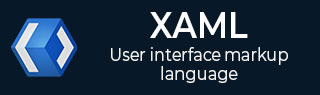
- XAML Tutorial
- XAML - Home
- XAML - Overview
- XAML - Environment Setup
- Writing XAML Aplication On MAC OS
- XAML Vs C# Code
- XAML Vs.VB.NET
- XAML - Building Blocks
- XAML - Controls
- XAML - Layouts
- XAML - Event Handling
- XAML - Data Binding
- XAML - Markup Extensions
- XAML - Dependency Properties
- XAML - Resources
- XAML - Templates
- XAML - Styles
- XAML - Triggers
- XAML - Debugging
- XAML - Custom Controls
- XAML Useful Resources
- XAML - Quick Guide
- XAML - Useful Resources
- XAML - Discussion
XAML - DataGrid
A DataGrid represents a control that displays data in a customizable grid. It provides a flexible way to display a collection of data in rows and columns. The hierarchical inheritance of DataGrid class is as follows −
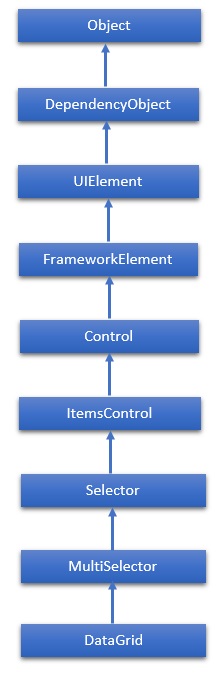
Properties
Sr.No. | Property & Description |
---|---|
1 | AlternatingRowBackground Gets or sets the background brush for use on alternating rows. |
2 | AreRowDetailsFrozen Gets or sets a value that indicates whether the row details can scroll horizontally. |
3 | AutoGenerateColumns Gets or sets a value that indicates whether the columns are created automatically. |
4 | CanUserAddRows Gets or sets a value that indicates whether the user can add new rows to the DataGrid. |
5 | CanUserDeleteRows Gets or sets a value that indicates whether the user can delete rows from the DataGrid. |
6 | CanUserReorderColumns Gets or sets a value that indicates whether the user can change the column display order by dragging column headers with the mouse. |
7 | CanUserResizeColumns Gets or sets a value that indicates whether the user can adjust the width of columns by using the mouse. |
8 | CanUserResizeRows Gets or sets a value that indicates whether the user can adjust the height of rows by using the mouse. |
9 | CanUserSortColumns Gets or sets a value that indicates whether the user can sort columns by clicking the column header. |
10 | ColumnHeaderHeight Gets or sets the height of the column headers row. |
11 | ColumnHeaderStyle Gets or sets the style applied to all column headers in the DataGrid. |
12 | Columns Gets a collection that contains all the columns in the DataGrid. |
13 | ColumnWidth Gets or sets the standard width and sizing mode of columns and headers in the DataGrid. |
14 | CurrentCell Gets or sets the cell that has focus. |
15 | CurrentColumn Gets or sets the column that contains the current cell. |
16 | CurrentItem Gets the data item bound to the row that contains the current cell. |
17 | FrozenColumnCount Gets or sets the number of non-scrolling columns. |
18 | HorizontalScrollBarVisibility Gets or sets a value that indicates how horizontal scroll bars are displayed in the DataGrid. |
19 | IsReadOnly Gets or sets a value that indicates whether the user can edit values in the DataGrid. |
20 | RowBackground Gets or sets the default brush for the row background. |
21 | RowHeight Gets or sets the suggested height for all rows. |
22 | SelectedCells Gets the list of cells that are currently selected. |
Methods
Sr.No. | Methods & Description |
---|---|
1 | BeginEdit Invokes the BeginEdit command, which will place the current cell or row into edit mode. |
2 | CancelEdit Invokes the CancelEditCommand command for the cell or row currently in edit mode. |
3 | ClearDetailsVisibilityForItem Clears the DetailsVisibility property for the DataGridRow that represents the specified data item. |
4 | ColumnFromDisplayIndex Gets the DataGridColumn at the specified index. |
5 | CommitEdit Invokes the CommitEditCommand command for the cell or row currently in edit mode. |
6 | GenerateColumns Generates columns for the specified properties of an object. |
7 | GetDetailsVisibilityForItem Gets the DetailsVisibility property for the DataGridRow that represents the specified data item. |
8 | OnApplyTemplate When overridden in a derived class, is invoked whenever application code or internal processes call ApplyTemplate. (Overrides FrameworkElement.OnApplyTemplate()) |
9 | ScrollIntoView Scrolls the DataGrid vertically to display the row for the specified data item. |
10 | SelectAllCells Selects all the cells in the DataGrid. |
11 | SetDetailsVisibilityForItem Sets the value of the DetailsVisibility property for the DataGridRow that contains the specified object. |
12 | UnselectAllCells Unselects all the cells in the DataGrid. |
Events
Sr.No. | Events & Description |
---|---|
1 | AddingNewItem Occurs before a new item is added to the DataGrid. |
2 | AutoGeneratedColumns Occurs when auto generation of all columns is completed. |
3 | AutoGeneratingColumn Occurs when an individual column is auto-generated. |
4 | BeginningEdit Occurs before a row or cell enters edit mode. |
5 | CellEditEnding Occurs before a cell edit is committed or canceled. |
6 | ColumnDisplayIndexChanged Occurs when the DisplayIndex property on one of the columns changes. |
7 | ColumnHeaderDragCompleted Occurs when the user releases a column header after dragging it by using the mouse. |
8 | ColumnHeaderDragDelta Occurs every time the mouse position changes while the user drags a column header. |
9 | ColumnHeaderDragStarted Occurs when the user begins dragging a column header by using the mouse. |
10 | ColumnReordered Occurs when a column moves to a new position in the display order. |
11 | ColumnReordering Occurs before a column moves to a new position in the display order. |
12 | CopyingRowClipboardContent Occurs after the default row content is prepared. |
13 | CurrentCellChanged Occurs when the value of the CurrentCell property has changed. |
14 | InitializingNewItem Occurs when a new item is created. |
15 | LoadingRow Occurs after a DataGridRow is instantiated, so that you can customize it before it is used. |
16 | LoadingRowDetails Occurs when a new row details template is applied to a row. |
17 | PreparingCellForEdit Occurs when a cell enters edit mode. |
18 | RowDetailsVisibilityChanged Occurs when the visibility of a row details element changes. |
19 | RowEditEnding Occurs before a row edit is committed or canceled. |
20 | SelectedCellsChanged Occurs when the SelectedCells collection changes. |
21 | Sorting Occurs when a column is being sorted. |
22 | UnloadingRow Occurs when a DataGridRow object becomes available for reuse. |
23 | UnloadingRowDetails Occurs when a row details element becomes available for reuse. |
Example
The following example shows how to display data in a DataGrid. Here is the XAML code to create two checkboxes with some properties and events.
