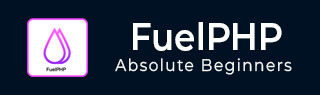
- FuelPHP Tutorial
- FuelPHP - Home
- FuelPHP - Introduction
- FuelPHP - Installation
- FuelPHP - Architecture Overview
- FuelPHP - Simple Web Application
- FuelPHP - Configuration
- FuelPHP - Controllers
- FuelPHP - Routing
- FuelPHP - Requests & Response
- FuelPHP - Views
- FuelPHP - Presenters
- FuelPHP - Models & Database
- FuelPHP - Form Programming
- FuelPHP - Validation
- Advanced Form Programming
- FuelPHP - File Uploading
- FuelPHP - Ajax
- FuelPHP - HMVC Request
- FuelPHP - Themes
- FuelPHP - Modules
- FuelPHP - Packages
- Cookie & Session Management
- FuelPHP - Events
- FuelPHP - Email Management
- FuelPHP - Profiler
- Error Handling & Debugging
- FuelPHP - Unit Testing
- Complete Working Example
- FuelPHP Useful Resources
- FuelPHP - Quick Guide
- FuelPHP - Useful Resources
- FuelPHP - Discussion
FuelPHP - Email Management
Email functionality is the most requested feature in a web framework. FuelPHP provides an elegant email class bundled as a package. It is used to send simple plain text email as well as advanced rich text email with multiple attachments. It supports the following features - Plain-text mails, HTML mails, attachments, and inline attachments.
Configuration
To enable email functionality in the application, we need to just load the email package as specified below in the main configuration file, fuel/app/config/config.php.
'always_load' => array ( 'packages' => array ( 'email', ), ),
Another option is to load the email package, the controller itself as follows.
\Package::load('email');
The email setting can be done in the main configuration file and some of the important options are as follows,
driver − Email driver such as smtp
is_html − Whether to send mail as HTML content or not
priority − Priority of the email
smtp.host − SMTP server host
smtp.port − SMTP server port
smtp.username − SMTP server username
smtp.password − SMTP server password
smtp.timeout − SMTP timeout
smtp.starttls − Whether the SMTP server needs STARTTLS command
Email API
Following are the API provided by the email and email driver class.
forge
Purpose: To create an instance of email driver. It creates the driver based on the configuration or input it receives. Email driver provides features to create and send mail. Some of the possible email drivers are smtp, sendmail, mailgun, and mandrill.
Parameter − None or array of configuration details
Returns − Returns the Email_Driver object
For example,
$email = \Email::forge(); $email = \Email::forge (array( 'driver' => 'smtp', ));
body
Purpose − To set the message body
Parameter − $body - message body
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->body('Body message'); //or pass it a View $email->body(\View::forge('my/view', $data);
alt_body
Purpose − To set the alternative message body
Parameter − $alt_body - alternative message body
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->alt_body('Body message'); //or pass it a View $email->alt_body(\View::forge('my/view', $data);
priority
Purpose − To set the mail's priority
Parameter −
$priority − value of the priority. The options are −
a. \Email::P_LOWEST * \Email::P_LOW * \Email::P_NORMAL * \Email::P_HIGH * \Email::P_HIGHEST
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->priority(\Email::P_HIGHEST);
html_body
Purpose − To set the message body in HTML format
Parameter −
$html − message body in HTML;
generate_alt − whether to generate alternative message;
auto_attach − whether to embed the image or not
Returns − Returns the current instance
For example,
$email = \Email::forge(); // Do generate the alt body, but don't auto attach images. $email->html_body(\View::forge('welcome/email', $data), true, false);
from
Purpose − To set the from address
Parameters −
$from − from email address;
$name − Name of the sender
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->from('test@test.com', 'My Name');
subject
Purpose − To set the subject of the message
Parameter − $subject - subject of the email message
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->subject('Suject of the mail message');
to
Purpose − To set the receiver email address
Parameters −
$email − email address or array of email address;
$name − receiver name
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->to('test@test.com', 'My Dear Name'); $email->to (array( 'test@test.com', 'test@test.com' => 'My Dear friend', ));
header
Purpose − To set custom header to the email message
Parameters −
$header − header type or array of header;
$value − value of the header
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email->header('X-SMTPAP', 'XXXXXXXX'); $email>reply_to (array( 'X-SMTPAP' => 'XXXXXX', 'X-SMTPAP2' > 'XXXXXA', ));
attach
Purpose − To attach a file to the email message
Parameters−
$file − file path;
$inline − whether to attach the file inline or not;
$cid − content identifier;
$mime − MIME type of the attachment file;
$name − attachment file name override
Returns − Returns the current instance
For example,
$email = \Email::forge(); $email>attach(DOCROOT.'attachments/sample_attachment.pdf');
send
Purpose − To send the mail.
Parameter −
$validate − whether to validate the email addresses
Returns − true or false
For example,
$email = \Email::forge(); try{ $email->send(); } catch(\EmailSendingFailedException $e) { // The driver could not send the mail. } catch(\EmailValidationFailedException $e) { // One or more email addresses failed validation. }
Working Email Sample
Let us use the API learned in the previous chapter and create a simple code to send a message. Following is the simplest code to send a message.
$email = Email::forge(); $email->from('someone@gmail.com', 'person1'); $email->to('anotherone@gmail.com', 'person2'); $email->subject('Add something'); $email->body('contents of mail'); $email->send();