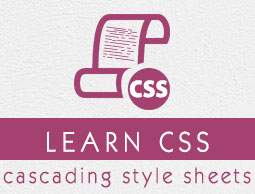
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Atrribute Selector Property
Description
CSS attribute selectors allow you to select HTML elements based on the presence or value of one or more attributes. They are a powerful way to target specific elements in your HTML markup. Attribute selectors are enclosed in square brackets [] and can take various forms.
The following sections discusses some common ways to use attribute selectors:
CSS [attribute] Selector
This selector selects elements that have specified attribute, regardless of its value.
Syntax
[attribute]
Example
Following is an example to select all the HTML elements with a "data-toggle" attribute
<html> <head> <style> [data-toggle] { background: yellow; color: red; } </style> </head> <body> <h2>CSS [attribute] Selector</h2> <div data-toggle="yes">The div with data-toggle attribute</div> <div>The div without data-toggle attribute</div> <p data-toggle="no">A paragraph with data-toggle attribute</p> <p>A paragraph without data-toggle attribute</p> </body> </html>
CSS [attribute="value"] Selector
This selector selects elements that have a specific attribute with a specific value.
Syntax
[attribute="value"]
Example
This selector selects all elements with a 'data-toggle' attribute whose value is set to 'yes'.
<html> <head> <style> [data-toggle="yes"] { background: yellow; color: red; } </style> </head> <body> <h2>CSS [attribute="value"] Selector</h2> <div data-toggle="yes">The div with data-toggle attribute</div> <div>The div without data-toggle attribute</div> <p data-toggle="no">A paragraph with data-toggle attribute</p> <p>A paragraph without data-toggle attribute</p> </body> </html>
CSS [attribute*="value"] Selector
This selector selects elements that have a specific attribute with a value containing a specific substring.
Syntax
[attribute*="value"]
Example
This selector selects all the elements with a "src" attribute which contain a "images" in the path:
<html> <head> <style> [src*="images"] { border: 2px dashed red; } </style> </head> <body> <h2>CSS [attribute*="value"] Selector</h2> <img alt="Logo" src = "/css/images/logo.png" /> </body> </html>
CSS [attribute^="value"] Selector
This selector selects elements that have a specific attribute with a value that starts with a specific string.
Syntax
[attribute^="value"]
Example
This selector selects all elements with a "href" attribute starting with "https://"
<html> <head> <style> [href^="https"] { background: yellow; text-decoration:none; color:red; } </style> </head> <body> <h2>CSS [attribute^="value"] Selector</h2> <p><a href="https://www.tutorialspoint.com">HTTPS Link</a></p> <p><a href="http://www.tutorialspoint.com">HTTP Link</a></p> </body> </html>
CSS [attribute$="value"] Selector
This selector selects elements that have a specific attribute with a value that ends with a specific string.
Syntax
[attribute$="value"]
Example
This selector selects all elements with a "src" attribute which ends with ".png"
<html> <head> <style> [src$=".png"] { border: 2px dashed red; } </style> </head> <body> <h2>CSS [attribute$="value"] Selector</h2> <img alt="Logo" src = "/css/images/logo.png" /> </body> </html>
CSS [attribute|="value"] Selector
This selector select elements that have a specific attribute with a value that begins with a specified substring followed by a hyphen (-). This selector is often used for selecting elements with language-specific attributes, such as lang attributes, which often use hyphens to denote language subcodes.
Syntax
[attribute|="value"]
Example
This selector selects all the elements with a "lang" attribute that starts with "en" followed by a hyphen:
<html> <head> <style> [lang|="en"] { border: 2px dashed red; } </style> </head> <body> <h2>CSS [attribute|="value"] Selector</h2> <div lang="en">Hello World in English!</div> <div lang="fr">Bonjour tout le monde en français!</div> <div lang="es">Hola Mundo en español!</div> </body> </html>
CSS [attribute~="value"] Selector
This selector is used to select elements that have a specific attribute with a value containing a specified word. The word should be a standalone word, surrounded by spaces or at the beginning or end of the attribute value.
Syntax
[attribute~="value"]
Example
This selector select all elements with a "class" attribute containing the word "beautifyme"
<html> <head> <style> [class~="beautifyme"] { background-color:lightblue; border:2px solid red; } </style> </head> <body> <h2>CSS [attribute|="value"] Selector</h2> <div class="beautifyme">div with <b>beautifyme</b> class</div> <div class="beautifyme1">div with <b>beautifyme1</b> class</div> <div class="beautifyme-2">div with <b>beautifyme-2</b> class</div> </body> </html>
Attribute Selectors For href Links
You can style elements based on their href attribute is a common attribute used on <a> elements to specify the URL that the link points to.
Here is an example −
<html> <head> <style> ul { list-style: none; } a[href] { color: rgb(11, 11, 231); } a[href="css_backgrounds.htm"] { color: rgb(224, 152, 18); } a[href~="css_colors.htm"] { color: rgb(51, 255, 0); } a[href|="css_padding.htm"] { color: rgb(0, 255, 255); } a[href^="css_margins.htm"] { color: rgb(255, 0, 55); } a[href$="css_lists.htm"] { color: rgb(255, 230, 0); } a[href*="css_borders.htm"] { color: rgb(112, 108, 108); } </style> </head> <body> <ul> <li> <a href="css_text.htm">CSS Text</a></li> <li><a href=".htm">CSS Background</a></li> <li><a href="css_colors.htm">CSS Color</a></li> <li><a href="css_padding.htm">CSS Padding</a></li> <li><a href="css_margins.htm">CSS Margin</a></li> <li><a href="css_lists.htm">CSS List</a></li> <li><a href="css_borders.htm">CSS Borders</a></li> </ul> </body> </html>
Attribute Selectors For Inputs
Attribute selectors can be used to select input elements based on specific criteria, such as their type, name, value, or other attributes.
