator. It reverses the logical state of its operand. If a condition is True, then the Logical NOT operator will make it False. | NOT(a<>0 OR b<>0) is false. |
XOR | Called Logical Exclusion. It is the combination of NOT and OR Operator. If one, and only one, of the expressions evaluates to True, result is True. | (a<>0 XOR b<>0) is true. |
To understand these operators in a better way, you can Try it yourself.
The Concatenation Operators
There are following Concatenation operators supported by VBScript language −
Assume variable A holds 5 and variable B holds 10 then −
Operator | Description | Example |
---|---|---|
+ | Adds two Values as Variable Values are Numeric | A + B will give 15 |
& | Concatenates two Values | A & B will give 510 |
Assume variable A = "Microsoft" and variable B="VBScript", then −
Operator | Description | Example |
---|---|---|
+ | Concatenates two Values | A + B will give MicrosoftVBScript |
& | Concatenates two Values | A & B will give MicrosoftVBScript |
Note − Concatenation Operators can be used for numbers and strings. The Output depends on the context if the variables hold numeric value or String Value.
To understand these Operators in a better way, you can Try it yourself.
VBScript - Decision Making
Decision making allows programmers to control the execution flow of a script or one of its sections. The execution is governed by one or more conditional statements.
Following is the general form of a typical decision making structure found in most of the programming languages −
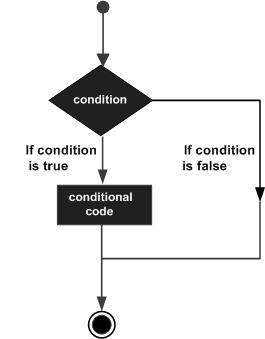
VBScript provides the following types of decision making statements.
Statement | Description |
---|---|
if statement | An if statement consists of a Boolean expression followed by one or more statements. |
if..else statement | An if else statement consists of a Boolean expression followed by one or more statements. If the condition is True, the statements under the If statements are executed. If the condition is false, then the Else part of the script is Executed |
if...elseif..else statement | An if statement followed by one or more ElseIf Statements, that consists of Boolean expressions and then followed by an optional else statement, which executes when all the condition becomes false. |
nested if statements | An if or elseif statement inside another if or elseif statement(s). |
switch statement | A switch statement allows a variable to be tested for equality against a list of values. |
VBScript - Loops
There may be a situation when you need to execute a block of code several number of times. In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
Programming languages provide various control structures that allow more complicated execution paths. A loop statement allows us to execute a statement or group of statements multiple times and following is the general from of a loop statement in VBScript.
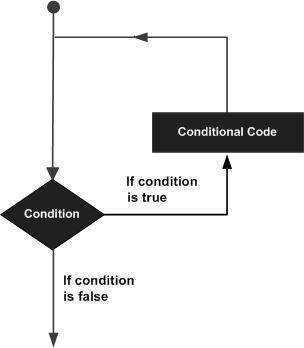
VBScript provides the following types of loops to handle looping requirements. Click the following links to check their detail.
Loop Type | Description |
---|---|
for loop | Executes a sequence of statements multiple times and abbreviates the code that manages the loop variable. |
for ..each loop | It is executed if there is at least one element in group and reiterated for each element in a group. |
while..wend loop | It tests the condition before executing the loop body. |
do..while loops | The do..While statements will be executed as long as condition is True.(i.e.,) The Loop should be repeated till the condition is False. |
do..until loops | The do..Until statements will be executed as long as condition is False.(i.e.,) The Loop should be repeated till the condition is True. |
Loop Control Statements
Loop control statements change execution from its normal sequence. When execution leaves a scope, all the remaining statements in the loop are NOT executed.
VBScript supports the following control statements. Click the following links to check their detail.
Control Statement | Description |
---|---|
Exit For statement | Terminates the For loop statement and transfers execution to the statement immediately following the loop |
Exit Do statement | Terminates the Do While statement and transfers execution to the statement immediately following the loop |
VBScript - Events
What is an Event ?
VBScript's interaction with HTML is handled through events that occur when the user or browser manipulates a page. When the page loads, that is an event. When the user clicks a button, that click too is an event. Other examples of events include pressing any key, closing window, resizing window, etc. Developers can use these events to execute VBScript coded responses, which cause buttons to close windows, messages to be displayed to users, data to be validated, and virtually any other type of response imaginable to occur.
Events are a part of the Document Object Model (DOM) and every HTML element has a certain set of events, which can trigger VBScript Code. Please go through this small tutorial for a better understanding HTML Event Reference. Here, we will see few examples to understand a relation between Event and VBScript.
onclick Event Type
This is the most frequently used event type, which occurs when a user clicks mouse's left button. You can put your validation, warning, etc., against this event type.
Example
<html> <head> <script language = "vbscript" type = "text/vbscript"> Function sayHello() msgbox "Hello World" End Function </script> </head> <body> <input type = "button" onclick = "sayHello()" value = "Say Hello"/> </body> </html>
It will produce the following result, and when you click the Hello button, the onclick event will occur which will trigger sayHello() function.
onsubmit event type
Another most important event type is onsubmit. This event occurs when you try to submit a form. So you can put your form validation against this event type. The Form is submitted by clicking on Submit button, the message box appears.
The Form is submitted by clicking on Submit button, the message box appears.
Example
<html> <head> </head> <body> <script language = "VBScript"> Function fnSubmit() Msgbox("Hello Tutorialspoint.Com") End Function </script> <form action = "/cgi-bin/test.cgi" method = "post" name = "form1" onSubmit = "fnSubmit()"> <input name = "txt1" type = "text"><br> <input name = "btnButton1" type = "submit" value="Submit"> </form> </body> </html>
onmouseover and onmouseout
These two event types will help you to create nice effects with images or even with text as well. The onmouseover event occurs when you bring your mouse over any element and the onmouseout occurs when you take your mouse out from that element.
