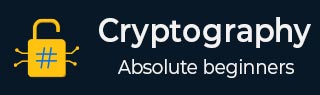
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - XOR Encryption
XOR functions are extensively used in cryptography, digital logic circuits, network protection, and programming languages like Python, Java, and C++. The XOR operation is also known as Exclusive OR.
The logical function XOR (exclusive OR) compares two binary digits and returns 1 if they differ. The XOR function returns 0 if the two bits are exactly equal. In other words, XOR gives 1 if one of the binary digits is 1, but not both.
So as we have discussed above, XOR can be used for encryption in cryptography. So with the help of XOR encryption we can encrypt data that is impossible to crack using brute-force. For example, creating random encryption keys to match the right key.
Implementation Using Python
We can implement the XOR Encryption using below methods in Python −
Using a For Loop and a Fixed Key
Using Random Key Generation
Using the base64 module
In the next sections, we will try to explain each of these ways in brief so that you can gain a better understanding of XOR Encryption using Python.
Approach 1: Using a for loop and a fixed key
XOR encryption is a simple symmetric encryption algorithm wherein each individual inside the input message is combined with a key with the help of the XOR operation. So we are able to encrypt our input message character by person the use of XOR operation and right here we are able to use a hard and fast key for every man or woman.In our case the key is 'T'. And then the outcome of the XOR operation is converted back to a character.
The process of XOR-ing every character with the key hides the original message, which makes it difficult to read without the encryption key.
Here is an example of performing XOR encryption on a plaintext −
Example
#Our XOR encryption function def xorEncrypt(inpString): my_xor_key = 'T' length = len(inpString) encrypted_msg = "" for i in range(length): encrypted_msg += chr(ord(inpString[i]) ^ ord(my_xor_key)) return encrypted_msg demoMsg = "This is Tutorialspoint" # Encrypt the string print("Original Message: ", demoMsg) encrypted_message = xorEncrypt(demoMsg) print("Our Encrypted Message: ", encrypted_message)
Following is the output of the above example −
Input/Output
Original Message: This is Tutorialspoint Our Encrypted Message: <='t='t! ;&=58'$;=:
Approach 2: Using Random Key Generation
In this approach we are going to use random key generation using the random module of Python to create a key of the same length as the message for XOR encryption. It uses the random.choice() method to randomly select ASCII characters from the string module.
In the xorEncrypt function, the generated key and the message are passed as arguments. The zip function is used to iterate over corresponding characters of the message and the key simultaneously.
This process adds randomness and security to the encryption process.
Below is the Python implementation for the XOR Encryption using random key generation −
Example
import random import string #Generate random key def generate_key(length): return ''.join(random.choice(string.printable) for _ in range(length)) # XOR Encryption function def xorEncrypt(message, key): encrypted = ''.join(chr(ord(char) ^ ord(key_char)) for char, key_char in zip(message, key)) return encrypted #Function execution and input message message = "Hello, Everyone!" key = generate_key(len(message)) encrypted_msg = xorEncrypt(message, key) print("Encrypted Message:", encrypted_msg) decrypted_msg = xorEncrypt(encrypted_msg, key) print("Decrypted Message:", decrypted_msg)
Following is the output of the above example −
Input/Output
Encrypted Message: e-2( vH#R\29 Decrypted Message: Hello, Everyone!
Approach 3: Using the base64 module
The base64 module in Python offers functions to encode and decode information the use of Base64 encoding. So we will carry out XOR encryption on the input message with the help of a consumer-described key. After encrypting the message, we will encode it using base64 to create an encrypted message.
Here is an implementation that shows XOR encryption with a user-defined key and then encodes the encrypted message using base64 −
Example
import base64 def xorEncrypt(message, key): encrypted = ''.join(chr(ord(char) ^ ord(key[i % len(key)])) for i, char in enumerate(message)) return encrypted def main(): try: message = "Hello my name is Azaad" key = "T" print("Our Original Message is:", message) # Encrypt the message using XOR encryption encrypted_message = xorEncrypt(message, key) # Encode the encrypted message using base64 encoded_message = base64.b64encode(encrypted_message.encode()).decode() print("Encrypted and encoded message:", encoded_message) except Exception as e: print("An error occurred:", str(e)) if __name__ == "__main__": main()
Following is the output of the above example −
Input/Output
Our Original Message is: Hello my name is Azaad Encrypted and encoded message: HDE4ODt0OS10OjU5MXQ9J3QVLjU1MA==
Implementation using Java
In this implementation we will use Java's Base64 class to implement the Base64 encoding and decoding functionality. This class contains only static methods for getting encoders and decoders for the Base64 encoding method. The implementation of this class supports the Base64 types specified in RFC 4648 and RFC 2045.
