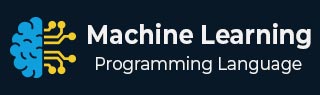
- Machine Learning Basics
- Machine Learning - Home
- Machine Learning - Getting Started
- Machine Learning - Basic Concepts
- Machine Learning - Python Libraries
- Machine Learning - Applications
- Machine Learning - Life Cycle
- Machine Learning - Required Skills
- Machine Learning - Implementation
- Machine Learning - Challenges & Common Issues
- Machine Learning - Limitations
- Machine Learning - Reallife Examples
- Machine Learning - Data Structure
- Machine Learning - Mathematics
- Machine Learning - Artificial Intelligence
- Machine Learning - Neural Networks
- Machine Learning - Deep Learning
- Machine Learning - Getting Datasets
- Machine Learning - Categorical Data
- Machine Learning - Data Loading
- Machine Learning - Data Understanding
- Machine Learning - Data Preparation
- Machine Learning - Models
- Machine Learning - Supervised
- Machine Learning - Unsupervised
- Machine Learning - Semi-supervised
- Machine Learning - Reinforcement
- Machine Learning - Supervised vs. Unsupervised
- Machine Learning Data Visualization
- Machine Learning - Data Visualization
- Machine Learning - Histograms
- Machine Learning - Density Plots
- Machine Learning - Box and Whisker Plots
- Machine Learning - Correlation Matrix Plots
- Machine Learning - Scatter Matrix Plots
- Statistics for Machine Learning
- Machine Learning - Statistics
- Machine Learning - Mean, Median, Mode
- Machine Learning - Standard Deviation
- Machine Learning - Percentiles
- Machine Learning - Data Distribution
- Machine Learning - Skewness and Kurtosis
- Machine Learning - Bias and Variance
- Machine Learning - Hypothesis
- Regression Analysis In ML
- Machine Learning - Regression Analysis
- Machine Learning - Linear Regression
- Machine Learning - Simple Linear Regression
- Machine Learning - Multiple Linear Regression
- Machine Learning - Polynomial Regression
- Classification Algorithms In ML
- Machine Learning - Classification Algorithms
- Machine Learning - Logistic Regression
- Machine Learning - K-Nearest Neighbors (KNN)
- Machine Learning - Naïve Bayes Algorithm
- Machine Learning - Decision Tree Algorithm
- Machine Learning - Support Vector Machine
- Machine Learning - Random Forest
- Machine Learning - Confusion Matrix
- Machine Learning - Stochastic Gradient Descent
- Clustering Algorithms In ML
- Machine Learning - Clustering Algorithms
- Machine Learning - Centroid-Based Clustering
- Machine Learning - K-Means Clustering
- Machine Learning - K-Medoids Clustering
- Machine Learning - Mean-Shift Clustering
- Machine Learning - Hierarchical Clustering
- Machine Learning - Density-Based Clustering
- Machine Learning - DBSCAN Clustering
- Machine Learning - OPTICS Clustering
- Machine Learning - HDBSCAN Clustering
- Machine Learning - BIRCH Clustering
- Machine Learning - Affinity Propagation
- Machine Learning - Distribution-Based Clustering
- Machine Learning - Agglomerative Clustering
- Dimensionality Reduction In ML
- Machine Learning - Dimensionality Reduction
- Machine Learning - Feature Selection
- Machine Learning - Feature Extraction
- Machine Learning - Backward Elimination
- Machine Learning - Forward Feature Construction
- Machine Learning - High Correlation Filter
- Machine Learning - Low Variance Filter
- Machine Learning - Missing Values Ratio
- Machine Learning - Principal Component Analysis
- Machine Learning Miscellaneous
- Machine Learning - Performance Metrics
- Machine Learning - Automatic Workflows
- Machine Learning - Boost Model Performance
- Machine Learning - Gradient Boosting
- Machine Learning - Bootstrap Aggregation (Bagging)
- Machine Learning - Cross Validation
- Machine Learning - AUC-ROC Curve
- Machine Learning - Grid Search
- Machine Learning - Data Scaling
- Machine Learning - Train and Test
- Machine Learning - Association Rules
- Machine Learning - Apriori Algorithm
- Machine Learning - Gaussian Discriminant Analysis
- Machine Learning - Cost Function
- Machine Learning - Bayes Theorem
- Machine Learning - Precision and Recall
- Machine Learning - Adversarial
- Machine Learning - Stacking
- Machine Learning - Epoch
- Machine Learning - Perceptron
- Machine Learning - Regularization
- Machine Learning - Overfitting
- Machine Learning - P-value
- Machine Learning - Entropy
- Machine Learning - MLOps
- Machine Learning - Data Leakage
- Machine Learning - Resources
- Machine Learning - Quick Guide
- Machine Learning - Useful Resources
- Machine Learning - Discussion
Machine Learning - Regularization
In machine learning, regularization is a technique used to prevent overfitting, which occurs when a model is too complex and fits the training data too well, but fails to generalize to new, unseen data. Regularization introduces a penalty term to the cost function, which encourages the model to have smaller weights and a simpler structure, thereby reducing overfitting.