Example
<html> <head> </head> <body> <script language = "VBScript"> Function AlertMsg Msgbox("ALERT !") End Function Function onmourse_over() Msgbox("Onmouse Over") End Function Sub txt2_OnMouseOut() Msgbox("Onmouse Out !!!") End Sub Sub btnButton_OnMouseOut() Msgbox("onmouse out on Button !") End Sub </script> <form action = "page.cgi" method = "post" name = "form1"> <input name = "txt1" type = "text" OnMouseOut = "AlertMsg()"><br> <input name = "txt2" type = "text" OnMouseOver = "onmourse_over()"> <br><input name = "btnButton" type = "button" value = "Submit"> </form> </body> </html>
It will produce a result when you hover the mouse over the text box and also when you move the focus away from the text box and the button.
HTML 4 Standard Events
The standard HTML 4 events are listed here for your reference. Here, script indicates a VBScript function to be executed against that event.
Event | Value | Description |
---|---|---|
onchange | script | Script runs when the element changes |
onsubmit | script | Script runs when the form is submitted |
onreset | script | Script runs when the form is reset |
onblur | script | Script runs when the element loses focus |
onfocus | script | Script runs when the element gets focus |
onkeydown | script | Script runs when key is pressed |
onkeypress | script | Script runs when key is pressed and released |
onkeyup | script | Script runs when key is released |
onclick | script | Script runs when a mouse click |
ondblclick | script | Script runs when a mouse double-click |
onmousedown | script | Script runs when mouse button is pressed |
onmousemove | script | Script runs when mouse pointer moves |
onmouseout | script | Script runs when mouse pointer moves out of an element |
onmouseover | script | Script runs when mouse pointer moves over an element |
onmouseup | script | Script runs when mouse button is released |
VBScript and Cookies
What are Cookies?
Web Browsers and Servers use HTTP protocol to communicate and HTTP is a stateless protocol. But for a commercial website, it is required to maintain session information among different pages. For example, one user registration ends after completing many pages. But how to maintain user's session information across all the web pages. In many situations, using cookies is the most efficient method of remembering and tracking preferences, purchases, commissions and other information required for better visitor experience or site statistics.
How It Works?
Your server sends some data to the visitor's browser in the form of a cookie. The browser may accept the cookie. If it does, it is stored as a plain text record on the visitor's hard drive. Now, when the visitor arrives at another page on your site, the browser sends the same cookie to the server for retrieval. Once retrieved, your server knows/remembers what was stored earlier. Cookies are a plain text data record of 5 variable-length fields −
Expires − The date the cookie will expire. If this is blank, the cookie will expire when the visitor quits the browser.
Domain − The domain name of your site.
Path − The path to the directory or web page that set the cookie. This may be blank if you want to retrieve the cookie from any directory or page.
Secure − If this field contains the word "secure", then the cookie may only be retrieved with a secure server. If this field is blank, no such restriction exists.
Name=Value − Cookies are set and retrieved in the form of key and value pairs.
Cookies were originally designed for CGI programming and cookies' data is automatically transmitted between the web browser and web server, so CGI scripts on the server can read and write cookie values that are stored on the client.
VBScript can also manipulate cookies using the cookie property of the Document object. VBScript can read, create, modify and delete the cookie or cookies that apply to the current web page.
Storing Cookies
The simplest way to create a cookie is to assign a string value to the document.cookie object, which looks like this −
Syntax
document.cookie = "key1 = value1;key2 = value2;expires = date"
Here expires attribute is optional. If you provide this attribute with a valid date or time, then cookie will expire at the given date or time and after that cookies' value will not be accessible.
Example
Following is the example to set a customer name in input cookie.
<html> <head> <script type = "text/vbscript"> Function WriteCookie If document.myform.customer.value = "" Then msgbox "Enter some value!" Else cookievalue = (document.myform.customer.value) document.cookie = "name = " + cookievalue msgbox "Setting Cookies : " & "name = " & cookievalue End If End Function </script> </head> <body> <form name = "myform" action = ""> Enter name: <input type = "text" name = "customer"/> <input type = "button" value = "Set Cookie" onclick = "WriteCookie()"/> </form> </body> </html>
It will produce the following result. Now enter something in the textbox and press the button "Set Cookie" to set the cookies.
Now, your system has a cookie called name. You can set multiple cookies using multiple key = value pairs separated by comma. You will learn how to read this cookie in next section.
Reading Cookies
Reading a cookie is just as simple as writing one, because the value of the document.cookie object is the cookie. So, you can use this string whenever you want to access the cookie. The document.cookie string will keep a list of name = value pairs separated by semicolons where name is the name of a cookie and value is its string value. You can use strings' split() function to break the string into key and values as follows −
Example
Following is the example to get the cookies set in the previous section −
<html> <head> <script type = "text/vbscript"> Function ReadCookie allcookies = document.cookie msgbox "All Cookies : " + allcookies cookiearray = split(allcookies,";") For i = 0 to ubound(cookiearray) Name = Split(cookiearray(i),"=") Msgbox "Key is : " + Name(0) + " and Value is : " + Name(1) Next End Function </script> </head> <body> <form name = "myform" action = ""> <input type = "button" value = "Get Cookie" onclick = "ReadCookie()"/> </form> </body> </html>
Note − Here, UBound is a method of Array class, which returns the length of an array. We will discuss Arrays in a separate chapter; until that time, please try to digest it.
It will produce the following result. Now, press the button "Get Cookie" to see the cookies, which you have set in previous section.
Note − There may be some other cookies already set on your machine. So, above code will show you all the cookies set at your machine.
Setting the Cookies Expiration Date
You can extend the life of a cookie beyond the current browser session by setting an expiration date and saving the expiration date within the cookie. This can be done by setting the expires attribute to a date and time.
Example
The following example illustrates how to set cookie expiration date after 1 Month −
<html> <head> <script type = "text/vbscript"> Function WriteCookie() x = now() y = dateadd("m",1,now()) ' Making it to expire next cookievalue = document.myform.customer.value document.cookie = "name = " & cookievalue document.cookie = "expires = " & y msgbox("Setting Cookies : " & "name=" & cookievalue ) End Function </script> </head> <body> <form name = "myform" action = ""> Enter name: <input type = "text" name = "customer"/> <input type = "button" value = "Set Cookie" onclick = "WriteCookie()"/> </form> </body> </html>
Deleting a Cookie
Sometimes, you will want to delete a cookie so that subsequent attempts to read the cookie return nothing. To do this, you just need to set the expiration date to a time in the past.
