
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Constants
- C - Literals
- C - Escape sequences
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C - Array of Strings
In C, a string is a one−dimensional array of char data type. It is a sequence of characters terminated by null character (\0). An array of strings therefore is an array of char arrays. An array of strings in C is a two−dimensional array of char type. Each row stores one null terminated string.
To declare a string we use the statement −
char string[] = {'H', 'e', 'l', 'l', 'o', '\0'};
or
char string = "Hello";
To construct an array of strings, the following syntax is used −
char strings [no of strings] [max size of each string];
Let us declare and initialize an array of strings to store names of Ten computer languages, each with the maximum length of fifteen characters.
char langs [10][15] = { "PYTHON", "JAVASCRIPT", "PHP", "NODE JS", "HTML", "KOTLIN", "C++", "REACT JS", "RUST", "VBSCRIPT" };
How is this array stored in the memory? We know that each char type occupies 1 byte in the memory. Hence, this array will be allocated a block of 150 bytes. Although this block is contagious memory locations, each group of 15 bytes constitutes a row.
Assuming that the array is situated at memory address 1000, the logical layout of this array can be shown as in the following figure −
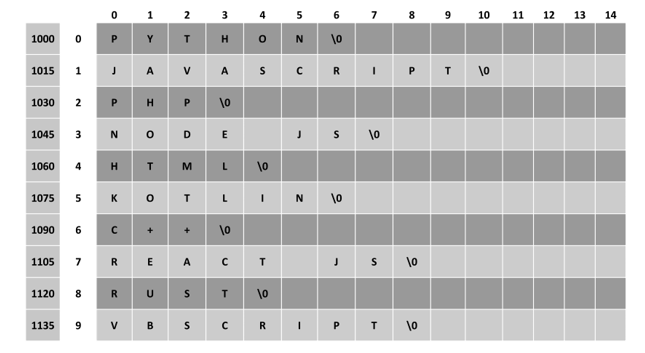
We can employ a loop to print the contents of this string array.
Example
#include <stdio.h> int main () { char langs [10][15] = { "PYTHON", "JAVASCRIPT", "PHP", "NODE JS", "HTML", "KOTLIN", "C++", "REACT JS", "RUST", "VBSCRIPT" }; int i; for (i=0; i<10; i++){ printf("%s\n", langs[i]); } return 0; }
Output
PYTHON JAVASCRIPT PHP NODE JS HTML KOTLIN C++ REACT JS RUST VBSCRIPT
Note that, the size of each string is not equal to the row size in the declaration of the array. The \0 symbol signals the termination of the string, and the remaining cells in the row are empty. Thus a substantial part of the memory allocated to the array is unused and thus wasted.
To use the memory more efficiently, we can use the pointers. Instead of a two−dimensional char array, we declare a one−dimensional array of char * type.
char *langs [10] = { "PYTHON", "JAVASCRIPT", "PHP", "NODE JS", "HTML", "KOTLIN", "C++", "REACT JS", "RUST", "VBSCRIPT" };
In the two−dimensional array of characters, the strings occupied 150 bytes. As against this, in array of pointers, the strings occupy far less number of bytes, as each string is randomly allocated memory as shown below −
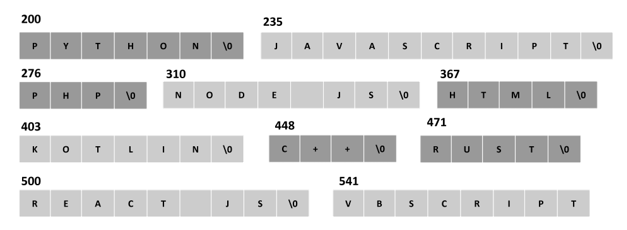
The lang[] array is an array of pointers of individual strings.
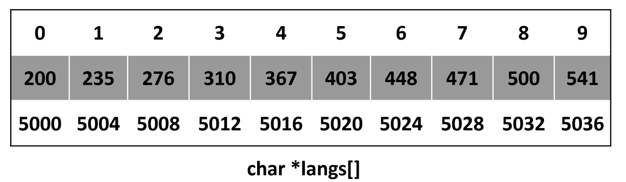
We can use the for loop as follows to print the array of strings −
Example
#include <stdio.h> int main(){ char *langs [10] = { "PYTHON", "JAVASCRIPT", "PHP", "NODE JS", "HTML", "KOTLIN", "C++", "REACT JS", "RUST", "VBSCRIPT" }; int i; for (i=0; i<10; i++) printf("%s\n", langs[i]); return 0; }
Output
PYTHON JAVASCRIPT PHP NODE JS HTML KOTLIN C++ REACT JS RUST VBSCRIPT
The langs is a pointer to an array of 10 strings. Therefore, if langs[0] points to address 5000 then langs+1 will point to address 5004 which stores the pointer to the second string..
Hence, we can also use the following variation of the loop to print the array of strings.
int i; for (i=0; i<10; i++){ printf("%s\n", *(langs+i)); }
When strings are stored in array, there are a lot use cases. Let study some of the use cases.
Find the string with largest length
We store the length of first string and its position (which is 0) in the variables l and p respectively. Inside a for loop, we update these variables whenever a string of larger length is found.
Example
#include <stdio.h> #include <string.h> int main () { char langs [10][15] = { "PYTHON", "JAVASCRIPT", "PHP", "NODE JS", "HTML", "KOTLIN", "C++", "REACT JS", "RUST", "VBSCRIPT" }; int i; int l = strlen(langs[0]); int p = 0; for (i=0; i<10; i++){ if (strlen(langs[i])>=l){ l=strlen(langs[i]); p=i; } } printf("Language with longest name: %s Length: %d", langs[p], l); return 0; }
Output
Language with longest name: JAVASCRIPT Length: 10
Sort string array in ascending order
We need to use the strcmp() function for comparison of two strings. If the value of comparison of strings is greater than 0, it means that the first argument string appears later than the second in alphabetical order. We then swap these two strings with strcmp() function.
Example
#include <stdio.h> #include <string.h> int main () { char langs [10][15] = { "PYTHON", "JAVASCRIPT", "PHP", "NODE JS", "HTML", "KOTLIN", "C++", "REACT JS", "RUST", "VBSCRIPT" }; int i, j; char temp[15]; for (i=0; i<9; i++){ for (j=i+1; j<10; j++){ if (strcmp(langs[i], langs[j])>0){ strcpy(temp, langs[i]); strcpy(langs[i], langs[j]); strcpy(langs[j], temp); } } } for (i=0; i<10; i++){ printf("%s\n", langs[i]); } return 0; }
Output
C++ HTML JAVASCRIPT KOTLIN NODE JS PHP PYTHON REACT JS RUST VBSCRIPT
In this chapter, we have learned how to declare an array of strings, and how to manipulate it with the help of string functions. The string.h header file provides a number of library functions for string manipulations. These functions will be discussed in a separate chapter in this tutorial.