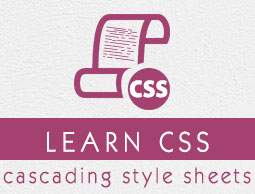
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Links
Links serve to connect and navigate between one webpage and another, with CSS properties offering options to style these links in a variety of ways.
Link States
The idea of link states involves understanding the different states in which links might exist. Various pseudo-classes can be used to style these states.
Link - A link styled with the :link pseudo class that has a destination (rather than merely being a named anchor).
Visited - A link with the :visited pseudo class style that indicates it has already been visited (it is present in the browser's history).
Hover - A link styled with the :hover pseudo class that is hovered over by the user's mouse cursor.
Focus - A focused link is styled with the :focus pseudo class. It can be focused dynamically using HTMLElement.focus(), or it can be shifted to by the user using the Tab key or any comparable keyboard shortcut.
Active - A link with the :active pseudo class applied when it is activated, such as when it is clicked.
Default Styles of Links
Properties of default links
Links are underlined.
Unvisited links are blue.
Visited links are purple
The mouse pointer changes to a little hand icon when you hover over a link.
Focused links are visually highlighted with an outline. You can navigate to links on this page using the keyboard by pressing the tab key. On Mac, use option + tab or enable Full Keyboard Access: All controls with Ctrl + F7.
Active links are styled in red. You can test this by holding down the mouse button while clicking on the link.
CSS links - Basic Example
The following example demonstrates the simple links.
<html> <head> <style> a { color: blue; text-decoration: none; } a:hover { text-decoration: underline; } </style> </head> <body> <a href="https://www.tutorialspoint.com/index.htm">Simple Link</a> </body> </html>
For clarity, make sure links are underlined; do not underline other items.
If you don't want links to be underlined, use another highlighting technique. Add link hover and focus effects as well as unique style when links are clicked.
The following CSS properties can be used to modify or disable the default styles:
CSS links- Stlying Some Links
The following example demonstrates the various types link states.
<html> <head> <style> body { width: 300px; margin: 0 auto; font-size: 1.2rem; font-family: sans-serif; } p { line-height: 1.4; } a { outline-color: transparent; } a:link { color: #007bff; } a:visited { color: #800080; } a:focus { text-decoration: none; background: #bae498; } a:hover { text-decoration: none; background: #cdfeaa; } a:active { background: #007bff; color: #cdfeaa; } </style> </head> <body> <p> Follow us on social media: <a href="https://www.youtube.com/" target="_blank">YouTube</a>, <a href="https://www.facebook.com/" target="_blank">Facebook</a>, <a href="https://www.instagram.com/" target="_blank">Instagram</a>, and <a href="https://twitter.com/" target="_blank">Twitter</a>. </p> </body> </html>
Including icons on links
By providing visual clues, integrating symbols with links especially external ones, improves user experience.
External links are typically indicated with a little arrow pointing out of a box, which helps users quickly determine the destination of a link.
CSS links - Including Icons
The following example demonstrates the usage of icons on links.
<html> <head> <style> body { width: 80%; margin: 0 auto; font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif; background-color: #f9f9f9; color: #333; } h1 { text-align: center; margin-top: 30px; } p { line-height: 1.6; } a { color: #007bff; text-decoration: none; transition: color 0.3s ease; } a:hover { color: #0056b3; } .links-list { margin-top: 20px; list-style-type: none; padding-left: 0; } .links-list li { margin-bottom: 10px; } .external-link-icon { display: inline-block; width: 16px; height: 16px; background: url("images/external-link.png") no-repeat; background-size: cover; margin-right: 5px; vertical-align: middle; } </style> </head> <body> <h1>Explore New Tutorial</h1> <p>Discover a world of learning, Tutorials Point, offering diverse courses from programming to personal development.</p> <p>Expand your knowledge, sharpen your skills, and embark on your learning journey today!</p> <ul class="links-list"> <li> <span class="external-link-icon"></span> <a href="https://www.tutorialspoint.com/css/index.htm">CSS Tutorial</a> </li> <li> <span class="external-link-icon"></span> <a href="https://www.tutorialspoint.com/python/index.htm">Python Tutorial</a> </li> <li> <span class="external-link-icon"></span> <a href="https://www.tutorialspoint.com/php/index.htm">PHP Tutorial</a> </li> </ul> </body> </html>
Links as buttons
Beyond the conventions of link styling, designers may improve user interaction and aesthetic appeal by using CSS to transform links into button-like objects.
Links may blend in with other website elements with ease by using characteristics like background-color, padding, and border-radius.
This makes for user experiences that are both engaging and navigational.
CSS links - Links as Buttons
The following example demonstrates links being styled as buttons.
<html> <head> <style> body, html { margin: 0; padding: 0; font-family: Arial, sans-serif; } .container { display: flex; gap: 10px; justify-content: center; margin-top: 20px; } .btn-link { flex: 1; text-decoration: none; outline-color: transparent; text-align: center; line-height: 3; color: white; background-color: #007bff; border: 1px solid #007bff; border-radius: 5px; transition: background-color 0.3s ease; padding: 10px 20px; margin: 0 5px; } .btn-link:hover { background-color: #0056b3; } .btn-link:active { background-color: #003366; } header { background-color: #333; color: white; text-align: center; padding: 20px 0; } h1 { margin: 0; } p { margin-top: 20px; text-align: center; } </style> </head> <body> <header> <h1>Welcome to Demo Website</h1> </header> <nav class="container"> <a href="#" class="btn-link">Home</a> <a href="#" class="btn-link">Technologies</a> <a href="#" class="btn-link">Services</a> <a href="#" class="btn-link">Gallery</a> <a href="#" class="btn-link">Contact Us</a> </nav> <p>This is a demonstration website showcasing various features.</p> </body> </html>