Example
The following example illustrates how to delete a cookie by setting its expiration date 1 Month in the past −
<html> <head> <script type = "text/vbscript"> Function WriteCookie() x = now() x = now() a = Month(x)-1 b = day(x) c = year(x) d = DateSerial(c,a,b) e = hour(x) msgbox e f = minute(x) msgbox f d = cdate(d & " " & e & ":" & f) msgbox d cookievalue = document.myform.customer.value document.cookie = "name = " & cookievalue document.cookie = "expires = " & d msgbox("Setting Cookies : " & "name=" & cookievalue ) End Function </script> </head> <body> <form name = "myform" action = ""> Enter name: <input type = "text" name = "customer"/> <input type = "button" value = "Set Cookie" onclick = "WriteCookie()"/> </form> </body> </html>
VBScript - Numbers
Number functions help the developers to handle numbers in an efficient way and also helps them to convert their subtypes. It also helps them to make use of the inbuilt mathematical functions associated with VBScript.
Number Conversion Functions
Number functions help us to convert a given number from one data subtype to another data subtype.
Sr.No | Function & Description |
---|---|
1 | CDbl A Function, which converts a given number of any variant subtype to double |
2 | CInt A Function, which converts a given number of any variant subtype to Integer |
3 | CLng A Function, which converts a given number of any variant subtype to Long |
4 | CSng A Function, which converts a given number of any variant subtype to Single |
5 | Hex A Function, which converts a given number of any variant subtype to Hexadecimal |
Number Formatting Functions
The Number formatting functions help the developers to express the given number in a format that they wish to.
Sr.No | Function & Description |
---|---|
1 | FormatNumber A Function, which would return an expression formatted as a number |
2 | FormatPercent A Function, which would return an expression formatted as a percentage |
Mathematical Functions
Mathematical Functions help us to evaluate the mathematical and trigonometrical functions of a given input number.
Sr.No | Function & Description |
---|---|
1 | Int A Function, which returns the integer part of the given number |
2 | Fix A Function, which returns the integer part of the given number |
3 | Log A Function, which returns the natural logarithm of the given number. Negative numbers disallowed |
4 | Oct A Function, which returns the Octal value of the given number. |
5 | Hex A Function, which returns the Hexadecimal value of the given number |
6 | Rnd A Function, which returns a random number between 0 and 1 |
7 | Sgn A Function, which returns a number corresponding to the sign of the specified number |
8 | Sqr A Function, which returns the square root of the given number. Negative numbers disallowed |
9 | Abs A Function, which returns the absolute value of the given number |
10 | Exp A Function, which returns the value of e raised to the specified number |
11 | Sin A Function, which returns sine value of the given number |
12 | Cos A Function, which returns cosine value of the given number |
13 | Tan A Function, which returns tan value of the given number |
VBScript - Strings
Strings are a sequence of characters, which can consist of alphabets or numbers or special characters or all of them. A variable is said to be a string if it is enclosed within double quotes " ".
Syntax
variablename = "string"
Examples
str1 = "string" ' Only Alphabets str2 = "132.45" ' Only Numbers str3 = "!@#$;*" ' Only Special Characters Str4 = "Asc23@#" ' Has all the above
String Functions
There are predefined VBScript String functions, which help the developers to work with the strings very effectively. Below are String methods that are supported in VBScript. Please click on each one of the methods to know in detail.
Function Name | Description |
---|---|
InStr | Returns the first occurrence of the specified substring. Search happens from left to right. |
InstrRev | Returns the first occurrence of the specified substring. Search happens from Right to Left. |
Lcase | Returns the lower case of the specified string. |
Ucase | Returns the Upper case of the specified string. |
Left | Returns a specific number of characters from the left side of the string. |
Right | Returns a specific number of characters from the Right side of the string. |
Mid | Returns a specific number of characters from a string based on the specified parameters. |
Ltrim | Returns a string after removing the spaces on the left side of the specified string. |
Rtrim | Returns a string after removing the spaces on the right side of the specified string. |
Trim | Returns a string value after removing both leading and trailing blank spaces. |
Len | Returns the length of the given string. |
Replace | Returns a string after replacing a string with another string. |
Space | Fills a string with the specified number of spaces. |
StrComp | Returns an integer value after comparing the two specified strings. |
String | Returns a String with a specified character the specified number of times. |
StrReverse | Returns a String after reversing the sequece of the characters of the given string. |
VBScript - Arrays
What is an Array?
We know very well that a variable is a container to store a value. Sometimes, developers are in a position to hold more than one value in a single variable at a time. When a series of values is stored in a single variable, then it is known as an array variable.
Array Declaration
Arrays are declared the same way a variable has been declared except that the declaration of an array variable uses parenthesis. In the following example, the size of the array is mentioned in the brackets.
'Method 1 : Using Dim Dim arr1() 'Without Size 'Method 2 : Mentioning the Size Dim arr2(5) 'Declared with size of 5 'Method 3 : using 'Array' Parameter Dim arr3 arr3 = Array("apple","Orange","Grapes")
Although, the Array size is indicated as 5, it can hold 6 values as array index starts from ZERO.
Array Index Cannot be Negative.
VBScript Arrays can store any type of variable in an array. Hence, an array can store an integer, string or characters in a single array variable.
Assigning Values to an Array
The values are assigned to the array by specifying array index value against each one of the values to be assigned. It can be a string.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim arr(5) arr(0) = "1" 'Number as String arr(1) = "VBScript" 'String arr(2) = 100 'Number arr(3) = 2.45 'Decimal Number arr(4) = #10/07/2013# 'Date arr(5) = #12.45 PM# 'Time document.write("Value stored in Array index 0 : " & arr(0) & "<br />") document.write("Value stored in Array index 1 : " & arr(1) & "<br />") document.write("Value stored in Array index 2 : " & arr(2) & "<br />") document.write("Value stored in Array index 3 : " & arr(3) & "<br />") document.write("Value stored in Array index 4 : " & arr(4) & "<br />") document.write("Value stored in Array index 5 : " & arr(5) & "<br />") </script> </body> </html>
When the above code is saved as .HTML and executed in Internet Explorer, it produces the following result −
Value stored in Array index 0 : 1 Value stored in Array index 1 : VBScript Value stored in Array index 2 : 100 Value stored in Array index 3 : 2.45 Value stored in Array index 4 : 7/10/2013 Value stored in Array index 5 : 12:45:00 PM
Multi Dimension Arrays
Arrays are not just limited to single dimension and can have a maximum of 60 dimensions. Two-dimension arrays are the most commonly used ones.
