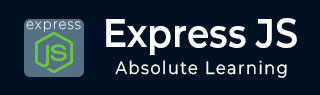
- ExpressJS Tutorial
- ExpressJS - Home
- ExpressJS - Overview
- ExpressJS - Environment
- ExpressJS - Hello World
- ExpressJS - Routing
- ExpressJS - HTTP Methods
- ExpressJS - URL Building
- ExpressJS - Middleware
- ExpressJS - Templating
- ExpressJS - Static Files
- ExpressJS - Form Data
- ExpressJS - Database
- ExpressJS - Cookies
- ExpressJS - Sessions
- ExpressJS - Authentication
- ExpressJS - RESTful APIs
- ExpressJS - Scaffolding
- ExpressJS - Error handling
- ExpressJS - Debugging
- ExpressJS - Best Practices
- ExpressJS - Resources
- ExpressJS Useful Resources
- ExpressJS - Quick Guide
- ExpressJS - Useful Resources
- ExpressJS - Discussion
ExpressJS - URL Building
We can now define routes, but those are static or fixed. To use the dynamic routes, we SHOULD provide different types of routes. Using dynamic routes allows us to pass parameters and process based on them.
Here is an example of a dynamic route −
var express = require('express'); var app = express(); app.get('/:id', function(req, res){ res.send('The id you specified is ' + req.params.id); }); app.listen(3000);
To test this go to http://localhost:3000/123. The following response will be displayed.
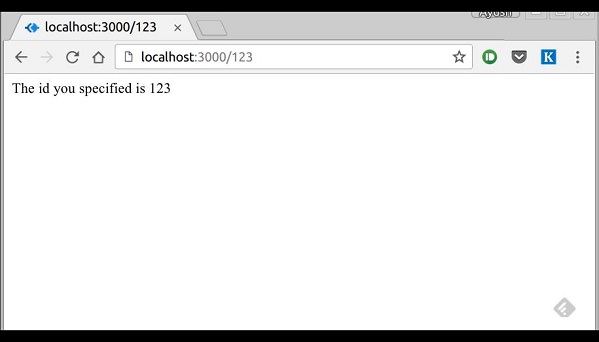
You can replace '123' in the URL with anything else and the change will reflect in the response. A more complex example of the above is −
var express = require('express'); var app = express(); app.get('/things/:name/:id', function(req, res) { res.send('id: ' + req.params.id + ' and name: ' + req.params.name); }); app.listen(3000);
To test the above code, go to http://localhost:3000/things/tutorialspoint/12345.
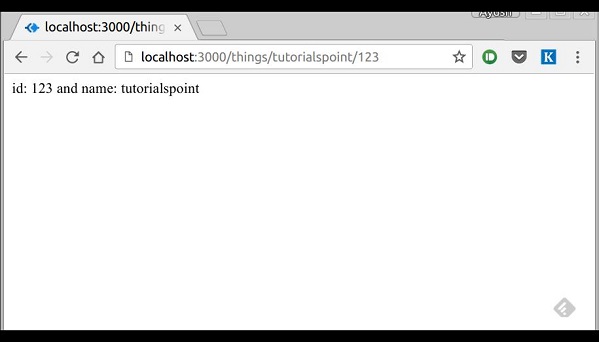
You can use the req.params object to access all the parameters you pass in the url. Note that the above 2 are different paths. They will never overlap. Also if you want to execute code when you get '/things' then you need to define it separately.
Pattern Matched Routes
You can also use regex to restrict URL parameter matching. Let us assume you need the id to be a 5-digit long number. You can use the following route definition −
var express = require('express'); var app = express(); app.get('/things/:id([0-9]{5})', function(req, res){ res.send('id: ' + req.params.id); }); app.listen(3000);
Note that this will only match the requests that have a 5-digit long id. You can use more complex regexes to match/validate your routes. If none of your routes match the request, you'll get a "Cannot GET <your-request-route>" message as response. This message be replaced by a 404 not found page using this simple route −
var express = require('express'); var app = express(); //Other routes here app.get('*', function(req, res){ res.send('Sorry, this is an invalid URL.'); }); app.listen(3000);
Important − This should be placed after all your routes, as Express matches routes from start to end of the index.js file, including the external routers you required.
For example, if we define the same routes as above, on requesting with a valid URL, the following output is displayed. −
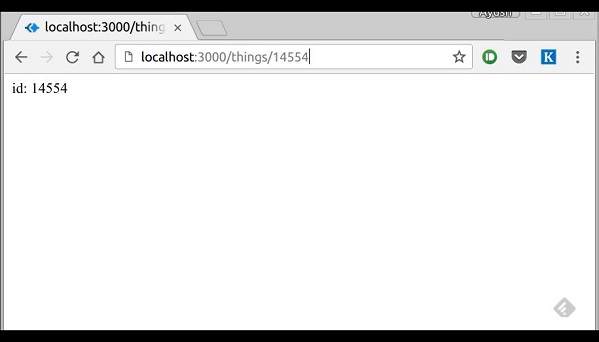
While for an incorrect URL request, the following output is displayed.
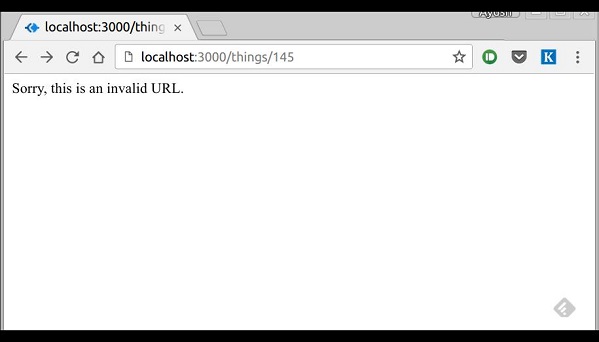