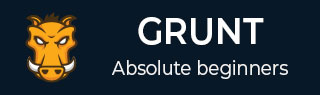
- Grunt Tutorial
- Grunt - Home
- Grunt - Overview
- Grunt - Features
- Grunt - Installing
- Grunt - Getting started
- Grunt - Configuring Tasks
- Grunt - Sample File
- Grunt - Creating Tasks
- Grunt Useful Resources
- Grunt - Quick Guide
- Grunt - Useful Resources
- Grunt - Discussion
Grunt - Configuring Tasks
You can define project-specific configuration data for Grunt in the Gruntfile.js file.
Grunt Configuration
The task configuration data can be initialized in the Gruntfile by using the grunt.initConfig() method. Inside the grunt.initConfig() function, take the configuration information from package.json file. The configuration will contain a task named properties and any arbitrary data.
grunt.initConfig({ jshint: { // configuration for jshint task }, cssmin: { // configuration for cssmin task }, // Arbitrary non-task-specific properties my_files: ['dir1/*.js', 'dir2/*.js'], });
Task Configuration and Targets
When you are running a task, Grunt looks for the configuration under task-named property. We will define tasks with multiple configurations and target options as shown below −
grunt.initConfig({ jshint: { myfile1: { // configuration for "myfile1" target options }, myfile2: { // configuration for "myfile2" target options }, }, cssmin: { myfile3: { // configuration for "myfile3" target options }, }, });
Here, jshint task has myfile1 and myfile2 targets and cssmin task has myfile3 target. When you are running the grunt jshint, it will iterate over both task and target to process the specified target's configuration.
Options
Define the options property inside task configuration which overrides the task defaults. Each target includes options property that overrides the task-level options. It will be having the following format −
grunt.initConfig({ jshint: { options: { // task-level options that overrides task defaults }, myfile: { options: { // "myfile" target options overrides task defaults }, }, myfile1: { // there is no option, target will use task-level options }, }, });
Files
Grunt provides some ideas for specifying on which files the task should operate and uses different ways to specify the src-dest file mappings. Following are some of the additional properties which are supported by the src and dest mappings −
filter − It is a function that specifies matched src file path and returns true or false values.
nonull − It defines the non matching patterns when it is set to true.
dot − It matches the file names starting with a period or otherwise.
matchBase − It matches the patterns which contains slashes with the basename of the path.
expand − It processes the src-dest file mapping.
Compact Format
It specifies the src-dest file mapping per target that can be used for read-only tasks and require only src property and no dest property.
grunt.initConfig({ jshint: { myfile1: { src: ['src/file1.js','src/file2.js'] }, }, cssmin: { myfile2: { src: ['src/file3.js','src/file4.js'], dest: 'dest/destfile.js', }, }, });
Files Object Format
It specifies the src-dest file mapping per target in which the property name is dest file and its value is src file.
grunt.initConfig({ jshint: { myfile1: { files: { 'dest/destfile.js':['src/file1.js','src/file2.js'], 'dest/destfile1.js':['src/file3.js','src/file4.js'], }, }, myfile2: { files: { 'dest/destfile2.js':['src/file22.js','src/file23.js'], 'dest/destfile21.js':['src/file24.js','src/file25.js'], }, }, }, });
Files Array Format
It specifies the src-dest file mapping per target by using additional properties per mapping.
grunt.initConfig({ jshint: { myfile1: { files: [ {src:['src/file1.js','src/file2.js'],dest:'dest/file3.js'}, {src:['src/file4.js','src/file4.js'],dest:'dest/file5.js'}, ], }, myfile2: { files: [ {src:['src/file6.js','src/file7.js'],dest:'dest/file8/', nonull:true}, {src:['src/file9.js','src/file10.js'],dest:'dest/file11/', filter:'isFalse'}, ], }, }, });
Older Formats
The dest-as-target file format was there before the existence of the multitasks where the destination file path is name of the target. The following format is deprecated and it is not to be used in the code.
grunt.initConfig({ jshint: { 'dest/destfile2.js':['src/file3.js','src/file4.js'], 'dest/destfile5.js':['src/file6.js','src/file7.js'], }, });
Custom Filter Function
You can help the target files with a great level of detail by using the filter property. The following format cleans files only if it matches an actual file.
grunt.initConfig({ clean: { myfile:{ src: ['temp/**/*'], filter: 'isFile', }, }, });
Globbing Patterns
Globbing means expanding the file name. Grunt supports globbing by using the built-in node-glob and minimatch libraries. The globbing pattern includes the following points −
- * matches any number of characters, but not /.
- ? matches a single character, but not /.
- ** matches a number of characters including /.
- {} specifies comma separated list of "or" expressions.
- ! will negate the pattern match at the beginning.
For Example −
{src: 'myfile/file1.js', dest: ...} // it specifies the single file {src: 'myfile/*.js', dest: ...} //it matches all the files ending wth .js {src: 'myfile/{file1,file2}*.js', dest: ...} //defines the single node glob pattern {src: ['myfile/*.js', '!myfile/file1.js'], dest: ...} // all files will display in alpha // order except for file1.js
Building the Files Object Dynamically
When you are working with individual files, you can use additional properties to build a files list dynamically. When you set the expand property to true, it will enable some of the following properties −
cwd matches all src to this path.
src matches the patterns to match, relative to the cwd.
dest property specifies destination path prefix.
ext will replace an existing extension with a value generated in dest paths.
extDot indicates where the period indicating the extension is located. It uses either the first period or the last period; by default, it is set to the first period
flatten removes all path parts from the dest paths.
rename specifies a string containing the new destination and filename.
The Rename Property
It is a unique JavaScript function which returns a string and you cannot use a string value for rename. In the following example, the copy task will create a backup of README.md.
grunt.initConfig({ copy: { backup: { files: [{ expand: true, src: ['docs/README.md'], // creating a backup of README.md rename: function () { // specifies the rename function return 'docs/BACKUP.txt'; // returns a string with the complete destination } }] } } });
Templates
You can specify the templates using <% %> delimiters. They will be expanded automatically when they are read from the config. It includes two types of properties −
<%= prop.subprop %> property is used to expand the value ofprop.subprop in the config which can reference the string values, arrays and other objects.
<% %> property executes the inline JavaScript code which is used for control flow or looping.
For Example −
grunt.initConfig({ concat: { myfile: { options: { banner: '/* <%= val %> */\n', }, src: ['<%= myval %>', 'file3/*.js'], dest: 'build/<%= file3 %>.js', }, }, // properties used in task configuration templates file1: 'c', file2: 'b<%= file1 %>d', file3: 'a<%= file2 %>e', myval: ['file1/*.js', 'file2/*.js'], });
Importing External Data
You can import external data from package.json file. The grunt-contrib-uglify plugin can be used to minify the source file and it creates a banner comment using metadata. You can use grunt.file.readJSON and grunt.file.readYAML for importing JSON and YAML data.
For Example −
grunt.initConfig({ pkg: grunt.file.readJSON('package.json'), uglify: { options: { banner: '/*! <%= pkg.name %> <%= grunt.template.today("yyyy-mm-dd") %> */\n' }, dist: { src: 'src/<%= pkg.name %>.js', dest: 'dist/<%= pkg.name %>.min.js' } } });