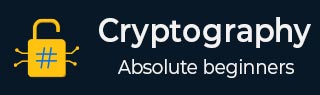
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Symmetric Algorithms
- Data Encryption Standard
- Triple DES
- Advanced Encryption Standard
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Key Generation
Key Management
The administration and security of the keys used to encrypt and decrypt data is referred to as key management. This covers −
Generation
Storage
Distribution
Revocation

Key Generation
People use a variety of online softwares and websites these days to transfer data between locations. Cryptographic-based methods are used in software and applications to protect user data from hackers. The cryptographic algorithm's foundation is the encryption and decryption procedures, which are executed with the use of a key. While one producing key directly interprets the password, cryptographic methods use automatic key generation that begins with the user's password.
The same key is used by symmetric algorithms for both encryption and decryption. Symmetric algorithms use a second or subsidiary approach for key generation. This key generation mechanism protects the password from several types of key attacks.
In cryptography, these keys serve as the means to encode and decode the data. Tools or software designed specifically for generating keys are known as key generators or keygens.
What are keys?
In cryptography, a key is just a really large number. Cryptography relies strongly on keys, which are used in operations like hashing, encryption, and signing to give desired qualities like authenticity (confirming the source of the information), confidentiality (keeping it hidden), and integrity (preventing it from being altered).
The number of binary digits, or "bits," or 1s and 0s, required to represent a key's value is how long the key is measured. Typically, keys consist of hundreds or maybe even thousands of bits. This provides a compromise between security and computational speed; if the key is too lengthy, cryptography becomes impractical and if it is too short, it is not secure.
Key types
Keys in cryptography can be of two types:
Symmetric Keys
Data is usually encrypted and decrypted using symmetric keys. They function to securely "lock" away information (i.e., encrypt it) so that only the owner of the key may "unlock" (i.e., decrypt it), making them comparable to physical keys. The fact that the same key is used for both encryption and decryption gives rise to the term "symmetric."
Symmetric keys need to be kept secret, long, and random in order to be considered secure, or "strong." Without this secret key, an attacker is unable to decrypt the data, even if they are aware of the encryption scheme that was used. An attacker cannot guess (or "brute force") a strong key and a high-quality symmetric encryption method in a reasonable amount of time, not even with a highly powerful computer capable of trying millions of key guesses per second.
Used for encrypting and decrypting data. The key is shared by both parties.
Asymmetric Keys
Asymmetric keys are typically found in pairs, each of which consists of a "public key" and a "private key" that are linked mathematically. The public key is intended for public distribution, while the private key must be kept secret. "Public key cryptography" is made possible by these keys.
The data can be encrypted with the help of the public key by anybody, but it can only be decrypted or decoded by the owner of the private key because of the features of asymmetric keys. Sending someone a private message is benificial because all they need is your public key.
Sending someone a private message is advantageous since all they need is your public key.
Verifying a message's authenticity also can be executed via asymmetric keys. To create a "digital signature," the message is first compressed the usage of a device referred to as a "hash function," and the ensuing "fingerprint" is then encrypted with the private key. Then, all people may additionally fast and without problems decrypt this signature using the sender's public key to confirm that it produces the equal end result as hashing the message themselves; if not, most effective the owner of the associated personal key can signed it.
Key Life−Cycle
The period of time from the moment a key is created until it is completely destroyed is referred to as the key life-cycle. Many things can happen to a key in its lifetime. It may be authorised, backed up, shared, or withdrawn. Also, a key can be updated on a regular basis (that is, its value changes even though it logically remains the same with the same meta-data). This is a smart security practice because the longer a key is used, the higher chances that it will be compromised.
Generating Symmetric Keys
Symmetric keys are often created using random number generators (RNGs) or pseudorandom number generators (PRNGs).
To create random numbers, use programming libraries or built-in functions in your programming language of your choice.
Make sure that random number creation is secure and unpredictable.