<Window x:Class = "DataGrid.MainWindow" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:core = "clr-namespace:System;assembly = mscorlib" xmlns:local = "clr-namespace:DataGrid" Title = "MainWindow" Height = "350" Width = "525"> <Window.Resources> <ObjectDataProvider x:Key = "myEnum" MethodName = "GetValues" ObjectType = "{x:Type core:Enum}"> <ObjectDataProvider.MethodParameters> <x:TypeExtension Type = "local:Party" /> </ObjectDataProvider.MethodParameters> </ObjectDataProvider> </Window.Resources> <Grid> <DataGrid Name = "dataGrid" AlternatingRowBackground = "LightBlue" AlternationCount = "2" AutoGenerateColumns = "False"> <DataGrid.Columns> <DataGridTextColumn Header = "Name" Binding = "{Binding Name}" /> <DataGridTextColumn Header = "Title" Binding = "{Binding Title}" > <DataGridCheckBoxColumn Header = "ReElected?" Binding = "{Binding WasReElected}"/> <DataGridComboBoxColumn Header = "Party" SelectedItemBinding = "{Binding Affiliation}" ItemsSource = "{Binding Source = {StaticResource myEnum}}" /> </DataGrid.Columns> </DataGrid> </Grid> </Window>
Here is the implementation in C# for two different classes.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; namespace DataGrid { /// <summary> /// Interaction logic for MainWindow.xaml /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); dataGrid.ItemsSource = Employee.GetEmployees(); } } public enum Party { Indepentent, Federalist, DemocratRepublican, } }
Here is another Employee class implementation in C#.
public class Employee : INotifyPropertyChanged { private string name; public string Name { get { return name; } set { name = value; RaiseProperChanged(); } } private string title; public string Title { get { return title; } set { title = value; RaiseProperChanged(); } } private bool wasReElected; public bool WasReElected { get { return wasReElected; } set { wasReElected = value; RaiseProperChanged(); } } private Party affiliation; public Party Affiliation { get { return affiliation; } set { affiliation = value; RaiseProperChanged(); } } public static ObservableCollection<Employee> GetEmployees() { var employees = new ObservableCollection<Employee>(); employees.Add(new Employee() { Name = "Ali", Title = "Minister", WasReElected = true, Affiliation = Party.Indepentent }); employees.Add(new Employee() { Name = "Ahmed", Title = "CM", WasReElected = false, Affiliation = Party.Federalist }); employees.Add(new Employee() { Name = "Amjad", Title = "PM", WasReElected = true, Affiliation = Party.DemocratRepublican }); employees.Add(new Employee() { Name = "Waqas", Title = "Minister", WasReElected = false, Affiliation = Party.Indepentent }); employees.Add(new Employee() { Name = "Bilal", Title = "Minister", WasReElected = true, Affiliation = Party.Federalist }); employees.Add(new Employee() { Name = "Waqar", Title = "Minister", WasReElected = false, Affiliation = Party.DemocratRepublican }); return employees; } public event PropertyChangedEventHandler PropertyChanged; private void RaiseProperChanged( [CallerMemberName] string caller = "") { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(caller)); } } }
When you compile and execute the above code, it will produce the following output −
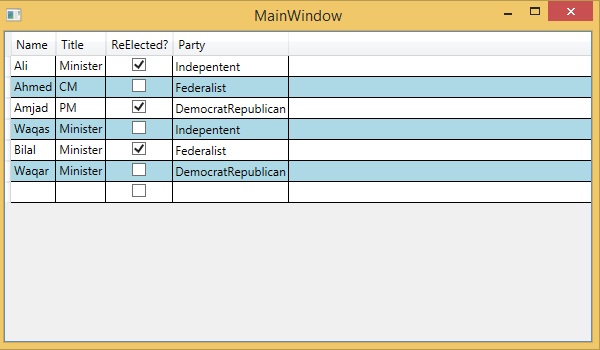
We recommend you to execute the above example code and experiment with some other properties and events.