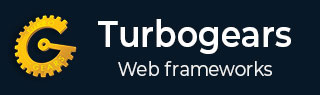
- TurboGears Tutorial
- TurboGears - Home
- TurboGears - Overview
- TurboGears - Environment
- TurboGears - First Program
- TurboGears - Dependencies
- TurboGears - Serving Templates
- TurboGears - HTTP Methods
- Genshi Template Language
- TurboGears - Includes
- TurboGears - JSON Rendering
- TurboGears - URL Hierarchy
- TurboGears - Toscawidgets Forms
- TurboGears - Validation
- TurboGears - Flash Messages
- TurboGears - Cookies and Sessions
- TurboGears - Caching
- TurboGears - Sqlalchemy
- TurboGears - Creating Models
- TurboGears - Crud Operations
- TurboGears - DataGrid
- TurboGears - Pagination
- TurboGears - Admin Access
- Authorization & Authentication
- TurboGears - Using MongoDB
- TurboGears - Scaffolding
- TurboGears - Hooks
- TurboGears - Writing Extensions
- TurboGears - Pluggable Applications
- TurboGears - Restful Applications
- TurboGears - Deployment
- TurboGears Useful Resources
- TurboGears - Quick Guide
- TurboGears - Useful Resources
- TurboGears - Discussion
TurboGears – Creating Models
Let us add a student model which will set up a student table in our sqlite database.
Hello\hello\model\student.py
from sqlalchemy import * from sqlalchemy.orm import mapper, relation, relation, backref from sqlalchemy import Table, ForeignKey, Column from sqlalchemy.types import Integer, Unicode, DateTime from hello.model import DeclarativeBase, metadata, DBSession from datetime import datetime class student(DeclarativeBase): __tablename__ = 'student' uid = Column(Integer, primary_key = True) name = Column(Unicode(20), nullable = False, default = '') city = Column(Unicode(20), nullable = False, default = '') address = Column(Unicode(100), nullable = False, default = '') pincode = Column(Unicode(10), nullable = False, default = '')
Now add this model in init_model() function inside __init__.py. This function already contains the auth model in it. Add our student model below it.
# Import your model modules here. from hello.model.auth import User, Group, Permission from hello.model.student import student
If you want the table to be initialized with some data at the time of setting up the models, add it in bootstrap.py in websetup package. Add the following statements in the bootstrap() function.
s1 = model.student() s1.name = 'M.V.Lathkar' s1.city = 'Nanded' s1.address = 'Shivaji Nagar' s1.pincode = '431602' model.DBSession.add(s1) model.DBSession.flush() transaction.commit()
The Models are initialized by running setup-app command of gearbox −
gearbox setup-app
Session object of SQLAlchemy manages all persistence operations of ORM object.
Advertisements