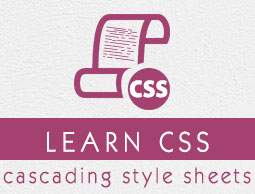
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Pseudo-class :focus Property
The :focus pseudo-class in CSS represents an element that has received focus. This class is generally triggered when a user clicks, taps on an element or selects an element using the tab key of keyboard.
:focus pseudo-class is applied to the focussed element itself. In order to select an element that contains a focussed element, use :focus-within pseudo-class.
Syntax
selector:focus{ properties }
CSS :focus Example
Following example demonstrates the use of :focus pseudo-class on a link. Click on the link or press the tab key to see the style change on link.
<html> <head> <style> a { color: darkviolet; transition: all 0.3s ease; } a:focus { outline: none; color: red; background-color: yellow; } body { margin: 5px; padding: 2rem; display: grid; font: 20px/1.4 system-ui, -apple-system, sans-serif; } </style> </head> <body> <p>The link here has a <a href="#0">change in background and foreground color</a> as it is focused.</p> </body> </html>
Following example demonstrates the use of :focus pseudo-class on a button. Click on the button to see the style change on button.
<html> <head> <style> button { display: block; margin: 2em; padding: 2px; font-weight: bold; font-size: 18px; text-align: center; color: black; background-color: #fff; border: 2px solid black; border-radius: 24px; cursor: pointer; transition: all 0.3s ease; width: 150px; height: 50px; } button:hover { color: blue; border-color: black; background-color: lightpink; } button:focus { background-color: darkred; color: white; border-radius: 2px; } </style> </head> <body> <button>Click Me!!</button> </body> </html>
Following example demonstrates the use of :focus pseudo-class on an input. Click in the input box to see the style change in the input box.
<html> <head> <style> label { display: block; font-size: 20px; color: black; width: 500px; } input[type="text"] { padding: 10px 16px; font-size: 16px; color: black; background-color: #fff; border: 1px solid #597183; border-radius: 8px; margin-top: 5px; width: 500px; transition: all 0.3s ease; } input[type="text"]:focus { background-color: lightyellow; } </style> </head> <body> <form> <label> Full Name <input type="text"> </label> </form> </body> </html>
Following example demonstrates the use of :focus pseudo-class on a dropdown. Click in the dropdown to see the style change of the dropdown.
<html> <head> <style> label { display: block; font-size: 18px; color: black; width: 500px; } select { padding: 10px 16px; font-size: 16px; color: black; background-color: #fff; border: 1px solid #597183; border-radius: 8px; margin-top: 25px; width: 300px; transition: all 0.3s ease; } select:focus { background-color: rgb(173, 233, 209); box-shadow: 20px 15px 30px yellow, -20px 15px 30px lime, -20px -15px 30px blue, 20px -15px 30px red; } </style> </head> <body> <form> <label> Ice cream Flavors: <select name="flavor"> <option>Cookie dough</option> <option>Pistachio</option> <option>Cookies & Cream</option> <option>Cotton Candy</option> <option>Lemon & Raspberry Sorbet</option> </select> </label> </form> </body> </html>
Following example demonstrates the use of :focus pseudo-class on a toggle. Check the checkbox to see the style change of the toggle.
<html> <head> <style> .toggle { position: relative; display: block; width: 100%; margin: 2em; } .toggle label { padding-right: 8px; font-family: Verdana, Geneva, Tahoma, sans-serif; font-size: 16px; line-height: 28px; color: black; } .toggle span { position: relative; display: inline-block; vertical-align: middle; cursor: pointer; } .toggle span:before { display: block; width: 50px; height: 30px; content: ""; background-color: #a9adaf; border-radius: 28px; transition: background-color 0.3s ease; } .toggle span:after { position: absolute; top: 1px; left: 1px; display: block; width: 30px; height: 28px; visibility: visible; content: ""; background-color: #fff; border-radius: 28px; transition: left 0.3s ease; } input[type="checkbox"]:checked ~ span:before { background-color: orange; } input[type="checkbox"]:checked ~ span:after { top: 1px; left: 21px; } input[type="checkbox"]:hover ~ span:before { background-color: #1194d1; } input[type="checkbox"]:not(:checked):hover ~ span:before { background-color: #afbec9; } input[type="checkbox"]:focus ~ span:before { outline: none; box-shadow: 0 0 0 4px red; } </style> </head> <body> <div class="toggle"> <label for="toggle1">Check the box to toggle me</label> <input type="checkbox" id="toggle1" /> <span></span> </div> </body> </html>
Apart from :focus, there are two more pseudo-classes that are associated with the :focus pseudo-class. They are listed in the table given below:
sr.no | pseudo-class | description |
---|---|---|
1 | :focus-visible | Focus is made evident on an element and it is shown to the user. |
2 | :focus-within | sets focus on the element that contains a focussed element. |
To Continue Learning Please Login