Here is an example −
<html> <head> <style> input { display: block; margin: 10px; } input[type] { border: 1px solid #e0dd29; } input[placeholder="Password"] { border: 1px solid #f00; } input[name|="emailid"] { background-color: rgb(236, 178, 233); } input[type~="submit"] { background-color: rgb(88, 241, 88); padding: 10px; } input[value^="But"] { background-color: rgb(236, 149, 68); padding: 10px; } input[type$="box"] { border-radius: 5px; height: 50px; width: 20px; } input[type*="adi"] { height: 50px; width: 20px; } </style> </head> <body> <input type="text" placeholder="Username"> <input type="password" placeholder="Password"> <input type="email" placeholder="Email" name="emailid"> <input type="submit" placeholder="Submit"> <input type="button" value="Button"> <input type="checkbox" placeholder="Checkbox"> <input type="radio" placeholder="Radio"> </body> </html>
Attribute Selectors For Title
To style elements based on the title attribute, you can use the CSS attribute selector title to style elements that have a title attribute containing a specific value.
Here is an example −
<html> <head> <style> span { display: block; margin: 10px; padding: 5px; } span[title] { border: 2px solid rgb(231, 40, 40); background-color: rgb(109, 177, 236); } span[title="CSS Background"] { border: 2px solid rgb(231, 40, 40); background-color: rgb(250, 163, 192); } span[title|="CSS Border"] { border: 2px solid rgb(231, 40, 40); background-color: rgb(245, 248, 79); } span[title^="Mar"] { border: 2px solid rgb(231, 40, 40); background-color: rgb(255, 147, 23); } span[title$="ght"] { border: 2px solid rgb(231, 40, 40); background-color: rgb(102, 201, 240); } span[title*="CSS Width"] { border: 2px solid rgb(231, 40, 40); background-color: rgb(191, 14, 235); } </style> </head> <body> <span title="CSS Text">A text refers to a piece of written or printed information in the form of words or characters.</span> <span title="CSS Background">You can set backgrounds of various HTML elements.</span> <span title="CSS Border"> border property is used to create a border around an element.</span> <span title="Margin">Setting up a margin around an HTML element.</span> <span title="Height">The height property sets the height of an element's content area.</span> <span title="CSS Width">The width sets the width of an element's content area.</span> </body> </html>
Attribute Selectors For Language
You can use the lang attribute to select elements based on their language. The lang attribute specifies the language of the text contained within an element.
Here is an example −
<html> <head> <style> div[lang] { color: red; } div[lang="fr"] { color: blue; } div[lang~="es"] { color: green; } div[lang|="de"] { color: orange; } div[lang^="it"] { color: purple; } div[lang$="ja"] { color: brown; } div[lang*="zh"] { color: teal; } </style> </head> <body> <div lang="en">Hello World in English!</div> <div lang="fr">Bonjour tout le monde en français!</div> <div lang="es">Hola Mundo en español!</div> <div lang="ja">こんにちは、日本語で世界!</div> <div lang="de">Hallo Welt auf Deutsch!</div> <div lang="it">Ciao Mondo in italiano!</div> <div lang="zh">你好世界,中文!</div> </body> </html>
CSS Multiple Attribute Selectors
CSS multiple attribute selectors allow you to select elements based on multiple attribute values. It is use to target specific element that meet multiple criteria.
Here is an example −
<html> <head> <style> ul { list-style: none; } a[href] { color: rgb(231, 11, 194); } a[href="css_backgrounds.htm"][href$=".htm"] { color: rgb(224, 152, 18); } a[href="css_colors.htm"] { color: rgb(51, 255, 0); } </style> </head> <body> <ul> <li><a href="css_text.htm">CSS Text</a></li> <li><a href="css_backgrounds.htm">CSS Background</a></li> <li><a href="css_colors.htm">CSS Color</a></li> </ul> </body> </html>
CSS Attribute Selectors With Sibling Combinator
CSS attribute selectors and sibling combinators can be used together to select specific elements based on their attributes and their relationship to other elements.
General sibling combinator (~) − This combinator selects all sibling elements that follow a specified element, but are not directly adjacent to it.
Syntax
selector1 ~ selector2 { /* CSS styles */ }
Adjacent sibling combinator (+) − This combinator selects the element that is directly after the specified element.
Syntax
selector1 + selector2 { /* CSS styles */ }
The following example demonstrates that the adjacent sibling combinator (+) selects the paragraph immediately after the heading, while the general sibling combinator (~) selects <div> after the heading −
<html> <head> <style> h2 + p { font-weight: bold; color:blue; } h2 ~ div { color: red; } </style> </head> <body> <h2>CSS Background</h2> <p>You can set backgrounds of various HTML elements.</p> <div> <p>Contrary to popular belief, Lorem Ipsum is not simply random text.</p> </div> <p>There are many variations of passages of Lorem Ipsum available.</p> </body> </html>