
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
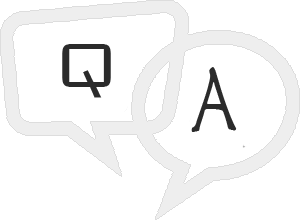
Q 1 - What is the output of the below code snippet?
#include<stdio.h> main() { for()printf("Hello"); }
Answer : D
Explanation
Compiler error, semi colons need to appear though the expressions are optional for the ‘for’ loop.
Q 2 - What is the output of the following program?
#include<stdio.h> main() { int i = 1; while(i++<=5); printf("%d ",i++); }
Answer : B
Explanation
6, there is an empty statement following ‘while’.
Q 3 - Choose the application option for the following program?
#include<stdio.h> main() { int *p, **q; printf("%u\n", sizeof(p)); printf("%u\n", sizeof(q)); }
A - Both the printf() will print the same value
B - First printf() prints the value less than the second.
Answer : A
Explanation
Irrespective of any data type every type of pointer variable occupies same amount of memory.
Q 4 - Choose the invalid predefined macro as per ANSI C.
Answer : D
Explanation
There is no macro define with the name __C++__, but __cplusplus is defined by ANSI)
Q 5 - What is the output of the following program?
#include<stdio.h> #define sqr(i) i*i main() { printf("%d %d", sqr(3), sqr(3+1)); }
Answer : B
Explanation
The equivalent expansion is -> printf("%d %d",3*3,3+1*3+1);
Q 6 - Choose the correct program that round off x value (a float value) to an int value to return the output value 4,
A - float x = 3.6;
int y = (int)(x + 0.5); printf ("Result = %d\n", y );
B - float x = 3.6;
int y = int(x + 0.5); printf ("Result = %d\n", y );
C - float x = 3.6;
int y = (int)x + 0.5 printf ("Result = %d\n", y );
D - float x = 3.6;
int y = (int)((int)x + 0.5) printf ("Result = %d\n", y );
Answer : A
Explanation
#include <math.h> #include <stdio.h> int main() { float x = 3.6; int y = (int)(x + 0.5); printf ("Result = %d\n", y ); return 0; }
Q 7 - What function can be used to free the memory allocated by calloc()?
Answer : C
Explanation
calloc(): Allocates space for an array elements, initializes to zero and then returns a pointer to memory.
Free(): Dellocate the space allocated by calloc()
Q 8 - What do you mean by “int (*ptr)[10]”
A - ptr is an array of pointers to 10 integers
B - ptr is a pointer to an array of 10 integers
Answer : B
Explanation
Explanation: with or without the brackets surrounding the *p, still the declaration says it’s an array of pointer to integers.
Q 9 - In the given below code, what will be return by the function get ()?
#include<stdio.h> int get(); int main() { const int x = get(); printf("%d", x); return 0; } int get() { return 40; }
Answer : A
Explanation
Firstly, “int get()” which is a get() function prototype returns an integer value without any parameters.
Secondly, const int x = get(); The constant variable x is declared as an integer data type and initialized with the value of get(). Hence, the value of get() is 40, printf("%d", x); will print the value of x, that means; 40. So, the program output will be 40.
#include<stdio.h> int get(); int main() { const int x = get(); printf("%d", x); return 0; } int get() { return 40; }
Q 10 - extern int fun(); - The declaration indicates the presence of a global function defined outside the current module or in another file.
Answer : A
Explanation
Extern is used to resolve the scope of global identifier.