Example
So below is the implementation of Base64 encoding and decoding using Java's Base64 class:
import java.util.Base64; public class Base64Class { public static void main(String[] args) { // Encoding process String plaintext = "Hello, Tutorialspoint!"; String encoded_message = Base64.getEncoder().encodeToString(plaintext.getBytes()); System.out.println("The Encoded string: " + encoded_message); // Decoding process byte[] decodedBytes = Base64.getDecoder().decode(encoded_message); String decodedString = new String(decodedBytes); System.out.println("The Decoded string: " + decodedString); } }
Following is the output of the above example −
Input/Output
The Encoded string: SGVsbG8sIFR1dG9yaWFsc3BvaW50IQ== The Decoded string: Hello, Tutorialspoint!
Implementation using C++
The C++ code implements a function to encode a string to Base64. Here we will basically use vector and iomanip library to implement Base64. Vector library contains definitions and implementations of useful data structures and functions and iomanip is a library which is basically used to manipulate the output of C++ program.
This code basically converts every character to its corresponding Base64 cost and appends it to the output string. Similarly, the deciphering feature converts each Base64 character back to its unique value and appends it to the output string. This way, the authentic string is reconstructed.
Example
Following is the implementation using C++
#include <iostream> #include <string> #include <vector> #include <iomanip> #include <sstream> std::string base64_encode(const std::string &in) { std::string out; std::string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "abcdefghijklmnopqrstuvwxyz" "0123456789+/"; int val = 0, valb = -6; for (unsigned char c : in) { val = (val << 8) + c; valb += 8; while (valb >= 0) { out.push_back(base64_chars[(val >> valb) & 0x3F]); valb -= 6; } } if (valb > -6) out.push_back(base64_chars[((val << 8) >> (valb + 8)) & 0x3F]); while (out.size() % 4) out.push_back('='); return out; } std::string base64_decode(const std::string &in) { std::string out; std::string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "abcdefghijklmnopqrstuvwxyz" "0123456789+/"; std::vector<int> base64_reverse_chars(256, -1); for (int i = 0; i < 64; i++) { base64_reverse_chars[base64_chars[i]] = i; } int val = 0, valb = -8; for (unsigned char c : in) { if (base64_reverse_chars[c] == -1) break; val = (val << 6) + base64_reverse_chars[c]; valb += 6; if (valb >= 0) { out.push_back(char((val >> valb) & 0xFF)); valb -= 8; } } return out; } int main() { std::string plaintext = "Hello, Everyone. This world is beautiful!"; std::string es = base64_encode(plaintext); std::cout << "The Encoded string: " << es << std::endl; std::string ds = base64_decode(es); std::cout << "The Decoded string: " << ds << std::endl; return 0; }
Following is the output of the above example −
Input/Output
The Encoded string: SGVsbG8sIEV2ZXJ5b25lLiBUaGlzIHdvcmxkIGlzIGJlYXV0aWZ1bCE= The Decoded string: Hello, Everyone. This world is beautiful!
Advantages
There are some advantages to use XOR Encryption process for encrypting you message −
XOR encryption is very simple and straightforward to implement and understand.
This method is computationally efficient which makes it a fast encryption method.
XOR encryption is a type of symmetric encryption so it simplifies key management as compared to asymmetric encryption methods.
It can be easily customized by using different keys, allowing for a wide range of encryption variations.
Disadvantages
Below are some disadvantages of XOR encryption to keep in mind −
XOR encryption needs a secure key exchange process to ensure the confidentiality of the key.
It is vulnerable to known plaintext attacks, in which an attacker can guess the patterns in the plaintext and ciphertext to get the key or decrypt other messages.
In this technique we are reusing the same key for multiple messages. This thing can weaken the security of XOR encryption.
It is not suitable for encrypting large amounts of data, because it lacks security features provided by modern encryption algorithms like AES.
To Continue Learning Please Login