Example
In the following example, a multi-dimension array is declared with 3 rows and 4 columns.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim arr(2,3) ' Which has 3 rows and 4 columns arr(0,0) = "Apple" arr(0,1) = "Orange" arr(0,2) = "Grapes" arr(0,3) = "pineapple" arr(1,0) = "cucumber" arr(1,1) = "beans" arr(1,2) = "carrot" arr(1,3) = "tomato" arr(2,0) = "potato" arr(2,1) = "sandwitch" arr(2,2) = "coffee" arr(2,3) = "nuts" document.write("Value in Array index 0,1 : " & arr(0,1) & "<br />") document.write("Value in Array index 2,2 : " & arr(2,2) & "<br />") </script> </body> </html>
When the above code is saved as .HTML and executed in Internet Explorer, it produces the following result −
Value stored in Array index : 0 , 1 : Orange Value stored in Array index : 2 , 2 : coffee
Redim Statement
ReDim Statement is used to declare dynamic-array variables and allocate or reallocate storage space.
ReDim [Preserve] varname(subscripts) [, varname(subscripts)]
Preserve − An Optional parameter used to preserve the data in an existing array when you change the size of the last dimension.
varname − A Required parameter, which denotes Name of the variable, which should follow the standard variable naming conventions.
subscripts − A Required parameter, which indicates the size of the array.
Example
In the below example, an array has been redefined and then preserved the values when the existing size of the array is changed.
Note − Upon resizing an array smaller than it was originally, the data in the eliminated elements will be lost.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim a() i = 0 redim a(5) a(0) = "XYZ" a(1) = 41.25 a(2) = 22 REDIM PRESERVE a(7) For i = 3 to 7 a(i) = i Next 'to Fetch the output For i = 0 to ubound(a) Msgbox a(i) Next </script> </body> </html>
When we save the above script as HTML and execute it in Internet Explorer, it produces the following result.
XYZ 41.25 22 3 4 5 6 7
Array Methods
There are various inbuilt functions within VBScript which help the developers to handle arrays effectively. All the methods that are used in conjunction with arrays are listed below. Please click on the method name to know in detail.
Function | Description |
---|---|
LBound | A Function, which returns an integer that corresponds to the smallest subscript of the given arrays. |
UBound | A Function, which returns an integer that corresponds to the Largest subscript of the given arrays. |
Split | A Function, which returns an array that contains a specified number of values. Splitted based on a Delimiter. |
Join | A Function, which returns a String that contains a specified number of substrings in an array. This is an exact opposite function of Split Method. |
Filter | A Function, which returns a zero based array that contains a subset of a string array based on a specific filter criteria. |
IsArray | A Function, which returns a boolean value that indicates whether or not the input variable is an array. |
Erase | A Function, which recovers the allocated memory for the array variables. |
VBScript - Date and Time Functions
VBScript Date and Time Functions help the developers to convert date and time from one format to another or to express the date or time value in the format that suits a specific condition.
Date Functions
Function | Description |
---|---|
Date | A Function, which returns the current system date |
CDate | A Function, which converts a given input to Date |
DateAdd | A Function, which returns a date to which a specified time interval has been added |
DateDiff | A Function, which returns the difference between two time period |
DatePart | A Function, which returns a specified part of the given input date value |
DateSerial | A Function, which returns a valid date for the given year,month and date |
FormatDateTime | A Function, which formats the date based on the supplied parameters |
IsDate | A Function, which returns a Boolean Value whether or not the supplied parameter is a date |
Day | A Function, which returns an integer between 1 and 31 that represents the day of the specified Date |
Month | A Function, which returns an integer between 1 and 12 that represents the month of the specified Date |
Year | A Function, which returns an integer that represents the year of the specified Date |
MonthName | A Function, which returns Name of the particular month for the specified date |
WeekDay | A Function, which returns an integer(1 to 7) that represents the day of the week for the specified day. |
WeekDayName | A Function, which returns the weekday name for the specified day. |
Time Functions
Function | Description |
---|---|
Now | A Function, which returns the current system date and Time |
Hour | A Function, which returns and integer between 0 and 23 that represents the Hour part of the the given time |
Minute | A Function, which returns and integer between 0 and 59 that represents the Minutes part of the the given time |
Second | A Function, which returns and integer between 0 and 59 that represents the Seconds part of the the given time |
Time | A Function, which returns the current system time |
Timer | A Function, which returns the number of seconds and milliseconds since 12:00 AM |
TimeSerial | A Function, which returns the time for the specific input of hour,minute and second |
TimeValue | A Function, which converts the input string to a time format |
VBScript - Procedures
What is a Function?
A function is a group of reusable code which can be called anywhere in your program. This eliminates the need of writing same code over and over again. This will enable programmers to divide a big program into a number of small and manageable functions. Apart from inbuilt Functions, VBScript allows us to write user-defined functions as well. This section will explain you how to write your own functions in VBScript.
Function Definition
Before we use a function, we need to define that particular function. The most common way to define a function in VBScript is by using the Function keyword, followed by a unique function name and it may or may not carry a list of parameters and a statement with an End Function keyword, which indicates the end of the function.
