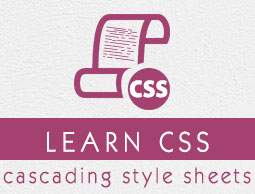
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Lists
Lists are useful as they present the information in a structured and organized manner. Lists improve the readability and comprehension of content on a web page. So, if the content is listed, it is easy to follow.
Lists are commonly used to display items, steps, options, or any other type of related information that should be presented sequentially or in a group.
This chapter will discuss how to control list type, position, style, etc., using CSS. Before that let us understand how are HTML lists different in the following sections.
HTML Lists
HTML provides mainly two kinds of lists - <ol> (ordered list) and <ul> (unordered list).
Name | Description | Syntax |
---|---|---|
Ordered List (<ol>) | Ordered lists are used when the items need to be presented in a specific order. Commonly used for procedures, steps, instructions, or any sequence of information where the order matters. |
<ol class="list"> <li></li> </ol> |
Unordered List (<ul>) | Unordered lists are used when the order of items doesn't matter, and you want to present items as a group. Commonly used for lists of features, benefits, options, or any non-sequential information. |
<ul class="list"> <li></li> </ul> |
Example
Let us see an example of the two kinds of lists (without CSS):
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> h2 {margin-bottom: 5px;} </style> </head> <body> <div> <h2>Ordered list</h2> <ol class="list"> <li>The list is ordered.</li> <li>It is used in cases where we need to follow a sequence.</li> <li>The points are numbered.</li> </ol> <h2>Unordered list</h2> <ul class="list"> <li>The list is unordered.</li> <li>It is used in cases where we need not follow a sequence.</li> <li>The points are bulleted.</li> </ul> </div> </body> </html>
Lists - CSS Properties
CSS provides a set of properties that can be applied to any list, which are as follows:
The list-style-type allows you to control the shape or appearance of the marker.
The list-style-position specifies whether a long point that wraps to a second line should align with the first line or start underneath the start of the marker.
The list-style-image specifies an image for the marker rather than a bullet point or number.
The list-style serves as shorthand for the preceding properties.
Item markers for lists or Bullet Styles
To change the type of marker used for a list’s items, property list-style-type can be used. The same property can be used for both ordered and unordered lists.
Let us see an example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> h2 { margin-bottom: 5px; } ol.o1 { list-style-type: lower-roman; } ol.o2 { list-style-type: upper-alpha; } ul.u1 { list-style-type:square; } ul.u2 { list-style-type: circle; } </style> </head> <body> <div> <h2>Ordered list - Item markers</h2> <ol class="o1"> <li>The item marker is lower-roman.</li> <li>It is used in cases where we need to follow a sequence.</li> </ol> <ol class="o2"> <li>The item marker is upper-alpha.</li> <li>It is used in cases where we need to follow a sequence.</li> </ol> <h2>Unordered list - Item markers</h2> <ul class="u1"> <li>The item marker is square.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> <ul class="u2"> <li>The item marker is circle.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> </div> </body> </html>
Following table lists possible values that can be used for property list-style-type:
Value | Description | Example |
---|---|---|
none | NA | NA |
disc (default) | A filled-in circle | |
circle | An empty circle | |
square | A filled-in square | |
decimal | Number | 1, 2, 3, 4, 5, ... |
decimal-leading-zero | 0 before the number | 01, 02, 03, 04, 05, ... |
lower-alpha | Lowercase alphanumeric characters | a, b, c, d, e, ... |
upper-alpha | Uppercase alphanumeric characters | A, B, C, D, E, ... |
lower-roman | Lowercase Roman numerals | i, ii, iii, iv, v, ... |
upper-roman | Uppercase Roman numerals | I, II, III, IV, V, ... |
lower-greek | The marker is lower-greek | alpha, beta, gamma, ... |
lower-latin | The marker is lower-latin | a, b, c, d, e, ... |
upper-latin | The marker is upper-latin | A, B, C, D, E, ... |
hebrew | The marker is traditional Hebrew numbering | |
armenian | The marker is traditional Armenian numbering | |
georgian | The marker is traditional Georgian numbering | |
cjk-ideographic | The marker is plain ideographic numbers | |
hiragana | The marker is Japanese numbering - hiragana | a, i, u, e, o, ka, ki |
katakana | The marker is Japanese numbering - katakana | A, I, U, E, O, KA, KI |
hiragana-iroha | The marker is Japanese numbering (hiragana-iroha) | i, ro, ha, ni, ho, he, to |
katakana-iroha | The marker is Japanese numbering (katakana-iroha) | I, RO, HA, NI, HO, HE, TO |
The list-style-type property, as well as all other list-related properties, can be applied only to an element that has a display of list-item, but CSS doesn’t distinguish between ordered and unordered list items. User agent may prohibit ordered markers from being applied to unordered lists, and vice versa. You can’t count on this, so be careful.
Item list marker - an image (Using a custom bullet image)
You might prefer to use an image as an item list marker. The list-style-image property can be used to to specify an image as an item list marker.
You can use your own bullet style. If no image is found, then default bullets are returned.
