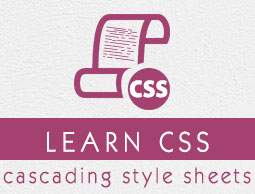
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Combinators
In CSS (Cascading Style Sheets), combinators are symbols or characters that specify the relationship between different HTML elements that you want to style. Combinators help you target elements based on their position and hierarchy within the HTML document.
These combinators allow you to apply styles selectively based on the relationships between elements in your HTML structure, making your CSS more specific and targeted to achieve the desired styling effects.
There are four main types of CSS combinators:
-
Descendant Combinator (space)
-
Child Combinator (>)
-
Adjacent sibling Combinator (+)
-
General sibling Combinator (~)
CSS Combinators - Descendant Combinator (space)
The descendant combinator, often represented by a single space (" "), selects elements that are descendants of specified element.
If an element matches the second selector(descendant) and has an ancestor (parent, parent's parent, etc.) that matches the first selector, then that element will be selected.
For example, if you have an HTML structure like this:
<div> <p>Paragraph 1</p> <p>Paragraph 2</p> </div>
You can target all <p> elements inside the <div> like this:
div p { /* CSS styles */ }
The following code demonstrates the usage of the descendant combinator. In this example, we have a parent element <div> with the class name parent. Inside this parent element, we have multiple <p> elements. We will apply a some styles to the child elements with the class name child.
<html> <head> <title>Descendant Combinator Example</title> <style> .parent { background-color: lightblue; padding: 20px; } .parent p { color: red; font-weight: bold; } </style> </head> <body> <div class="parent"> <h1>This is the parent element</h1> <p>This is a paragraph inside the parent element.</p> <p>This is a paragraph inside the parent element.</p> </div> <div> <p>This is a paragraph outside the parent element.</p> </div> </body> </html>
CSS Combinators - Using List
The following example demonstrates the usage of the descendant combinator for list elements.
<html> <head> <title>Descendant Combinator Example</title> <style> div ul { background-color: lightblue; padding: 10px; } div ul li { color: white; margin-bottom: 5px; } </style> </head> <body> <div> <h2>Fruits</h2> <ul> <li>Apple</li> <li>Banana</li> <li>Orange</li> </ul> </div> </body> </html>
CSS Combinators - Child Combinator (>)
The child combinator, represented by the greater-than symbol (>), selects all elements that are immediate children of a specified element. Using the same HTML structure as above, you can target only the immediate child <p> elements like this:
div > p { /* CSS styles */ }
The following example demonstrates the usage of the child selector(>). In this example, we have a parent element <div> with the class name parent. Inside the parent element, we have multiple <p> elements. We want to apply a specific style to the child elements with the class name child.
<html> <head> <title>Child Combinator Example</title> <style> .parent > .child { color: blue; } </style> </head> <body> <div class="parent"> <p>This is the parent element.</p> <p class="child">This is the first child element.</p> <p class="child">This is the second child element.</p> <p>This is the third child element.</p> <p>This is the fourth child element.</p> </div> <div> <p>This is another paragraph.</p> </div> </body> </html>
The following example demonstrates how the child combinator selector can be used to target specific elements that are direct children of a parent element, allowing for more precise styling and customization.
<html> <head> <title>Child Combinator Example</title> <style> div > span { color: red; } ul > li { font-weight: bold; } </style> </head> <body> <div> <span>This text will be red.</span> <span>This text will not be affected.</span> </div> <ul> <li>This list item will have bold font-weight.</li> <li>This list item will also have bold font-weight.</li> </ul> </body> </html>
CSS Combinators - Adjacent sibling Combinator (+)
The adjacent sibling combinator, represented by the plus sign (+), selects an element that is immediately preceded by a specified element. For example, if you have the following HTML:
<h2>Heading 1</h2> <p>Paragraph 1</p> <h2>Heading 2</h2> <p>Paragraph 2</p>
You can target the <p> element that directly follows a <h2> like this:
h2 + p { /* CSS styles */ }
The following code demonstrates the usage of the adjacent sibling selector(+). In this example we are targeting the paragraphs that directly follow <h2> elements.
<html> <head> <style> h2 + p { color: red; } </style> </head> <body> <div> <h2>Example of Adjacent Sibling Combinator</h2> <p>This is the first paragraph.</p> <p>This is the second paragraph.</p> </div> <div> <p>This is the third paragraph.</p> <p>This is the fourth paragraph.</p> </div> </body> </html>
Here is another example demonstrating the usage of the adjacent sibling selector(+).
div + span: This selects the span element that immediately follows a div element. It sets the color of the span text to red.
span + button: This selects the button element that immediately follows a span element. It sets the background color of the button to yellow.
button + ul: This selects the ul element that immediately follows a button element. It changes the list-style-type of the unordered list to square bullets.
<html> <head> <style> div + span { color: red; } span + button { background-color: yellow; } button + ul { list-style-type: square; } </style> </head> <body> <div>This is a div element.</div> <span>This is a span element.</span> <button>This is a button element.</button> <ul> <li>List item 1</li> <li>List item 2</li> <li>List item 3</li> </ul> </body> </html>
CSS Combinators - General sibling Combinator (~)
TThe general sibling combinator, represented by the tilde symbol (~), selects all elements that are siblings of a specified element. It's similar to the adjacent sibling combinator but doesn't require elements to be immediately adjacent. For instance:
<h2>Heading 1</h2> <p>Paragraph 1</p> <h2>Heading 2</h2> <p>Paragraph 2</p>
You can target all <p> elements that are siblings of </h2> like this:
h2 ~ p { /* CSS styles */ }
The following code uses the general sibling selector (div ~ p) to select all <p> elements that are siblings of a <div>. In this case, the selector will target the second and third paragraphs, applying the specified style to them.
<html> <head> <style> div ~ p { color: blue; } </style> </head> <body> <h2>Example of General Sibling Combinator <h2> <div> <p>This paragraph is not affected by the general sibling selector.</p> </div> <p>This paragraph is affected by the general sibling selector.</p> <p>This paragraph is also affected by the general sibling selector.</p> </body> </html>
In the following example a <div> element contains three buttons, followed by three additional buttons outside the <div>. The CSS code selects all the buttons that are siblings of the <div> element using the general sibling combinator (div ~ button).
<html> <head> <title>General Sibling Combinator Example</title> <style> div ~ button { background-color: blue; color: white; padding: 10px; margin: 5px; } </style> </head> <body> <div> <button>Button 1</button> <button>Button 2</button> <button>Button 3</button> </div> <button>Button 4</button> <button>Button 5</button> <button>Button 6</button> </body> </html>
CSS Combinators - Nesting of Combinators
CSS gives the flexibility to utilize any of the selectors along with combinators to target specific sections of your document.
In the following example, we see using comination of combinators to target spectic sections of the HTML document.
<html> <head> <title>Nesting of Combinators</title> <style> /* Parent selector */ .parent { background-color: lightblue; padding: 20px; } /* Child selector */ .parent .child { background-color: lightgreen; padding: 10px; } /* Descendant selector */ .parent span { color: red; } /* Adjacent sibling selector */ .parent + .sibling { font-weight: bold; } /* General sibling selector */ .parent ~ .sibling { text-decoration: underline; } </style> </head> <body> <div class="parent"> <div class="child"> <span>This is a child element</span> </div> </div> <div class="sibling">This is a sibling element</div> </body> </html>