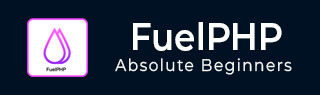
- FuelPHP Tutorial
- FuelPHP - Home
- FuelPHP - Introduction
- FuelPHP - Installation
- FuelPHP - Architecture Overview
- FuelPHP - Simple Web Application
- FuelPHP - Configuration
- FuelPHP - Controllers
- FuelPHP - Routing
- FuelPHP - Requests & Response
- FuelPHP - Views
- FuelPHP - Presenters
- FuelPHP - Models & Database
- FuelPHP - Form Programming
- FuelPHP - Validation
- Advanced Form Programming
- FuelPHP - File Uploading
- FuelPHP - Ajax
- FuelPHP - HMVC Request
- FuelPHP - Themes
- FuelPHP - Modules
- FuelPHP - Packages
- Cookie & Session Management
- FuelPHP - Events
- FuelPHP - Email Management
- FuelPHP - Profiler
- Error Handling & Debugging
- FuelPHP - Unit Testing
- Complete Working Example
- FuelPHP Useful Resources
- FuelPHP - Quick Guide
- FuelPHP - Useful Resources
- FuelPHP - Discussion
FuelPHP - Requests & Response
HTTP request and HTTP response play an important role in any web application. We need to get the complete details of the http request to process it properly. Once processed, we need to send the processed data to the client through http response.
FuelPHP provides excellent Request and Response class to read and write HTTP request and HTTP response respectively. Let us learn about both Request and Response class in this chapter.
Request
In a typical web application, the application need to parse the details of the current request. Request class provides simple methods to parse the current request to be processed by the application. Request also provides an option to create a new request by acting as a http client.
Creating a new request enables the application to request other part of the application or entirely another application and show the result. Let us learn how to parse the incoming request in this chapter and learn how to create a new request in HMVC Request chapter.
Parsing a Request
Request class provides three methods to get the details of the http request. They are as follows,
active − It is a static method, which returns the current active http request.
$currentRequest = Request::active();
param − It returns the value of the specified parameter. It contains two arguments. The first argument is the parameter name and the second argument is the value to return, if the parameter is not available in the current http request.
$param = Request::active()->param('employee_name', 'none');
params − It is same as param except it returns all the parameters as an array.
$params = Request::active()->params();
Example
Let us create a simple form and process the form using request class.
Step 1 − Create a new action, action_request in the employee controller.
public function action_request() { }
Step 2 − Call the request methods to get all the parameters of the current request.
public function action_request() { $params = Request::active()->params(); }
Step 3 − Dump the fetched parameter array.
public function action_request() { $params = Request::active()->params(); echo dump($params); }
Step 4 − Change the routing to include the parameters in the route configuration file, fuel/app/config/routes.php
'employee/request(/:name)?' => array('employee/request', 'name' => 'name'),
Now, requesting the new action, http://localhost:8080/employee/request/Jon, it will show the following response.

Response
Response class provides options to create a http response. By default, we don't need to use a response class directly in most situations. Instead, we use View (which we will learn in the next chapter) to create http response. View hides the http response from the developer and sends the response to the client using underlying Response class. In an advanced situation, we use Response class directly and create a complete http response.
Creating a Response
Response consists of headers and body. The main header is http status code. Http status code are standard codes defined in the HTTP protocol to describe the response. For example, a status code, 200 means the request is success.
Response class provides three arguments to create the http response,
- $body − body of the http response
- $status_code − status code of the http response
- $headers − optional headers as array
$body = "Hi, FuelPHP"; $headers = array ( 'Content-Type' => 'text/html', ); $response = new Response($body, 200, $headers);
Let us create a new action, action_response in the employee controller as follows.
public function action_response() { $body = "Hi, FuelPHP"; $headers = array ( 'Content-Type' => 'text/html', ); $response = new Response($body, 200, $headers); return $response; }
Result
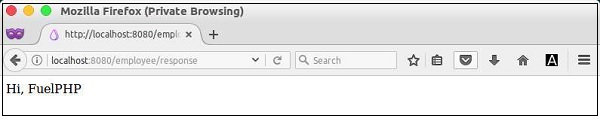
Methods
Response class provides a lot of methods to manipulate http responses. They are as follows,
forge − It is the same as response class constructor as seen above.
return Response::forge("Hi, FuelPHP", 404);
redirect − It provides option to redirecting to a URL instead of sending a response. It contains the following arguments,
a.url − destination url b. method - redirection methods. location (default) and refresh c.redirect_code - http status code. The default value is 302.
// use a URL Response::redirect('http://some-domain/index', 'refresh'); // or use a relative URI Response::redirect('employee/list');
redirect_back − It is similar to redirect method except it redirects to the previous page. We can specify the redirection page, if no back page is available.
// If there is no back page, go to the employee list page Response::redirect_back('/employee/list', 'refresh');
set_status − It provides an option to set the http status code.
$response = new Response(); $response->set_status(404);
set_header − It provides an option to set the http headers.
$response = new Response(); $response->set_header('Content-Type', 'application/pdf'); // replace previous value using third arguments $response->set_header('Content-Type', 'application/pdf', 'text/plain');
set_headers − It is same as set_header except it provides an option to set multiple headers using array.
$response = new Response(); $response->set_headers(array 'Content-Type' => 'application/pdf', 'Pragma' => 'no-cache', ));
get_header − It enables to get the previous set header details.
$response = new Response(); $response->set_header('Pragma', 'no-cache'); // returns 'no-cache' $header = $response->get_header('Pragma'); // returns array('Pragma' => 'no-cache') $header = $response->get_header();
body − It provides an option to set the body of the http response.
$response = new Response(); $response->body('Hi, FuelPHP'); // returns 'Hi, FuelPHP' $body = $response->body();
send_headers − It sends the headers to the requested client. FuelPHP uses this method to send the response to the client. Normally, we don't need to use this method.
$response->send_headers();
Send − Same as send_headers except headers may be restricted in the http response.
// send the headers as well $response->send(true); // only send the body $response->send(false); $response->send();