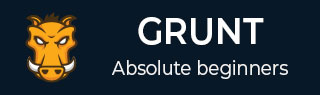
- Grunt Tutorial
- Grunt - Home
- Grunt - Overview
- Grunt - Features
- Grunt - Installing
- Grunt - Getting started
- Grunt - Configuring Tasks
- Grunt - Sample File
- Grunt - Creating Tasks
- Grunt Useful Resources
- Grunt - Quick Guide
- Grunt - Useful Resources
- Grunt - Discussion
Grunt - Sample File
In this chapter, let us create a simple Grunt file using the following plugins −
- grunt-contrib-uglify
- grunt-contrib-concat
- grunt-contrib-jshint
- grunt-contrib-watch
Install all the above plugins and follow the steps given below to create a simple Gruntfile.js −
Step 1 − You need to create a wrapper function, which encapsulates the configurations for your Grunt.
module.exports = function(grunt) {};
Step 2 − Initialize your configuration object as shown below −
grunt.initConfig({});
Step 3 − Next, read the project settings from the package.json file into the pkg property. It enables us to refer to the properties values within yourpackage.json file.
pkg: grunt.file.readJSON('package.json')
Step 4 − Next, you can define configurations for tasks. Let us create our first task concat to concatenate all the files that are present in the src/ folder and store the concatenated .js file under the dist/ folder.
concat: { options: { // define a string to insert between files in the concatenated output separator: ';' }, dist: { // files needs to be concatenated src: ['src/**/*.js'], // location of the concatenated output JS file dest: 'dist/<%= pkg.name %>.js' } }
Step 5 − Now, let us create another task called uglify to minify our JavaScript.
uglify: { options: { // banner will be inserted at the top of the output which displays the date and time banner: '/*! <%= pkg.name %> <%= grunt.template.today() %> */\n' }, dist: { files: { 'dist/<%= pkg.name %>.min.js': ['<%= concat.dist.dest %>'] } } }
The above task creates a file within the dist/ folder which contains the minified .js files. The <%= concat.dist.dest %> will instruct uglify to minify the file that concat task generates.
Step 6 − Let us configure JSHint plugin by creating jshint task.
jshint: { // define the files to lint files: ['Gruntfile.js', 'src/**/*.js'], // configure JSHint options: { // more options here if you want to override JSHint defaults globals: { jQuery: true, } } }
The above jshint task accepts an array of files and then an object of options. The above task will look for any coding violation in Gruntfile.js and src/**/*.js files.
Step 7 − Next, we have the watch task which looks for changes in any of the specified files and runs the tasks you specify.
watch: { files: ['<%= jshint.files %>'], tasks: ['jshint'] }
Step 8 − Next, we have to load Grunt plugins which have all been installed via _npm.
grunt.loadNpmTasks('grunt-contrib-uglify'); grunt.loadNpmTasks('grunt-contrib-jshint'); grunt.loadNpmTasks('grunt-contrib-watch'); grunt.loadNpmTasks('grunt-contrib-concat');
Step 9 − Finally, we have to define the default task.
grunt.registerTask('default', ['jshint', 'concat', 'uglify']);
The default task can be run by just typing the grunt command on command line.
Here is your complete Gruntfile.js −
module.exports = function(grunt) { grunt.initConfig({ pkg: grunt.file.readJSON('package.json'), concat: { options: { separator: ';' }, dist: { src: ['src/**/*.js'], dest: 'dist/<%= pkg.name %>.js' } }, uglify: { options: { banner: '/*! <%= pkg.name %> <%= grunt.template.today() %> */\n' }, dist: { files: { 'dist/<%= pkg.name %>.min.js': ['<%= concat.dist.dest %>'] } } }, jshint: { // define the files to lint files: ['Gruntfile.js', 'src/**/*.js'], // configure JSHint options: { // more options here if you want to override JSHint defaults globals: { jQuery: true, } } }, watch: { files: ['<%= jshint.files %>'], tasks: ['jshint'] } }); grunt.loadNpmTasks('grunt-contrib-uglify'); grunt.loadNpmTasks('grunt-contrib-jshint'); grunt.loadNpmTasks('grunt-contrib-watch'); grunt.loadNpmTasks('grunt-contrib-concat'); grunt.registerTask('default', ['jshint', 'concat', 'uglify']); };