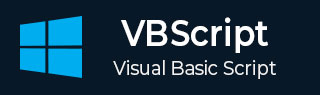
- VBScript Tutorial
- VBScript - Home
- VBScript - Overview
- VBScript - Syntax
- VBScript - Enabling
- VBScript - Placement
- VBScript - Variables
- VBScript - Constants
- VBScript - Operators
- VBScript - Decisions
- VBScript - Loops
- VBScript - Events
- VBScript - Cookies
- VBScript - Numbers
- VBScript - Strings
- VBScript - Arrays
- VBScript - Date
- VBScript Advanced
- VBScript - Procedures
- VBScript - Dialog Boxes
- VBScript - Object Oriented
- VBScript - Reg Expressions
- VBScript - Error Handling
- VBScript - Misc Statements
- VBScript Useful Resources
- VBScript - Questions and Answers
- VBScript - Quick Guide
- VBScript - Useful Resources
- VBScript - Discussion
VBScript Dictionary Objects
A Dictionary object can be compared to a PERL associative array. Any Values can be stored in the array and each item is associated with a unique key. The key is used to retrieve an individual element and it is usually an integer or a string, but can be anything except an array.
Syntax
VBScript classes are enclosed within Class .... End Class.
Dim variablename Set variablename = CreateObject("Scripting.Dictionary") variablename.Add (key, item)
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim obj_datadict ' Create a variable. Set obj_datadict = CreateObject("Scripting.Dictionary") obj_datadict.Add "a", "Apple" ' Add some keys and items. obj_datadict.Add "b", "Bluetooth" obj_datadict.Add "c", "Clear" </script> </body> </html>
There are various methods associated with DataDictionary Objects which enable the developers to work with dictionary objects seamlessly.
Exists Method
Exist Method helps the user to check whether or not the Key Value pair exists.
object.Exists(key)
Parameter Description
Object, a Mandatory Parameter. This represents the name of the Dictionary Object.
Key, a Mandatory Parameter. This represents the value of the Dictionary Object.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim d, msg ' Create some variables. Set d = CreateObject("Scripting.Dictionary") d.Add "a", "Apple" ' Add some keys and items. d.Add "b", "BlueTooth" d.Add "c", "C++" If d.Exists("c") Then msgbox "Specified key exists." Else msgbox "Specified key doesn't exist." End If </script> </body> </html>
Save the file as .HTML, and upon executing the above script in IE, it displays the following message in a message box.
Specified key exists.
Items Method
Items Method helps us to get the values stored in the key value pair of the data dictionary object.
object.Items( )
Parameter Description
Object, a Mandatory Parameter. This represents the name of the Dictionary Object.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim obj_datadict ' Create a variable. Set obj_datadict = CreateObject("Scripting.Dictionary") obj_datadict.Add "a", "Apple" ' Add some keys and items. obj_datadict.Add "b", "Bluetooth" obj_datadict.Add "c", "C++" a = obj_datadict.items msgbox a(0) msgbox a(2) </script> </body> </html>
Save the file as .HTML, and upon executing the above script in IE, it displays the following message in a message box.
Apple C++
Keys Method
object.Keys( )
Parameter Description
Object, a Mandatory Parameter. This represents the name of the Dictionary Object.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim obj_datadict ' Create a variable. Set obj_datadict = CreateObject("Scripting.Dictionary") obj_datadict.Add "a", "Apple" ' Add some keys and items. obj_datadict.Add "b", "Bluetooth" obj_datadict.Add "c", "C++" a = obj_datadict.Keys msgbox a(0) msgbox a(2) </script> </body> </html>
Save the file as .HTML, and upon executing the above script in IE, it displays the following message in a message box.
a c
Remove Method
object.Remove(key)
Parameter Description
Object, a Mandatory Parameter. This represents the name of the Dictionary Object.
Key, a Mandatory Parameter. This represents the key value pair that needs to be removed from the Dictionary Object.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim obj_datadict ' Create a variable. Set obj_datadict = CreateObject("Scripting.Dictionary") obj_datadict.Add "a", "Apple" ' Add some keys and items. obj_datadict.Add "b", "Bluetooth" obj_datadict.Add "c", "C++" a = obj_datadict.Keys msgbox a(0) msgbox a(2) obj_datadict.remove("b") 'The key value pair of "b" is removed' </script> </body> </html>
Save the file as .HTML, and upon executing the above script in IE, it displays the following message in a message box.
a c
Remove All Method
object.RemoveAll()
Parameter Description
Object, a Mandatory Parameter. This represents the name of the Dictionary Object.
Example
<!DOCTYPE html> <html> <body> <script language = "vbscript" type = "text/vbscript"> Dim obj_datadict ' Create a variable. Set obj_datadict = CreateObject("Scripting.Dictionary") obj_datadict.Add "a", "Apple" ' Add some keys and items. obj_datadict.Add "b", "Bluetooth" obj_datadict.Add "c", "C++" a = obj_datadict.Keys msgbox a(0) msgbox a(2) obj_datadict.removeall </script> </body> </html>