The basic syntax is shown below −
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Function Functionname(parameter-list) statement 1 statement 2 statement 3 ....... statement n End Function </script> </body> </html>
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Function sayHello() msgbox("Hello there") End Function </script> </body> </html>
Calling a Function
To invoke a function somewhere later in the script, you would simple need to write the name of that function with the Call keyword.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Function sayHello() msgbox("Hello there") End Function Call sayHello() </script> </body> </html>
Function Parameters
Till now, we have seen function without a parameter, but there is a facility to pass different parameters while calling a function. These passed parameters can be captured inside the function and any manipulation can be done over those parameters. The Functions are called using the Call Keyword.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Function sayHello(name, age) msgbox( name & " is " & age & " years old.") End Function Call sayHello("Tutorials point", 7) </script> </body> </html>
Returning a Value from a Function
A VBScript function can have an optional return statement. This is required if you want to return a value from a function. For example, you can pass two numbers in a function and then you can expect from the function to return their multiplication in your calling program.
NOTE − A function can return multiple values separated by comma as an array assigned to the function name itself.
Example
This function takes two parameters and concatenates them and returns result in the calling program. In VBScript, the values are returned from a function using function name. In case if you want to return two or more values, then the function name is returned with an array of values. In the calling program, the result is stored in the result variable.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Function concatenate(first, last) Dim full full = first & last concatenate = full 'Returning the result to the function name itself End Function </script> </body> </html>
Now, we can call this function as follows −
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Function concatenate(first, last) Dim full full = first & last concatenate = full 'Returning the result to the function name itself End Function ' Here is the usage of returning value from function. dim result result = concatenate("Zara", "Ali") msgbox(result) </script> </body> </html>
Sub Procedures
Sub-Procedures are similar to functions but there are few differences.
Sub-procedures DONOT Return a value while functions may or may not return a value.
Sub-procedures Can be called without call keyword.
Sub-procedures are always enclosed within Sub and End Sub statements.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Sub sayHello() msgbox("Hello there") End Sub </script> </body> </html>
Calling Procedures
To invoke a Procedure somewhere later in the script, you would simply need to write the name of that procedure with or without the Call keyword.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Sub sayHello() msgbox("Hello there") End Sub sayHello() </script> </body> </html>
Advanced Concepts for Functions
There is lot to learn about VBScript functions. We can pass the parameter byvalue or byreference. Please click on each one of them to know more.
VBScript - Dialog Boxes
What is a Dialog Box ?
VBScript allows the developers to interact with the user effectively. It can be a message box to display a message to a user or an input box with which user can enter the values.
VBScript MsgBox Function
The MsgBox function displays a message box and waits for the user to click a button and then an action is performed based on the button clicked by the user.
Syntax
MsgBox(prompt[,buttons][,title][,helpfile,context])
Parameter Description
Prompt − A Required Parameter. A String that is displayed as a message in the dialog box. The maximum length of prompt is approximately 1024 characters. If the message extends to more than a line, then we can separate the lines using a carriage return character (Chr(13)) or a linefeed character (Chr(10)) between each line.
buttons − An Optional Parameter. A Numeric expression that specifies the type of buttons to display, the icon style to use, the identity of the default button, and the modality of the message box. If left blank, the default value for buttons is 0.
Title − An Optional Parameter. A String expression displayed in the title bar of the dialog box. If the title is left blank, the application name is placed in the title bar.
helpfile − An Optional Parameter. A String expression that identifies the Help file to use to provide context-sensitive help for the dialog box.
context − An Optional Parameter. A Numeric expression that identifies the Help context number assigned by the Help author to the appropriate Help topic. If context is provided, helpfile must also be provided.
The Buttons parameter can take any of the following values −
0 vbOKOnly Displays OK button only.
1 vbOKCancel Displays OK and Cancel buttons.
2 vbAbortRetryIgnore Displays Abort, Retry, and Ignore buttons.
3 vbYesNoCancel Displays Yes, No, and Cancel buttons.
4 vbYesNo Displays Yes and No buttons.
5 vbRetryCancel Displays Retry and Cancel buttons.
16 vbCritical Displays Critical Message icon.
32 vbQuestion Displays Warning Query icon.
48 vbExclamation Displays Warning Message icon.
64 vbInformation Displays Information Message icon.
0 vbDefaultButton1 First button is default.
256 vbDefaultButton2 Second button is default.
512 vbDefaultButton3 Third button is default.
768 vbDefaultButton4 Fourth button is default.
0 vbApplicationModal Application modal. The current application will not work until the user responds to the message box.
4096 vbSystemModal System modal. All applications will not work until the user responds to the message box.
The above values are logically divided into four groups: The first group(0 to 5) indicates the buttons to be displayed in the message box. The second group (16, 32, 48, 64) describes the sytle of the icon to be displayed, the third group (0, 256, 512, 768) indicates which button must be the default, and the fourth group (0, 4096) determines the modality of the message box.
Return Values
The MsgBox function can return one of the following values −
1 - vbOK - OK was clicked
2 - vbCancel - Cancel was clicked
3 - vbAbort - Abort was clicked
4 - vbRetry - Retry was clicked
5 - vbIgnore - Ignore was clicked
6 - vbYes - Yes was clicked
7 - vbNo - No was clicked
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> 'Message Box with just prompt message MsgBox("Welcome") 'Message Box with title, yes no and cancel Butttons a = MsgBox("Do you like blue color?",3,"Choose options") ' Assume that you press No Button document.write("The Value of a is " & a) </script> </body> </html>
When the above script is executed, the message box is displayed, and if you press No Button, then the value of a is 7.
The Value of a is 7
VBScript InputBox Function
The InputBox function helps the user to get the values from the user. After entering the values, if the user clicks the OK button or presses ENTER on the keyboard, the InputBox function will return the text in the text box. If the user clicks on the Cancel button, the function will return an empty string ("").
Syntax
InputBox(prompt[,title][,default][,xpos][,ypos][,helpfile,context])
Parameter Description
Prompt − A Required Parameter. A String that is displayed as a message in the dialog box. The maximum length of prompt is approximately 1024 characters. If the message extends to more than a line, then we can separate the lines using a carriage return character (Chr(13)) or a linefeed character (Chr(10)) between each line.
Title − An Optional Parameter. A String expression displayed in the title bar of the dialog box. If the title is left blank, the application name is placed in the title bar.
Default − An Optional Parameter. A default text in the text box that the user would like to be displayed.
XPos − An Optional Parameter. The Position of X axis which represents the prompt distance from left side of the screen horizontally. If left blank, the input box is horizontally centered.
