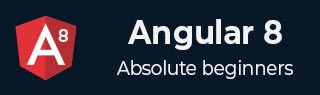
- Angular 8 Tutorial
- Angular 8 - Home
- Angular 8 - Introduction
- Angular 8 - Installation
- Creating First Application
- Angular 8 - Architecture
- Angular Components and Templates
- Angular 8 - Data Binding
- Angular 8 - Directives
- Angular 8 - Pipes
- Angular 8 - Reactive Programming
- Services and Dependency Injection
- Angular 8 - Http Client Programming
- Angular 8 - Angular Material
- Routing and Navigation
- Angular 8 - Animations
- Angular 8 - Forms
- Angular 8 - Form Validation
- Authentication and Authorization
- Angular 8 - Web Workers
- Service Workers and PWA
- Angular 8 - Server Side Rendering
- Angular 8 - Internationalization (i18n)
- Angular 8 - Accessibility
- Angular 8 - CLI Commands
- Angular 8 - Testing
- Angular 8 - Ivy Compiler
- Angular 8 - Building with Bazel
- Angular 8 - Backward Compatibility
- Angular 8 - Working Example
- Angular 9 - What’s New?
- Angular 8 Useful Resources
- Angular 8 - Quick Guide
- Angular 8 - Useful Resources
- Angular 8 - Discussion
Angular 8 - Architecture
Let us see the architecture of the Angular framework in this chapter.
Angular framework is based on four core concepts and they are as follows −
- Components.
- Templates with Data binding and Directives.
- Modules.
- Services and dependency injection.
Component
The core of the Angular framework architecture is Angular Component. Angular Component is the building block of every Angular application. Every angular application is made up of one more Angular Component. It is basically a plain JavaScript / Typescript class along with a HTML template and an associated name.
The HTML template can access the data from its corresponding JavaScript / Typescript class. Component’s HTML template may include other component using its selector’s value (name). The Angular Component may have an optional CSS Styles associated it and the HTML template may access the CSS Styles as well.
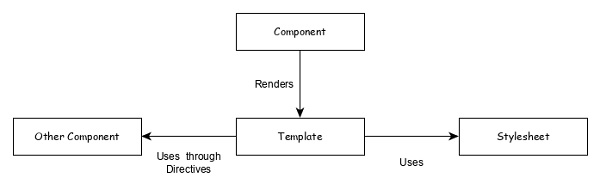
Let us analyse the AppComponent component in our ExpenseManager application. The AppComponent code is as follows −
// src/app/app.component.ts import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Expense Manager'; }
@Component is a decorator and it is used to convert a normal Typescript class to Angular Component.
app-root is the selector / name of the component and it is specified using selector meta data of the component’s decorator. app-root can be used by application root document, src/index.html as specified below
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>ExpenseManager</title> <base href="/"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/x-icon" href="favicon.ico"> </head> <body> <app-root></app-root> </body> </html>
app.component.html is the HTML template document associated with the component. The component template is specified using templateUrl meta data of the @Component decorator.
app.component.css is the CSS style document associated with the component. The component style is specified using styleUrls meta data of the @Component decorator.
AppComponent property (title) can be used in the HTML template as mentioned below −
{{ title }}
Template
Template is basically a super set of HTML. Template includes all the features of HTML and provides additional functionality to bind the component data into the HTML and to dynamically generate HTML DOM elements.
The core concept of the template can be categorised into two items and they are as follows −
Data binding
Used to bind the data from the component to the template.
{{ title }}
Here, title is a property in AppComponent and it is bind to template using Interpolation.
Directives
Used to include logic as well as enable creation of complex HTML DOM elements.
<p *ngIf="canShow"> This sectiom will be shown only when the *canShow* propery's value in the corresponding component is *true* </p> <p [showToolTip]='tips' />
Here, ngIf and showToolTip (just an example) are directives. ngIf create the paragraph DOM element only when canShow is true. Similarly, showToolTip is Attribute Directives, which adds the tooltip functionality to the paragraph element.
When user mouse over the paragraph, a tooltip with be shown. The content of the tooltip comes from tips property of its corresponding component.
Modules
Angular Module is basically a collection of related features / functionality. Angular Module groups multiple components and services under a single context.
For example, animations related functionality can be grouped into single module and Angular already provides a module for the animation related functionality, BrowserAnimationModule module.