Syntax
ul { list-style-image: url('URL') }
Let us see an example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> h2 { margin-bottom: 5px; } div { padding: 5px; margin-left: 100px;} ul { list-style-image: url('/images/bullet.gif') } </style> </head> <body> <div> <h2>Unordered list - Image as item marker</h2> <ul> <li>The item marker is square.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> <ul> <li>The item marker is circle.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> </div> </body> </html>
Points to remember:
It is advisable to provide an alternative for an image as list marker, so that in case of image not getting loaded or gets corrupted, the user is having a fallback option.
Syntax:
ul { list-style-image: url('URL'); list-style-type: circle / square; }
Syntax:
ul { list-style-image: url('URL'); list-style-type: circle / square; } ul ul { list-style-image: none; }
The above points are demonstrated in the following example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> h2 { margin-bottom: 5px; } div { padding: 5px; margin-left: 100px;} ul { list-style-image: url('/images/bullet.gif') } ul ul { list-style-image: none; } </style> </head> <body> <div> <h2>Unordered list - Image as item marker</h2> <ul> <li>The item marker is an image.</li> <li>It is used in cases where we need use images. <ul> <li>The item marker is a circle.</li> <li>this demonstrates skipping image marker for sub item list.</li> </ul> </li> </ul> </div> </body> </html>
The list item marker - Position or Bullet position
The list-style-position property indicates whether the marker should appear inside or outside of the box containing the bullet points. It can have one of the following values −
Sr.No. | Value | Description |
---|---|---|
1 | none | NA |
2 | inside | If the text goes onto a second line, the text will wrap underneath the marker. It will also have proper indentation. |
3 | outside | If the text goes onto a second line, the text will be aligned with the start of the first line (to the right of the bullet). |
4 | inherit | Inherits the property of the parent list. |
Let us see an example below with the padding removed from the lists and a left red border added:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> body { padding-left: 50px; } ul:nth-of-type(1) { list-style-position: inside; padding: 0; border-left: solid 2px red; } ul:nth-of-type(2) { list-style-position: outside; padding: 0; border-left: solid 2px red; } </style> </head> <body> <h2>List style position</h2> <div> <ul style = "list-style-type:circle; list-style-position:outside;"> <li>The item marker is circle, with style position as outside. Let us see where the bullet is lying.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> </div> <div> <ul style = "list-style-type:square; list-style-position:inside;"> <li>The item marker is square, with style position as inside. Let us see where the bullet is lying.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> </div> </body> </html>
list-style - Shorthand Property
The list-style allows you to specify all the three list properties into a single expression.
Following are the values that list-style can hold:
<list-style-type>
<list-style-image>
<list-style-position>
Points to remember:
The order of the values passed to the list-style is not fixed.
Any of the three values can be omitted.
If any of the value(s) is missing, it will be filled by the default value for the same. But there has to be minimum one value passed.
Let us see an example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> div { border: 2px solid; width: 500px;} </style> </head> <body> <h2>List style - shorthand</h2> <h3>Three values passed</h3> <ul style = "list-style: url('C:/WORK/CSS/tp-css/images/try-it.jpg') circle outside;"> <li>The item marker is an image, position as outside. In absence of image, the marker will be a circle. Try not loading the image and see the type as circle.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> <h3>Two values passed</h3> <ul style = "list-style: square inside"> <li>The item marker is square, with style position as inside and no image.</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> <h3>One value passed</h3> <ul style = "list-style: disc"> <li>The item marker is disc, with style position as outside (default) and no image (default - none).</li> <li>It is used in cases where we need not follow a sequence.</li> </ul> </body> </html>
Controlled list counting
At time we might want to count differently on an ordered list — e.g., starting from a number other than 1, or counting backwards, or counting in steps of more than 1. HTML and CSS have some tools to help you here.
start
The start attribute allows you to start the list counting from a number other than 1 as demonstared in the following example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <h2>Controlled counting using start</h2> <ol start="4"> <li>Java.</li> <li>HTML.</li> <li>CSS.</li> <li>React.</li> </ol> </body> </html>
reversed
The reversed attribute will start the list counting reverse or down instead of up as demonstared in the following example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <h2>Controlled counting using start</h2> <ol start="4" reversed> <li>Java.</li> <li>HTML.</li> <li>CSS.</li> <li>React.</li> </ol> </body> </html>
value
The value attribute allows you to set your list items to specific numerical values, as demonstared in the following example:
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <h2>Controlled counting using start</h2> <ol start="4" reversed> <li value="2">Java.</li> <li value="3">HTML.</li> <li value="1">CSS.</li> <li value="4">React.</li> </ol> </body> </html>
Styling lists with colors
We can make lists look more stylish and colorful using some styling as demonstared in the following example. As we see any styling added to the <ul> or <ol> tag will affect the entire list, whereas the addition to the individual <li> tag will affect only the items of the corresponding list.
<!DOCTYPE html> <html> <head> <title>CSS - Lists</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> ol{ list-style: upper-latin; background: Aquamarine; padding:20px; } ol li{ background: silver; padding:10px; font-size:20px; margin:10px; } ul{ list-style: square inside; background: teal; padding:20px; } ul li{ background: olive; color:white; padding:10px; font-size:20px; margin:10px; } </style> </head> <body> <h2>Controlled counting using start</h2> <ol> <li>Java.</li> <li>HTML.</li> <li>CSS.</li> <li>React.</li> </ol> <ul> <li>Java.</li> <li>HTML.</li> <li>CSS.</li> <li>React.</li> </ul> </body> </html>