YPos − An Optional Parameter. The Position of Y axis which represents the prompt distance from left side of the screen Vertically. If left blank, the input box is Vertically centered.
helpfile − An Optional Parameter. A String expression that identifies the Help file to use to provide context-sensitive Help for the dialog box.
context − An Optional Parameter. A Numeric expression that identifies the Help context number assigned by the Help author to the appropriate Help topic. If context is provided, helpfile must also be provided.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> ' Input Box with only Prompt InputBox("Enter a number") ' Input Box with a Title a = InputBox("Enter a Number","Enter Value") msgbox a ' Input Box with a Prompt,Title and Default value a = InputBox("Enter a Number","Enter Value",123) msgbox a ' Input Box with a Prompt,Title,Default and XPos a = InputBox("Enter your name","Enter Value",123,700) msgbox a ' Input Box with a Prompt,Title and Default and YPos a = InputBox("Enter your name","Enter Value",123,,500) msgbox a </script> </body> </html>
When the above script is executed, the input box is displayed and displays the entered value by the user.
Object Oriented VBScript
What is an Object
VBScript runtime objects help us to accomplish various tasks. This section will help you understand how to instantiate an object and work with it.
Syntax
In order to work with objects seamlessly, we need to declare the object and instantiate it using Set Keyword.
Dim objectname 'Declare the object name Set objectname = CreateObject(object_type)
Example
In the below example, we are creating an object of type Scripting.Dictionary.
Dim obj Set obj = CreateObject("Scripting.Dictionary")
Destroying the Objects
The significance of destroying the Object is to free the memory and reset the object variable.
Syntax
In order to destroy the objects, we need to use Set Keyword followed by the object name and point it to Nothing.
Set objectname = Nothing 'Destroy the object.
Example
In the below example, we are creating an object of type Scripting.Dictionary.
Dim obj Set obj = CreateObject("Scripting.Dictionary") Set obj = Nothing.
Object Usage
Please click on each one of the given object types to know more.
Object Type | Description |
---|---|
Class | Class is a container, which holds methods and variables associated with it and accessed by creating an object of Type Class. |
Scripting.FileSystemObject | It is the group of objects with which we can work with file system. |
Scripting.Dictionary | A Group of objects, which are used for creating the dictionary objects. |
Debug | A Global Object with which we can send output to the Microsoft script debugger. |
VBScript - Regular Expressions
Regular Expressions is a sequence of characters that forms a pattern, which is mainly used for search and replace. The purpose of creating a pattern is to match specific strings, so that the developer can extract characters based on conditions and replace certain characters.
RegExp Object
RegExp object helps the developers to match the pattern of strings and the properties and methods help us to work with Regular Expressions easily. It is similar to RegExp in JavaScript
Properties
Pattern − The Pattern method represents a string that is used to define the regular expression and it should be set before using the regular expression object.
IgnoreCase − A Boolean property that represents if the regular expression should be tested against all possible matches in a string if true or false. If not specified explicitly, IgnoreCase value is set to False.
Global − A Boolean property that represents if the regular expression should be tested against all possible matches in a string. If not specified explicitly, Global value is set to False.
Methods
Test(search-string) − The Test method takes a string as its argument and returns True if the regular expression can successfully be matched against the string, otherwise False is returned.
Replace(search-string, replace-string) − The Replace method takes 2 parameters. If the search is successful then it replaces that match with the replace-string, and the new string is returned. If there are no matches then the original search-string is returned.
Execute(search-string) − The Execute method works like Replace, except that it returns a Matches collection object, containing a Match object for each successful match. It doesn't modify the original string.
Matches Collection Object
The Matches collection object is returned as a result of the Execute method. This collection object can contain zero or more Match objects and the properties of this object are read-only.
Count − The Count method represents the number of match objects in the collection.
Item − The Item method enables the match objects to be accessed from matches collections object.
Match Object
The Match object is contained within the matches collection object. These objects represent the successful match after the search for a string.
FirstIndex − It represents the position within the original string where the match occurred. This index are zero-based which means that the first position in a string is 0.
Length − A value that represents the total length of the matched string.
Value − A value that represents the matched value or text. It is also the default value when accessing the Match object.
All about Pattern Parameter
The pattern building is similar to PERL. Pattern building is the most important thing while working with Regular Expressions. In this section, we will deal with how to create a pattern based on various factors.
Position Matching
The significance of position matching is to ensure that we place the regular expressions at the correct places.
Symbol | Description |
---|---|
^ | Matches only the beginning of a string. |
$ | Match only the end of a string. |
\b | Matches any word boundary |
\B | Matches any non-word boundary |
Literals Matching
Any form of characters such as alphabet, number or special character or even decimal, hexadecimal can be treated as a Literal. Since few of the characters have already got a special meaning within the context of Regular Expression, we need to escape them using escape sequences.
Symbol | Description |
---|---|
Alphanumeric | Matches alphabetical and numerical characters only. |
\n | Matches a new line. |
\[ | Matches [ literal only |
\] | Matches ] literal only |
\( | Matches ( literal only |
\) | Matches ) literal only |
\t | Matches horizontal tab |
\v | Matches vertical tab |
\| | Matches | literal only |
\{ | Matches { literal only |
\} | Matches } literal only |
\\ | Matches \ literal only |
\? | Matches ? literal only |
\* | Matches * literal only |
\+ | Matches + literal only |
\. | Matches . literal only |
\b | Matches any word boundary |
\B | Matches any non-word boundary |
\f | Matches a form feed |
\r | Matches carriage return |
\xxx | Matches the ASCII character of an octal number xxx. |
\xdd | Matches the ASCII character of an hexadecimal number dd. |
\uxxxx | Matches the ASCII character of an UNICODE literal xxxx. |
Character Classes Matching
The character classes are the Pattern formed by customized grouping and enclosed within [ ] braces. If we are expecting a character class that should not be in the list, then we should ignore that particular character class using the negative symbol, which is a cap ^.
