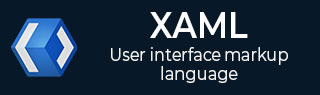
- XAML Tutorial
- XAML - Home
- XAML - Overview
- XAML - Environment Setup
- Writing XAML Aplication On MAC OS
- XAML Vs C# Code
- XAML Vs.VB.NET
- XAML - Building Blocks
- XAML - Controls
- XAML - Layouts
- XAML - Event Handling
- XAML - Data Binding
- XAML - Markup Extensions
- XAML - Dependency Properties
- XAML - Resources
- XAML - Templates
- XAML - Styles
- XAML - Triggers
- XAML - Debugging
- XAML - Custom Controls
- XAML Useful Resources
- XAML - Quick Guide
- XAML - Useful Resources
- XAML - Discussion
XAML - RichEditBox
A RichEditBox is a rich text editing control that supports formatted text, hyperlinks, and other rich content. WPF projects do not support this control. So it will be implemented in Windows App. The hierarchical inheritance of RichEditBox class is as follows −
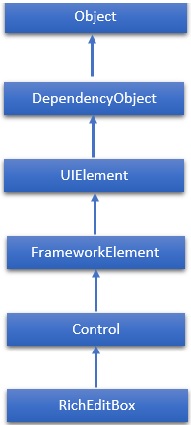
Properties
Sr.No. | Property & Description |
---|---|
1 | AcceptsReturn Gets or sets a value that indicates whether the RichEditBox allows and displays the newline or return characters when the ENTER or RETURN keys are pressed. |
2 | AcceptsReturnProperty Identifies the AcceptsReturn dependency property. |
3 | DesiredCandidateWindowAlignment Gets or sets a value that indicates the preferred alignment of the Input Method Editor (IME). |
4 | DesiredCandidateWindowAlignmentProperty Identifies the DesiredCandidateWindowAlignment dependency property. |
5 | Document Gets an object that enables access to the text object model for the text contained in a RichEditBox. |
6 | Header Gets or sets the content for the control's header. |
7 | HeaderProperty Identifies the Header dependency property. |
8 | HeaderTemplate Gets or sets the DataTemplate used to display the content of the control's header. |
9 | HeaderTemplateProperty Identifies the HeaderTemplate dependency property. |
10 | InputScope Gets or sets the context for input used by this RichEditBox. |
11 | InputScopeProperty Identifies the InputScope dependency property. |
12 | IsColorFontEnabled Gets or sets a value that determines whether font glyphs that contain color layers, such as Segoe UI Emoji, are rendered in color. |
13 | IsColorFontEnabledProperty Identifies the IsColorFontEnabled dependency property. |
14 | IsReadOnly Gets or sets a value that indicates whether the user can change the text in the RichEditBox. |
15 | IsReadOnlyProperty Identifies the IsReadOnly dependency property. |
16 | IsSpellCheckEnabled Gets or sets a value that indicates whether the text input should interact with a spell check engine. |
17 | IsSpellCheckEnabledProperty Identifies the IsSpellCheckEnabled dependency property. |
18 | IsTextPredictionEnabled Gets or sets a value that indicates whether text prediction features ("autocomplete") are enabled for this RichEditBox. |
19 | IsTextPredictionEnabledProperty Identifies the IsTextPredictionEnabled dependency property. |
20 | PlaceholderText Gets or sets the text that is displayed in the control until the value is changed by a user action or some other operation. |
21 | PlaceholderTextProperty Identifies the PlaceholderText dependency property. |
22 | PreventKeyboardDisplayOnProgrammaticFocus Gets or sets a value that indicates whether the on-screen keyboard is shown when the control receives focus programmatically. |
23 | PreventKeyboardDisplayOnProgrammaticFocusProperty Identifies the PreventKeyboardDisplayOnProgrammaticFocus dependency property. |
24 | SelectionHighlightColor Gets or sets the brush used to highlight the selected text. |
25 | SelectionHighlightColorProperty Identifies the SelectionHighlightColor dependency property. |
26 | TextAlignment Gets or sets a value that indicates how text is aligned in the RichEditBox. |
27 | TextAlignmentProperty Identifies the TextAlignment dependency property. |
28 | TextReadingOrder Gets or sets a value that indicates how the reading order is determined for the RichEditBox. |
29 | TextReadingOrderProperty Identifies the TextReadingOrder dependency property. |
30 | TextWrapping Gets or sets a value that indicates how text wrapping occurs if a line of text extends beyond the available width of the RichEditBox. |
31 | TextWrappingProperty Identifies the TextWrapping dependency property. |
Events
Sr.No. | Event & Description |
---|---|
1 | CandidateWindowBoundsChanged Occurs when the Input Method Editor (IME) window open, updates, or closes. |
2 | ContextMenuOpening Occurs when the system processes an interaction that displays a context menu. |
3 | Paste Occurs when text is pasted into the control. |
4 | SelectionChanged Occurs when the text selection has changed. |
5 | TextChanged Occurs when content changes in the RichEditBox. |
6 | TextChanging Occurs when the text in the RichEditBox starts to change. |
7 | TextCompositionChanged Occurs when text being composed through an Input Method Editor (IME) changes. |
8 | TextCompositionEnded Occurs when a user stops composing text through an Input Method Editor (IME). |
9 | TextCompositionStarted Occurs when a user starts composing text through an Input Method Editor (IME). |
Methods
Sr.No. | Method & Description |
---|---|
1 | OnManipulationCompleted Called before the ManipulationCompleted event occurs. (Inherited from Control) |
2 | OnManipulationDelta Called before the ManipulationDelta event occurs. (Inherited from Control) |
3 | OnManipulationInertiaStarting Called before the ManipulationInertiaStarting event occurs. (Inherited from Control) |
4 | OnManipulationStarted Called before the ManipulationStarted event occurs. (Inherited from Control) |
5 | OnManipulationStarting Called before the ManipulationStarting event occurs. (Inherited from Control) |
6 | OnMaximumChanged Called when the Maximum property changes. (Inherited from RangeBase) |
7 | OnMinimumChanged Called when the Minimum property changes. (Inherited from RangeBase) |
8 | OnValueChanged Fires the ValueChanged routed event. (Inherited from RangeBase) |
9 | SetBinding Attaches a binding to a FrameworkElement, using the provided binding object. (Inherited from FrameworkElement) |
10 | SetValue Sets the local value of a dependency property on a DependencyObject. (Inherited from DependencyObject) |
11 | StartDragAsync Initiates a drag-and-drop operation. (Inherited from UIElement) |
12 | UnregisterPropertyChangedCallback Cancels a change notification that was previously registered by calling RegisterPropertyChangedCallback. (Inherited from DependencyObject) |
Example
The following example shows how to open and save an RTF file in RichEditBox. Here is the XAML code to create and initialize two buttons and a RichEditBox with some properties and events.
