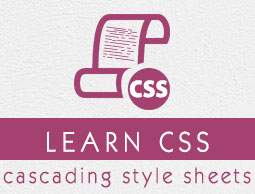
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Positioning
CSS Positioning helps to manipulate position of any element in a web page. In this tutorial we will learn position property and values associated with it.
Table of Contents
What is CSS Position?
In CSS position property is used to create floating elements, floating sidebar, and other interactive features by adjusting position of elements in webpage.
Along with position property, other properties like top, bottom, right, and left are used to control its exact position on the page. They specify the offsets of an element from its edges. We will see examples for those in this tutorial.
Syntax
Following are possible values for css position.
position: static | relative | absolute | fixed | sticky;
Static Positioned Element
When you use the position: static property, the element will be positioned normally in the document flow. The left, right, top, bottom, and z-index properties will not affect the element. This is the default value for position property.
Example
In this example we defined two static positioned div elements and one relative positioned element. See the difference.
<!DOCTYPE html> <html lang="en"> <head> <style> .container { padding: 20px; border: 1px solid #ccc; } .static-element { position: static; background-color: lightblue; padding: 10px; margin: 10px 0; } .non-static-element { position: relative; top: 20px; background-color: lightgreen; padding: 10px; } </style> </head> <body> <div class="container"> <div class="static-element"> This is a static element (default position). </div> <div class="non-static-element"> This element is not static and is moved 20px down. </div> <div class="static-element"> This is another static element (default position). </div> </div> </body> </html>
Relative Positioned Elements
CSS position: relative property positions the elements relative to their original position in the page. You can use the left, right, top, and bottom properties to move the element around, but it will still take up space in the document flow.
Example
In this example we defined one static positioned div element and one relative positioned element. See the difference.
<!DOCTYPE html> <html lang="en"> <head> <style> *{ padding: 15px; } .container { border: 1px solid #ccc; } .static-element { position: static; background-color: lightblue; } .relative-element { position: relative; top: 20px; left: 30px; background-color: lightgreen; } .normal-flow { background-color: lightcoral; margin: 10px 0; } </style> </head> <body> <div class="container"> <div class="static-element"> This is a static element (default position). </div> <div class="relative-element"> This element is relatively positioned 20px down and 30px right. </div> <div class="normal-flow"> This element is in the normal document flow, after the relative element. </div> </div> </body> </html>
Absolutely Positioned Elements
An element with position: absolute is taken out of the document flow and positioned relative to its nearest positioned ancestor (if any). If there is no positioned ancestor, then the element is positioned relative to the viewport.
You can use top, right, bottom, and left properties to specify the position of the element relative to its containing block.
Example
This example show use of position absolute
<!DOCTYPE html> <html lang="en"> <head> <style> *{ padding: 15px; } .container { /* This makes container positioned ancestor */ position: relative; width: 70%; height: 200px; border: 1px solid #ccc; } .static-element { position: static; background-color: lightblue; padding: 10px; margin: 10px 0; } .absolute-element { position: absolute; /* 50px from top of nearest positioned ancestor */ top: 50px; /* 30px from left of nearest positioned ancestor */ left: 30px; background-color: lightgreen; padding: 10px; } </style> </head> <body> <div class="container"> <div class="static-element"> This is a static element (default position). </div> <div class="absolute-element"> This element is absolutely positioned 30px from top and 50px from left. </div> </div> </body> </html>
Position Fixed Element
In CSS position: fixed property is used to make element stay in the same place on the screen even when the user scrolls. You can then use the left, right, top, and bottom properties to position the element where you want it.
Example
In this example you can see that paragraph element remain there when you scroll down.
<html> <head> <style> div { width: 100%; height: 500px; background-color: lightgrey; overflow: auto; padding: 15px; } .fixed-position { position: fixed; top: 50%; left: 20%; padding: 5px; background-color: white; } </style> </head> <body> <div> <p>You can Scroll down...</p> <p class="fixed-position"> Tutorialspoint CSS Position Fixed </p> <p> White screen will remain at 50% height from top </p> </div> </body> </html>
Sticky Positioned Element
In CSS position: sticky property is used to make any element stay at the top of container even when the user scrolls. You can then use the left, right, top, and bottom properties to position the element where you want it.
The position: sticky property is a combination of the position: relative and position: fixed properties
Example
In this example you can see that when you scroll, fixed move to top
<html> <head> <style> div { width: 100%; height: 500px; background-color: lightgrey; overflow: auto; padding: 15px; } .sticky-position { position: sticky; top: 50%; left: 20%; padding: 5px; background-color: white; } </style> </head> <body> <div> <p>You can Scroll down...</p> <p class="sticky-position"> TutorialsPoint CSS Position Fixed </p> <p> White screen will stick at top of screen when you scroll down. </p> </div> </body> </html>
Positioning Text in an Image
In following example, you can use the position: absolute property to position text in different directions inside an image. The text elements are positioned at the top left, top right, bottom left, and bottom right corners.
Example
In the following example we have positioned text on an image.
<html> <head> <style> .container { position: relative; border: 2px solid #ef2c2c; } .center { position: absolute; top: 45%; width: 100%; text-align: center; } .top-left { position: absolute; top: 12px; left: 30px; } .top-right { position: absolute; top: 12px; right: 30px; } .bottom-left { position: absolute; bottom: 12px; left: 30px; } .bottom-right { position: absolute; bottom: 12px; right: 30px; } img { width: 100%; opacity: 0.7; } </style> </head> <body> <div class="container"> <img src="/css/images/red-flower.jpg" width="1000px" height="350px"> <h3 class="center"> Text at Center </h3> <h3 class="top-left"> Text at Top Left </h3> <h3 class="top-right"> Text at Top Right </h3> <h3 class="bottom-left"> Text at Bottom Left</h3> <h3 class="bottom-right"> Text at Bottom Right </h3> </div> </body> </html>
CSS Position Related Properties
Following is the list of all the CSS properties related to position:
Property | Description | Example |
---|---|---|
bottom | Used with the position property to place the bottom edge of an element. | |
clip | Sets the clipping mask for an element. | |
left | Used with the position property to place the left edge of an element. | |
overflow | Determines how overflow content is rendered. | |
right | Used with the position property to place the right edge of an element. | |
top | Sets the positioning model for an element. | |
vertical-align | Sets the vertical positioning of an element. | |
z-index | Sets the rendering layer for the current element. |