Symbol | Description |
---|---|
[xyz] | Match any of the character class enclosed within the character set. |
[^xyz] | Matches any of the character class that are NOT enclosed within the character set. |
. | Matches any character class except \n |
\w | Match any word character class. Equivalent to [a-zA-Z_0-9] |
\W | Match any non-word character class. Equivalent to [^a-zA-Z_0-9] |
\d | Match any digit class. Equivalent to [0-9]. |
\D | Match any non-digit character class. Equivalent to [^0-9]. |
\s | Match any space character class. Equivalent to [ \t\r\n\v\f] |
\S | Match any space character class. Equivalent to [^\t\r\n\v\f] |
Repetition Matching
Repetition matching allows multiple searches within the regular expression. It also specifies the number of times an element is repeated in a Regular Expression.
Symbol | Description |
---|---|
* | Matches zero or more occurrences of the given regular Expression. Equivalent to {0,}. |
+ | Matches one or more occurrences of the given regular Expression. Equivalent to {1,}. |
? | Matches zero or one occurrences of the given regular Expression. Equivalent to {0,1}. |
{x} | Matches exactly x number of occurrences of the given regular expression. |
{x,} | Match atleast x or more occurrences of the given regular expression. |
{x,y} | Matches x to y number of occurences of the given regular expression. |
Alternation & Grouping
Alternation and grouping helps developers to create more complex Regular Expressions in particularly handling intricate clauses within a Regular Expression which gives a great flexibility and control.
Symbol | Description |
---|---|
0 | Grouping a clause to create a clause. "(xy)?(z)" matches "xyz" or "z". |
| | Alternation combines one regular expression clause and then matches any of the individual clauses. "(ij)|(23)|(pq)" matches "ij" or "23" or "pq". |
Building Regular Expressions
Given below are a few examples that clearly explain how to build a Regular Expression.
Regular Expression | Description |
---|---|
"^\s*.." and "..\s*$" | Represents that there can be any number of leading and trailing space characters in a single line. |
"((\$\s?)|(#\s?))?" | Represents an optional $ or # sign followed by an optional space. |
"((\d+(\.(\d\d)?)?))" | Represents that at least one digit is present followed by an optional decimals and two digits after decimals. |
Example
The below example checks whether or not the user entered an email id whose format should match such that there is an email id followed by '@' and then followed by domain name.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> strid = "welcome.user@tutorialspoint.co.us" Set re = New RegExp With re .Pattern = "^[\w-\.]{1,}\@([\da-zA-Z-]{1,}\.){1,}[\da-zA-Z-]{2,3}$" .IgnoreCase = False .Global = False End With ' Test method returns TRUE if a match is found If re.Test( strid ) Then Document.write(strid & " is a valid e-mail address") Else Document.write(strid & " is NOT a valid e-mail address") End If Set re = Nothing </script> </body> </html>
VBScript - Error Handling
There are three types of errors in programming: (a) Syntax Errors, (b) Runtime Errors, and (c) Logical Errors.
Syntax errors
Syntax errors, also called parsing errors, occur at interpretation time for VBScript. For example, the following line causes a syntax error because it is missing a closing parenthesis −
<script type = "text/vbscript"> dim x,y x = "Tutorialspoint" y = Ucase(x </script>
Runtime errors
Runtime errors, also called exceptions, occur during execution, after interpretation. For example, the following line causes a runtime error because here syntax is correct but at runtime it is trying to call fnmultiply, which is a non-existing function −
<script type = "text/vbscript"> Dim x,y x = 10 y = 20 z = fnadd(x,y) a = fnmultiply(x,y) Function fnadd(x,y) fnadd = x+y End Function </script>
Logical errors
Logic errors can be the most difficult type of errors to track down. These errors are not the result of a syntax or runtime error. Instead, they occur when you make a mistake in the logic that drives your script and you do not get the result you expected. You cannot catch those errors, because it depends on your business requirement what type of logic you want to put in your program. For example, dividing a number by zero or a script that is written which enters into infinite loop.
Err Object
AAssume if we have a runtime error, then the execution stops by displaying the error message. As a developer, if we want to capture the error, then Error Object is used.
Example
In the below example, Err.Number gives the error number and Err.Description gives error description.
<script type = "text/vbscript"> Err.Raise 6 ' Raise an overflow error. MsgBox "Error # " & CStr(Err.Number) & " " & Err.Description Err.Clear ' Clear the error. </script>
VBScript Miscellaneous Statements
VBScript has a few other important statements to help developers develop an efficient script. The following table lists a set of such important statements. In this chapter, we will discuss each of these statements in detail with examples.
Category | Function Name/Statement Name |
---|---|
Options | Option Explicit |
Script Engine ID | ScriptEngine |
variants | IsArray, IsEmpty, IsNull, IsNumeric, IsObject, TypeName |
Expression | Eval,Execute |
Control Statement | With...End With |
Math Function | Randomize |
Option Explicit
Option Explicit forces the developer to declare the variables using Dim statement before they are used in some part of the code.
Syntax
Option Explicit
Example
If we use Option Explicit and if we don't declare the variables then the interpreter will throw and error.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Option Explicit Dim x,y,z,a x = 10 y = 20 z = fnadd(x,y) a = fnmultiply(x,y) Function fnadd(x,y) fnadd = x+y End Function </script> </body> </html>
ScriptEngine
ScriptEngine represents the details of the scripting language in use. It is also used in combination with ScriptEngineMajorVersion, ScriptEngineMinor Version, ScriptEngineBuildVersion which gives the major version of the vbscript engine, minor version the vbscript engine, and the build version of vbscript respectively.
Syntax
ScriptEngine
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim scriptdetails scriptdetails = " Version " & ScriptEngine & " - " 'For getting Major version, use ScriptEngineMajorVersion' scriptdetails = scriptdetails & ScriptEngineMajorVersion & "." 'For getting Minor version, use ScriptEngineMinorVersion' scriptdetails = scriptdetails & ScriptEngineMinorVersion & "." 'For getting Build version, use ScriptEngineBuildVersion' scriptdetails = scriptdetails & ScriptEngineBuildVersion Document.write scriptdetails </script> </body> </html>
Save the file with .html extension upon executing the script in IE , the following result is displayed on the screen.
