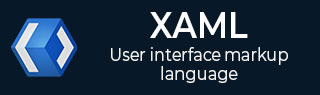
- XAML Tutorial
- XAML - Home
- XAML - Overview
- XAML - Environment Setup
- Writing XAML Aplication On MAC OS
- XAML Vs C# Code
- XAML Vs.VB.NET
- XAML - Building Blocks
- XAML - Controls
- XAML - Layouts
- XAML - Event Handling
- XAML - Data Binding
- XAML - Markup Extensions
- XAML - Dependency Properties
- XAML - Resources
- XAML - Templates
- XAML - Styles
- XAML - Triggers
- XAML - Debugging
- XAML - Custom Controls
- XAML Useful Resources
- XAML - Quick Guide
- XAML - Useful Resources
- XAML - Discussion
XAML - Calendar
Calendar represents a control that enables a user to select a date by using a visual calendar display. It provides some basic navigation facilities using either the mouse or the keyboard. The hierarchical inheritance of Calendar class is as follows −
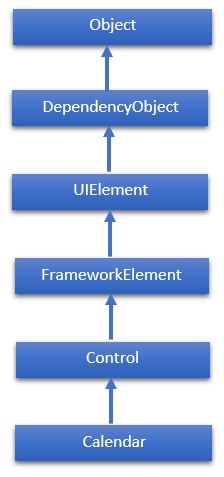
Properties
Sr.No. | Properties & Description |
---|---|
1 | BlackoutDates Gets a collection of dates that are marked as not selectable. |
2 | CalendarButtonStyle Gets or sets the Style associated with the control's internal CalendarButton object. |
3 | CalendarDayButtonStyle Gets or sets the Style associated with the control's internal CalendarDayButton object. |
4 | CalendarItemStyle Gets or sets the Style associated with the control's internal CalendarItem object. |
5 | DisplayDate Gets or sets the date to display. |
6 | DisplayDateEnd Gets or sets the last date in the date range that is available in the calendar. |
7 | DisplayDateStart Gets or sets the first date that is available in the calendar. |
8 | DisplayMode Gets or sets a value that indicates whether the calendar displays a month, year, or decade. |
9 | FirstDayOfWeek Gets or sets the day that is considered the beginning of the week. |
10 | IsTodayHighlighted Gets or sets a value that indicates whether the current date is highlighted. |
11 | SelectedDate Gets or sets the currently selected date. |
12 | SelectedDates Gets a collection of selected dates. |
13 | SelectionMode Gets or sets a value that indicates what kind of selections are allowed. |
Methods
Sr.No. | Method & Description |
---|---|
1 | OnApplyTemplate Builds the visual tree for the Calendar control when a new template is applied. (Overrides FrameworkElement.OnApplyTemplate()) |
2 | ToString Provides a text representation of the selected date. (Overrides Control.ToString()) |
Events
Sr.No. | Event & Description |
---|---|
1 | DisplayDateChanged Occurs when the DisplayDate property is changed. |
2 | DisplayModeChanged Occurs when the DisplayMode property is changed. |
3 | SelectedDatesChanged Occurs when the collection returned by the SelectedDates property is changed. |
4 | SelectionModeChanged Occurs when the SelectionMode changes. |
Example
The following example contains a Calendar control with selections and blackout dates. When you click on any date except the blackout dates, the program will update the title with that date.
Here is the XAML code in which a calendar is created with some properties and a click event.
<Window x:Class = "XAMLCalendar.MainWindow" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" Title = "MainWindow" Height = "350" Width = "604"> <Grid> <StackPanel Orientation = "Horizontal"> <!-- Create a Calendar that displays dates through Januarary 31, 2015 and has dates that are not selectable. --> <Calendar Margin = "20" SelectionMode = "MultipleRange" IsTodayHighlighted = "false" DisplayDate = "1/1/2015" DisplayDateEnd = "1/31/2015" SelectedDatesChanged = "Calendar_SelectedDatesChanged" xmlns:sys = "clr-namespace:System;assembly = mscorlib"> <Calendar.BlackoutDates> <CalendarDateRange Start = "1/2/2015" End = "1/4/2015"/> <CalendarDateRange Start = "1/9/2015" End = "1/9/2015"/> <CalendarDateRange Start = "1/16/2015" End = "1/16/2015"/> <CalendarDateRange Start = "1/23/2015" End = "1/25/2015"/> <CalendarDateRange Start = "1/30/2015" End = "1/30/2015"/> </Calendar.BlackoutDates> <Calendar.SelectedDates> <sys:DateTime>1/5/2015</sys:DateTime> <sys:DateTime>1/12/2015</sys:DateTime> <sys:DateTime>1/14/2015</sys:DateTime> <sys:DateTime>1/13/2015</sys:DateTime> <sys:DateTime>1/15/2015</sys:DateTime> <sys:DateTime>1/27/2015</sys:DateTime> <sys:DateTime>4/2/2015</sys:DateTime> </Calendar.SelectedDates> </Calendar> </StackPanel> </Grid> </Window>
Here is the select event implementation in C#.
using System; using System.Windows; using System.Windows.Controls; using System.Windows.Media; namespace XAMLCalendar { /// <summary> /// Interaction logic for MainWindow.xaml /// </summary> public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } private void Calendar_SelectedDatesChanged(object sender, SelectionChangedEventArgs e) { var calendar = sender as Calendar; // ... See if a date is selected. if (calendar.SelectedDate.HasValue) { // ... Display SelectedDate in Title. DateTime date = calendar.SelectedDate.Value; this.Title = date.ToShortDateString(); } } } }
When you compile and execute the above code, it will display the following screen −
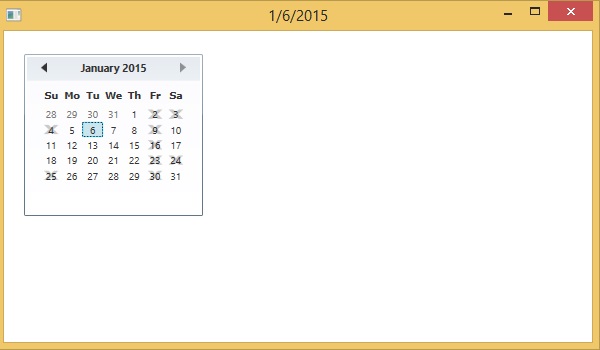
We recommend you to execute the above example code and experiment with some other properties and events.