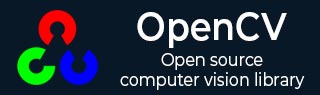
- OpenCV Tutorial
- OpenCV - Home
- OpenCV - Overview
- OpenCV - Environment
- OpenCV - Storing Images
- OpenCV - Reading Images
- OpenCV - Writing an Image
- OpenCV - GUI
- Types of Images
- OpenCV - The IMREAD_XXX Flag
- Reading an Image as Grayscale
- OpenCV - Reading Image as BGR
- Image Conversion
- Colored Images to GrayScale
- OpenCV - Colored Image to Binary
- OpenCV - Grayscale to Binary
- Drawing Functions
- OpenCV - Drawing a Circle
- OpenCV - Drawing a Line
- OpenCV - Drawing a Rectangle
- OpenCV - Drawing an Ellipse
- OpenCV - Drawing Polylines
- OpenCV - Drawing Convex Polylines
- OpenCV - Drawing Arrowed Lines
- OpenCV - Adding Text
- Filtering
- OpenCV - Bilateral Filter
- OpenCV - Box Filter
- OpenCV - SQRBox Filter
- OpenCV - Filter2D
- OpenCV - Dilation
- OpenCV - Erosion
- OpenCV - Morphological Operations
- OpenCV - Image Pyramids
- Sobel Derivatives
- OpenCV - Sobel Operator
- OpenCV - Scharr Operator
- Transformation Operations
- OpenCV - Laplacian Transformation
- OpenCV - Distance Transformation
- Camera and Face Detection
- OpenCV - Using Camera
- OpenCV - Face Detection in a Picture
- Face Detection using Camera
- Geometric Transformations
- OpenCV - Affine Translation
- OpenCV - Rotation
- OpenCV - Scaling
- OpenCV - Color Maps
- Miscellaneous Chapters
- OpenCV - Canny Edge Detection
- OpenCV - Hough Line Transform
- OpenCV - Histogram Equalization
- OpenCV Useful Resources
- OpenCV - Quick Guide
- OpenCV - Useful Resources
- OpenCV - Discussion
OpenCV - Dilation
Erosion and dilation are the two types of morphological operations. As the name implies, morphological operations are the set of operations that process images according to their shapes.
Based on the given input image a "structural element" is developed. This might be done in any of the two procedures. These are aimed at removing noise and settling down the imperfections, to make the image clear.
Dilation
This procedure follows convolution with some kernel of a specific shape such as a square or a circle. This kernel has an anchor point, which denotes its center.
This kernel is overlapped over the picture to compute maximum pixel value. After calculating, the picture is replaced with anchor at the center. With this procedure, the areas of bright regions grow in size and hence the image size increases.
For example, the size of an object in white shade or bright shade increases, while the size of an object in black shade or dark shade decreases.
You can perform the dilation operation on an image using the dilate() method of the imgproc class. Following is the syntax of this method.
dilate(src, dst, kernel)
This method accepts the following parameters −
src − A Mat object representing the source (input image) for this operation.
dst − A Mat object representing the destination (output image) for this operation.
kernel − A Mat object representing the kernel.
Example
You can prepare the kernel matrix using the getStructuringElement() method. This method accepts an integer representing the morph_rect type and an object of the type Size.
Imgproc.getStructuringElement(int shape, Size ksize);
The following program demonstrates how to perform the dilation operation on a given image.
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.core.Size; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; public class DilateTest { public static void main( String[] args ) { // Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); // Reading the Image from the file and storing it in to a Matrix object String file ="C:/EXAMPLES/OpenCV/sample.jpg"; Mat src = Imgcodecs.imread(file); // Creating an empty matrix to store the result Mat dst = new Mat(); // Preparing the kernel matrix object Mat kernel = Imgproc.getStructuringElement(Imgproc.MORPH_RECT, new Size((2*2) + 1, (2*2)+1)); // Applying dilate on the Image Imgproc.dilate(src, dst, kernel); // Writing the image Imgcodecs.imwrite("E:/OpenCV/chap10/Dilation.jpg", dst); System.out.println("Image Processed"); } }
Input
Assume that following is the input image sample.jpg specified in the above program.
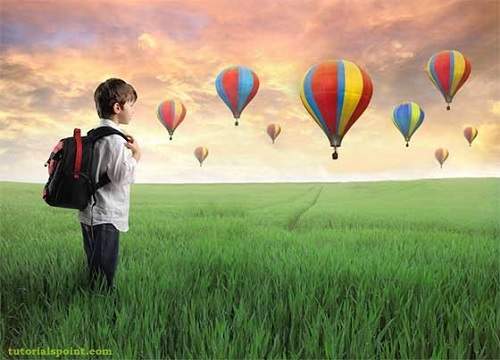
Output
On executing the program, you will get the following output −
Image Processed
If you open the specified path, you can observe the output image as follows −
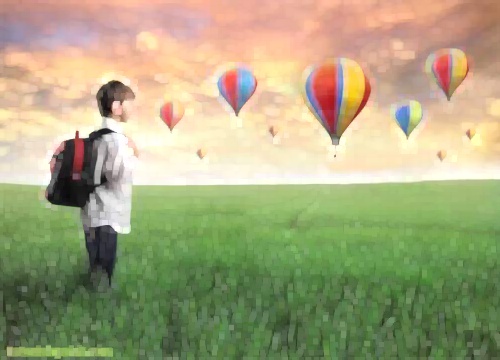