Version VBScript - 5.8.16996
IsEmpty
The Function IsEmpty is used to check whether or not the expression is empty. It returns a Boolean value. IsEmpty returns True if the variable is uninitialized or explicitly set to Empty. Otherwise the expression returns False.
Syntax
IsEmpty(expression)
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim var, MyCheck MyCheck = IsEmpty(var) Document.write "Line 1 : " & MyCheck & "<br />" var = Null ' Assign Null. MyCheck = IsEmpty(var) Document.write "Line 2 : " & MyCheck & "<br />" var = Empty ' Assign Empty. MyCheck = IsEmpty(var) Document.write "Line 3 : " & MyCheck & "<br />" </script> </body> </html>
Save the file with .html extension upon executing the script in IE, the following result is displayed on the screen.
Line 1 : True Line 2 : False Line 3 : True
IsNull
The Function IsNull is used to check whether or not the expression has a valid data. It returns a Boolean value. IsNull returns True if the variable is Null otherwise the expression returns False.
Syntax
IsNull(expression)
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim var, res res = IsNull(var) document.write "Line 1 : " & res & "<br />" var = Null res = IsNull(var) document.write "Line 2 : " & res & "<br />" var = Empty res = IsNull(var) document.write "Line 3 : " & res & "<br />" </script> </body> </html>
Save the file with .html extension upon executing the script in IE, the following result is displayed on the screen.
Line 1 : False Line 2 : True Line 3 : False
IsObject
The IsObject Function is used to check whether or not the expression has a valid Object. It returns a Boolean value. IsObject returns True if the expression contains an object subtype otherwise the expression returns False.
Syntax
IsObject(expression)
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim fso,b b = 10 set fso = createobject("Scripting.Filesystemobject") x = isobject(fso) Document.write "Line 1 : " & x & "<br />" y = isobject(b) Document.write "Line 2 : " & y & "<br />" </script> </body> </html>
Save the file with .html extension upon executing the script in IE, the following result is displayed on the screen.
Line 1 : True Line 2 : False
IsNumeric
The IsNumeric Function is used to check whether or not the expression has a number subtype. It returns a boolean value. IsObject returns True if the expression contains an number subtype otherwise the expression returns False.
Syntax
IsNumeric(expression)
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim var, chk var = 20 chk = IsNumeric(var) Document.write "Line 1 : " & chk & "<br />" var = "3.1415935745" chk = IsNumeric(var) Document.write "Line 2 : " & chk & "<br / >" var = "20 Chapter 23.123 VBScript" chk = IsNumeric(var) Document.write "Line 3 : " & chk & "<br / >" </script> </body> </html>
Save the file with .html extension upon executing the script in IE , the following result is displayed on the screen.
Line 1 : True Line 2 : True Line 3 : False
TypeName
The TypeName Function is used to return the variant subtype information of the variable.
Syntax
TypeName(varname)
The Typename function can return any of the following values.
Byte − Byte Value
Integer − Integer Value
Long − Long Integer Value
Single − Single-precision floating-point Value
Double − Double-precision floating-point Value
Currency − Currency Value
Decimal − Decimal Value
Date − Date or Time Value
String − Character string Value
Boolean − Boolean Value
Empty − Uninitialized Value
Null − No Valid Data
Object − typename of Object
Nothing − Object variable that doesn't yet refer to an object instance
Error
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim ArrVar(2), vartype NullVar = Null ' Assign Null value. vartype = TypeName(3.1450) Document.write "Line 1 : " & vartype & "<br />" vartype = TypeName(432) Document.write "Line 2 : " & vartype & "<br />" vartype = TypeName("Microsoft") Document.write "Line 3 : " & vartype & "<br />" vartype = TypeName(NullVar) Document.write "Line 4 : " & vartype & "< br />" vartype = TypeName(ArrVar) Document.write "Line 5 : " & vartype & "<br />" </script> </body> </html>
Save the file with .html extension upon executing the script in IE, the following result is displayed on the screen.
Line 1 : Double Line 2 : Integer Line 3 : String Line 4 : Null Line 5 : Variant()
Eval
The Eval Function executes an expression and returns the result either as a string or a number.
Syntax
Eval(expression)
The argument Expression can be a string expression or a number. If you pass to the Eval function a string that doesn't contain a numeric expression or a function name but only a simple text string, a run-time error occurs. For example, Eval("VBScript") results in an error.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Document.write Eval("10 + 10") & "<br />" Document.write Eval("101 = 200") & "<br />" Document.write Eval("5 * 3") & "<br />" </script> </body> </html>
Save the file with .html extension upon executing the script in IE, the following result is displayed on the screen.
20 false 15
Execute
The Execute statement accepts argument that is a string expression containing one or more statements for execution.
Syntax
Execute(expression)
In VBScript, a = b can be interpreted two ways. It can be treated as an assignment statement where the value of x is assigned to y. It can also be interpreted as an expression that tests if a and b have the same value. If they do, result is True; if they are not, result is False. The Execute statement always uses the first interpretation while the Eval statement always uses the second.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim x x = "Global" y = "VBScript" Execute("x = y") msgbox x msgbox y </script> </body> </html>
Save the file with .html extension upon executing the script in IE, the following result is displayed on the screen.
VBScript VBScript
With..End With
The With statement allows us to perform a series of operation on a specified object without explicitly mentioning the object name over again and again.
Syntax
With (objectname) statement 1 statement 2 statement 3 ... ... statement n End With
Example
Upon Executing the following script, Winword gets opened and the specified text is entered.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Msg = "Vbscript" & vbCrLf & "Programming" Set objWord = CreateObject("Word.Application") objWord.Visible = True ' Objects methods are accessed without requaliyfying the objects again.' With objWord .Documents.Add .Selection.TypeText Msg .Selection.WholeStory End With </script> </body> </html>
Randomize
The Randomize statement initializes the random number generator which is helpful for the developers to generate a random number.
Syntax
Randomize [number]
Example
Upon Executing the following script, Winword gets opened and the specified text is entered.
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim MyValue Randomize MyValue = Int((100 * Rnd) + 1) ' Generate random value between 1 and 100. MsgBox MyValue </script> </body> </html>
Save the above script as HTML and upon executing the script in IE, the following output is shown.
42