An Angular application can have any number of modules but only one module can be set as root module, which will bootstrap the application and then call other modules as and when necessary. A module can be configured to access functionality from other module as well. In short, components from any modules can access component and services from any other modules.
Following diagram depicts the interaction between modules and its components.
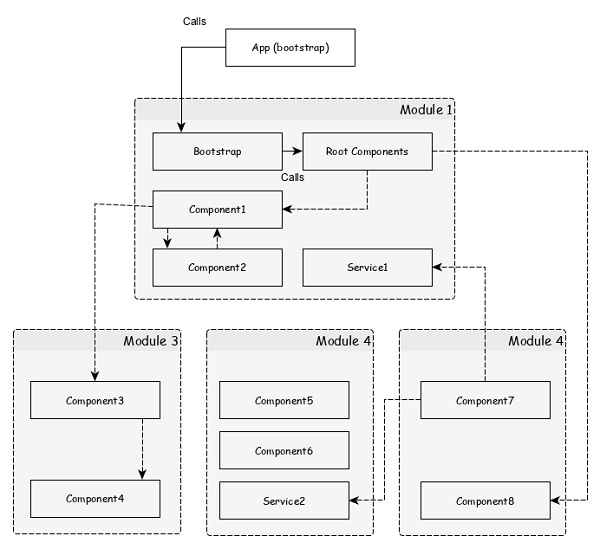
Let us check the root module of our Expense Manager application.
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Here,
NgModule decorator is used to convert a plain Typescript / JavaScript class into Angular module.
declarations option is used to include components into the AppModulemodule.
bootstrap option is used to set the root component of the AppModulemodule.
providers option is used to include the services for the AppModulemodule.
imports option is used to import other modules into the AppModulemodule.
The following diagram depicts the relationship between Module, Component and Services
Services
Services are plain Typescript / JavaScript class providing a very specific functionality. Services will do a single task and do it best. The main purpose of the service is reusability. Instead of writing a functionality inside a component, separating it into a service will make it useable in other component as well.
Also, Services enables the developer to organise the business logic of the application. Basically, component uses services to do its own job. Dependency Injection is used to properly initialise the service in the component so that the component can access the services as and when necessary without any setup.
Workflow of Angular application
We have learned the core concepts of Angular application. Let us see the complete flow of a typical Angular application.
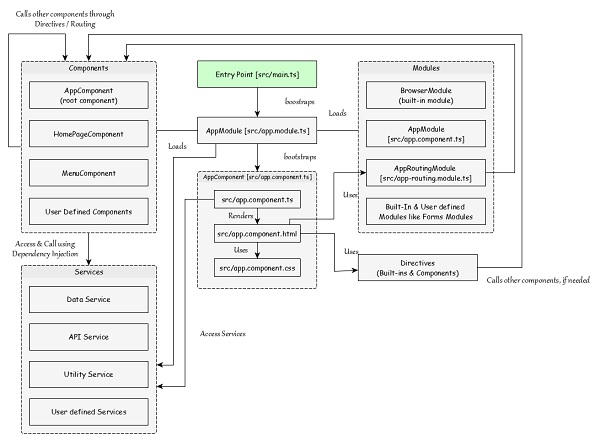
src/main.ts is the entry point of Angular application.
src/main.ts bootstraps the AppModule (src/app.module.ts), which is the root module for every Angular application.
platformBrowserDynamic().bootstrapModule(AppModule) .catch(err => console.error(err));
AppModule bootstraps the AppComponent (src/app.component.ts), which is the root component of every Angular application.
@NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Here,
AppModule loads modules through imports option.
AppModule also loads all the registered service using Dependency Injection (DI) framework.
AppComponent renders its template (src/app.component.html) and uses the corresponding styles (src/app.component.css). AppComponent name, app-root is used to place it inside the src/index.html.
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>ExpenseManager</title> <base href="/"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/x-icon" href="favicon.ico"> </head> <body> <app-root></app-root> </body> </html>
AppComponent can use any other components registered in the application.
@NgModule({ declarations: [ AppComponent AnyOtherComponent ], imports: [ BrowserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Component use other component through directive in its template using target component’s selector name.
<component-selector-name></component-selector-name>
Also, all registered services are accessible to all Angular components through Dependency Injection (DI) framework.