Example
Here is a simple example of symmetric key generation in Python using the secrets module −
This code uses Python's secrets module to generate cryptographically strong random numbers suited for creating keys. The generate_symmetric_key function produces a random key of a given length in bytes. These keys are very much important for encrypting and decrypting data. For added security, the generated key is displayed in a hexadecimal format, making it difficult for unauthorized parties to interpret.
import secrets def generate_symmetric_key(key_length): # Generate a random key with the specified length key = secrets.token_bytes(key_length) return key # Create a symmetric key with a length of 32 bytes (256 bits) key = generate_symmetric_key(32) print("Symmetric Key:", key.hex())
Output
Symmetric Key: 58441e28a9515d10aa56d7f379e7320922211088a9dcd927278c42dc024d37df
Generating Asymmetric Key
A public key and a private key are the two linked parts of an asymmetric key. Many cryptographic toolkits contain methods such as RSA and ECC for generating these keys. To create an asymmetric key, you will need a public key and its corresponding private key. While the public key can be shared publicly, the private key must be kept secret.
You may use cryptographic libraries, like the cryptography in Python, for asymmetric key generation. Here is an example using RSA −
Example
This code creates an RSA key pair with a key size of 2048 bits. It then serializes the keys into PEM format for simpler storage and use. Finally, it prints the generated private and public keys.
from cryptography.hazmat.backends import default_backend from cryptography.hazmat.primitives.asymmetric import rsa from cryptography.hazmat.primitives import serialization def generate_asymmetric_keypair(): # generate an RSA key pair private_key = rsa.generate_private_key( public_exponent=65537, key_size=512, backend=default_backend() ) # extract the public key public_key = private_key.public_key() # change keys to PEM format. private_key_pem = private_key.private_bytes( encoding=serialization.Encoding.PEM, format=serialization.PrivateFormat.PKCS8, encryption_algorithm=serialization.NoEncryption() ) public_key_pem = public_key.public_bytes( encoding=serialization.Encoding.PEM, format=serialization.PublicFormat.SubjectPublicKeyInfo ) return private_key_pem, public_key_pem # generate an RSA key pair private_key, public_key = generate_asymmetric_keypair() print("Private Key:\n", private_key.decode()) print("Public Key:\n", public_key.decode())
Output
Private Key: -----BEGIN PRIVATE KEY----- MIIBVAIBADANBgkqhkiG9w0BAQEFAASCAT4wggE6AgEAAkEAsFw/Vd5hyAsDQdzM yL2igxDU/1+d57cK3TW6GJKLB8lmYulgxzn2ngXeWoz9o2SHtcQtplnlQM/WgtQl PUjnLwIDAQABAkAOSKpojgZlaV7uKq/7YkCTVP2rYVcsuUqL+BoGe3f/PVI4gwn2 EvCUC77/RNoaFd/cy2TEUM6ihyDtK93DulQBAiEA3E0puSNJs0Cq3s/ZN9lNq6gQ UgDgx8j6vDyroEivHoECIQDM8EMawf1yStHhfo5H3cSVUUJZDlf77sbaZ36cgsaN rwIhANpXd/TQbqlEfJXcttNNgleReKttx1r7bNbH4uo3X6kBAiB2I/tLLZGBlYgw SdiTrVBqE5H/7Ljjzt5rgUYHy4vSmQIgPean16P7bUik+o56/NGVo4VTJpbYZbpd i6NkyEW0pWY= -----END PRIVATE KEY----- Public Key: -----BEGIN PUBLIC KEY----- MFwwDQYJKoZIhvcNAQEBBQADSwAwSAJBALBcP1XeYcgLA0HczMi9ooMQ1P9fnee3 Ct01uhiSiwfJZmLpYMc59p4F3lqM/aNkh7XELaZZ5UDP1oLUJT1I5y8CAwEAAQ== -----END PUBLIC KEY-----
Considerations for Key Length
There are certain Considerations to be followed for generating Symmetric and Asymmetric Keys −
The encryption strength is determined by the key length.
Longer keys provide greater security, but they may need more computational resources.
Symmetric keys often have lengths of 128 bits or more.
Asymmetric keys in RSA typically have a length of 2048 bits or more.
Testing and Validating Keys
After securely creating the keys we must test and validate it in your cryptographic system to make sure that they are working as expected.
Then, validate the keys' security by evaluating how vulnerable they are to known attacks and errors.
Summary
Overall, key generation is essential for preserving confidentiality, integrity, and validity of data in cryptographic systems. To minimize the risk of cryptographic attacks, carefully analyze randomization, algorithm selection, key size, and secure storage methods.
To Continue Learning Please Login