
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Constants
- C - Literals
- C - Escape sequences
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Infinite Loop in C
An infinite loop is a loop that never ends own its own. A program in C is a list of instructions. By default, the instructions are executed in a sequential order, from the first instruction to the last. If the flow of execution is directed to any of the earlier instructions, it constitutes a loop. Generally, a loop is designed to perform a certain number of iterations, however, if the loop body keeps on executing repeatedly, it is called an infinite loop.
Flowchart of Infinite Loop
If the flow of the program is unconditionally directed to any previous step, an infinite loop is created, as shown in the following flowchart −
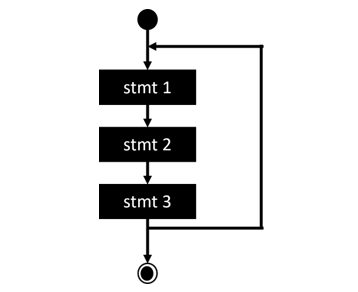
An infinite loop is very rarely created intentionally. In case of embedded headless systems and server applications, the application runs in an infinite loop to listen to the client requests. In other circumstances, infinite loops are mostly created due to inadvertent programming errors.
Program Hangs Due to Infinite Loops
We usually hear people saying their computer or phone getting hanged and that it is not doing anything. As a matter of fact, because the system gets caught in a endless loop, it is performing a task again and again and cannot come out of the loop, hence it cannot respond to user actions.
In C, the keywords such as whilewhile, do-while and for are used to design looping constructs. If the program control goes back unconditionally or the condition for redirection is not satisfied, then the compiler keeps on executing the statements in the loop body, as there is no mechanism to stop the loop.
Stopping an Infinite Loop
When the program enters an infinite loop, it doesn’t stop on its own. It has to be forcibly stopped. This is done by pressing "Crtrl + C" or "Ctrl + Break" or any other key combination depending on the operating system.
Example
The following C program enters in an infinite loop −
#include <stdio.h> int main(){ // local variable definition int a = 0; // do loop execution LOOP: a++; printf("a: %d\n", a); goto LOOP; return 0; }
Output
When executed, the above program prints incrementing values of "a" from 1 onwards, but it doesn’t stop. It will have to be forcibly stopped by pressing "Ctrl + Break" keys.
a: 1 a: 2 ... ... a: 10 a: 11 ... ...
Infinite While Loop
The while keyword is used to form a counted loop. The loop is scheduled to repeat till the value of some variable successively increments to a predefined value. However, if the programmer forgets to put the increment statement within the loop body, the test condition doesn’t arrive at all, hence it becomes endless or infinite.
Example 1
Take a look at the following example −
#include <stdio.h> // infinite while loop int main(){ int i = 0; while (i <= 10){ // i++; printf("i: %d\n", i); } return 0; }
Output
Since the increment statement is commented out here, the value of "i" continues to remain "0", hence the output shows "i: 0" continuously until you forcibly stop the execution.
i: 0 i: 0 i: 0 ... ...
Example 2
The parenthesis of while keyword has a Boolean expression that initially evaluates to True, and is eventually expected to become False. Note that any non-zero number is treated as True in C. Hence, the following while loop is an infinite loop:
#include <stdio.h> // infinite while loop int main(){ while(1){ printf("Hello World \n"); } return 0; }
Output
It will keep printing "Hello World" endlessly.
Hello World Hello World Hello World ... ...
The syntax of while loop is as follows −
while (condition){ . . . . . . }
Note that the there is no semicolon symbol in front of while, indicating that the following code block (within the curly brackets) is the body of the loop. If we place a semicolon, the compiler treats this as a loop without body, and hence the while condition is never met.
Example 3
In the following code, an increment statement is put inside the loop block, but because of the semicolon in front of while, the loop becomes infinite.
#include <stdio.h> // infinite while loop int main(){ int i = 0; while(i < 10);{ i++; printf("Hello World \n"); } return 0; }
Output
When the program is run, it won't print the message "Hello World". There is no output because the while loop becomes an infinite loop with no body.
Infinite For Loop
The for loop in C is used for performing iteration of the code block for each value of a variable from its initial value to the final value, incrementing it on each iteration.
Take a look at its syntax −
for (initial val; final val; increment){ . . . . . . }
Example 1
Note that all the three clauses of the for statement are optional. Hence, if the middle clause that specifies the final value to be tested is omitted, the loop turns infinite.
#include <stdio.h> // infinite for loop int main(){ int i; for(i=1; ; i++){ i++; printf("Hello World \n"); } return 0; }
Output
The program keeps printing Hello World endlessly until you stop it forcibly, because it has no effect of incrementing "i" on each turn.
Hello World Hello World Hello World ... ...
You can also construct a for loop for decrementing the values of a looping variable. In that case, the initial value should be greater than the final test value, and the third clause in for must be a decrement statement (using the "--" operator).
If the initial value is less than the final value and the third statement is decrement, the loop becomes infinite. The loop still becomes infinite if the initial value is larger but you mistakenly used an increment statement.
Hence, both the for loops result in an infinite loop.
Example 2
Take a look at the following example −
#include <stdio.h> int main(){ // infinite for loop for(int i = 10; i >= 1; i++){ i++; printf("Hello World \n"); } }
Output
The program will print a series of "Hello World" in an infinite loop −
Hello World Hello World Hello World ... ...
Example 3
Take a look at the following example −
#include <stdio.h> int main(){ // infinite for loop for(int i = 1; i <= 10 ; i--){ i++; printf("Hello World \n"); } }
Output
The program keeps printing "Hello World" in a loop −
Hello World Hello World Hello World ... ...
Example 4
If all the three statements in the parenthesis are blank, the loop obviously is an infinite loop, as there is no condition to test.
#include <stdio.h> int main(){ int i; // infinite for loop for ( ; ; ){ i++; printf("Hello World \n"); } }
Output
The program prints "Hello World" in an endless loop −
Hello World Hello World Hello World ... ...
There may be certain situations in programming where you need to start with an unconditional while or for statement, but then you need to provide a way to terminate the loop by placing a conditional break statement.
Example 5
In the following program, there is no test statement in for, but we used a break statement to make it a finite loop.
#include <stdio.h> int main(){ // infinite while loop for(int i = 1; ; ){ i++; printf("Hello World \n"); if(i == 5) break; } return 0; }
Output
The program prints "Hello World" till the counter variable reaches 5.
Hello World Hello World Hello World Hello World
Infinite loops are mostly unintentionally created as a result of programming bug. Even if the looping keyword doesn’t specify the termination condition, the loop has to be terminated with the break keyword.