<Page x:Class = "XAMLRichEditBox.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local = "using:XAMLRichEditBox" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d"> <Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}"> <Grid Margin = "120"> <Grid.RowDefinitions> <RowDefinition Height="50"/> <RowDefinition/> </Grid.RowDefinitions> <StackPanel Orientation = "Horizontal"> <Button Content = "Open file" Click = "OpenButton_Click"/> <Button Content = "Save file" Click = "SaveButton_Click"/> </StackPanel> <RichEditBox x:Name = "editor" Grid.Row = "1"/> </Grid> </Grid> </Page>
Here is the implementation in C# for different events and file handling −
using System; using System.Collections.Generic; using System.IO; using System.Linq; using System.Runtime.InteropServices.WindowsRuntime; using Windows.Foundation; using Windows.Foundation.Collections; using Windows.Storage; using Windows.Storage.Pickers; using Windows.Storage.Provider; using Windows.UI.ViewManagement; using Windows.UI.Xaml; using Windows.UI.Xaml.Controls; using Windows.UI.Xaml.Controls.Primitives; using Windows.UI.Xaml.Data; using Windows.UI.Xaml.Input; using Windows.UI.Xaml.Media; using Windows.UI.Xaml.Navigation; // The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238 namespace XAMLRichEditBox { /// <summary> /// An empty page that can be used on its own or navigated to within a Frame. /// </summary> public sealed partial class MainPage : Page { public MainPage() { this.InitializeComponent(); } private async void OpenButton_Click(object sender, RoutedEventArgs e) { // Open a text file. Windows.Storage.Pickers.FileOpenPicker open = new Windows.Storage.Pickers.FileOpenPicker(); open.SuggestedStartLocation = Windows.Storage.Pickers.PickerLocationId.DocumentsLibrary; open.FileTypeFilter.Add(".rtf"); Windows.Storage.StorageFile file = await open.PickSingleFileAsync(); if (file != null) { Windows.Storage.Streams.IRandomAccessStream randAccStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read); // Load the file into the Document property of the RichEditBox. editor.Document.LoadFromStream(Windows.UI.Text.TextSetOptions.FormatRtf, randAccStream); } } private async void SaveButton_Click(object sender, RoutedEventArgs e) { if (((ApplicationView.Value != ApplicationViewState.Snapped) || ApplicationView.TryUnsnap())) { FileSavePicker savePicker = new FileSavePicker(); savePicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary; // Dropdown of file types the user can save the file as savePicker.FileTypeChoices.Add("Rich Text", new List <string>() { ".rtf" }); // Default file name if the user does not type one in or select a file to replace savePicker.SuggestedFileName = "New Document"; StorageFile file = await savePicker.PickSaveFileAsync(); if (file != null) { // Prevent updates to the remote version of the file until we //finish making changes and call CompleteUpdatesAsync. CachedFileManager.DeferUpdates(file); // write to file Windows.Storage.Streams.IRandomAccessStream randAccStream = await file.OpenAsync(Windows.Storage.FileAccessMode.ReadWrite); editor.Document.SaveToStream(Windows.UI.Text.TextGetOptions.FormatRtf, randAccStream); // Let Windows know that we're finished changing the file so the // other app can update the remote version of the file. FileUpdateStatus status = await CachedFileManager.CompleteUpdatesAsync(file); if (status != FileUpdateStatus.Complete) { Windows.UI.Popups.MessageDialog errorBox = new Windows.UI.Popups.MessageDialog( "File " + file.Name + " couldn't be saved."); await errorBox.ShowAsync(); } } } } } }
When you compile and execute the above code, it will produce the following output. You can open, edit, and save any RTF file in this application.
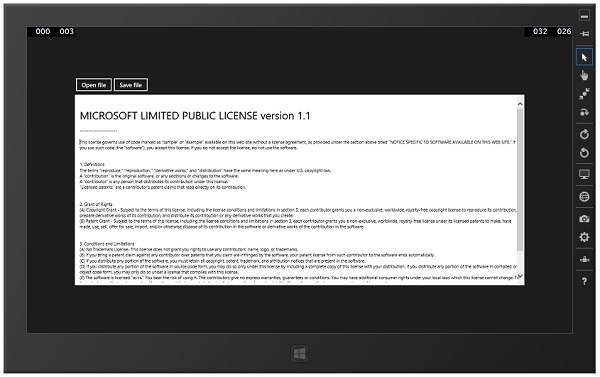
We recommend you to execute the above example code and experiment with some other properties and events.