There are several types of regularization techniques commonly used in machine learning, including L1 and L2 regularization, dropout regularization, and early stopping. In this article, we will focus on L1 and L2 regularization, which are the most commonly used techniques.
L1 Regularization
L1 regularization, also known as Lasso regularization, is a technique that adds a penalty term to the cost function, equal to the absolute value of the sum of the weights. The formula for the L1 regularization penalty is −
$$\lambda \times \Sigma \left|w_{i} \right|$$
where λ is a hyperparameter that controls the strength of the regularization, and 𝑤𝑖 is the i-th weight in the model.
The effect of the L1 regularization penalty is to encourage the model to have sparse weights, that is, to eliminate the weights that have little or no impact on the output. This has the effect of simplifying the model and reducing overfitting.
Example
To implement L1 regularization in Python, we can use the Lasso class from the scikit-learn library. Here is an example of how to use L1 regularization for linear regression −
from sklearn.linear_model import Lasso from sklearn.datasets import load_boston from sklearn.model_selection import train_test_split from sklearn.metrics import mean_squared_error # Load the Boston Housing dataset boston = load_boston() # Split the data into training and test sets X_train, X_test, y_train, y_test = train_test_split(boston.data, boston.target, test_size=0.2, random_state=42) # Create a Lasso model with L1 regularization lasso = Lasso(alpha=0.1) # Train the model on the training data lasso.fit(X_train, y_train) # Make predictions on the test data y_pred = lasso.predict(X_test) # Calculate the mean squared error of the predictions mse = mean_squared_error(y_test, y_pred) print("Mean squared error:", mse)
In this example, we load the Boston Housing dataset, split it into training and test sets, and create a Lasso model with L1 regularization using an alpha value of 0.1. We then train the model on the training data and make predictions on the test data. Finally, we calculate the mean squared error of the predictions.
Output
When you execute this code, it will produce the following output −
Mean squared error: 25.155593753934173
L2 Regularization
L2 regularization, also known as Ridge regularization, is a technique that adds a penalty term to the cost function, equal to the square of the sum of the weights. The formula for the L2 regularization penalty is −
$$\lambda \times \Sigma\left (w_{i} \right )^{2}$$
where λ is a hyperparameter that controls the strength of the regularization, and wi is the ith weight in the model.
The effect of the L2 regularization penalty is to encourage the model to have small weights, that is, to reduce the magnitude of all the weights in the model. This has the effect of smoothing the model and reducing overfitting.
Example
To implement L2 regularization in Python, we can use the Ridge class from the scikit-learn library. Here is an example of how to use L2 regularization for linear regression −
from sklearn.linear_model import Ridge from sklearn.model_selection import train_test_split from sklearn.metrics import mean_squared_error from sklearn.datasets import load_boston from sklearn.preprocessing import StandardScaler import numpy as np # load the Boston housing dataset boston = load_boston() # create feature and target arrays X = boston.data y = boston.target # standardize the feature data scaler = StandardScaler() X = scaler.fit_transform(X) # split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # define the Ridge regression model with L2 regularization model = Ridge(alpha=0.1) # fit the model on the training data model.fit(X_train, y_train) # make predictions on the testing data y_pred = model.predict(X_test) # calculate the mean squared error mse = mean_squared_error(y_test, y_pred) print("Mean Squared Error: ", mse)
In this example, we first load the Boston housing dataset and split it into training and testing sets. We then standardize the feature data using a StandardScaler.
Next, we define the Ridge regression model and set the alpha parameter to 0.1, which controls the strength of the L2 regularization.
We fit the model on the training data and make predictions on the testing data. Finally, we calculate the mean squared error to evaluate the performance of the model.
Output
When you execute this code, it will produce the following output −
Mean Squared Error: 24.29346250596107