
- WebAssembly Tutorial
- WebAssembly - Home
- WebAssembly - Overview
- WebAssembly - Introduction
- WebAssembly - WASM
- WebAssembly - Installation
- WebAssembly - Tools to Compile to WASM
- WebAssembly - Program Structure
- WebAssembly - Javascript
- WebAssembly - Javascript API
- WebAssembly - Debugging WASM in Firefox
- WebAssembly - “Hello World”
- WebAssembly - Modules
- WebAssembly - Validation
- WebAssembly - Text Format
- WebAssembly - Convert WAT to WASM
- WebAssembly - Dynamic Linking
- WebAssembly - Security
- WebAssembly - Working with C
- WebAssembly - Working with C++
- WebAssembly - Working with Rust
- WebAssembly - Working with Go
- WebAssembly - Working with Nodejs
- WebAssembly - Examples
- WebAssembly Useful Resources
- WebAssembly - Quick Guide
- WebAssembly - Useful Resources
- WebAssembly - Discussion
WebAssembly - WASM
WebAssembly is also called wasm, which is an improvement to Javascript. It is designed to run inside browsers just like javascript and also with nodejs. You happen to get wasm output, when any high level language like C, C++, Rust is compiled.
Consider the following C program −
int factorial(int n) { if (n == 0) return 1; else return n * factorial(n-1); }
Make use of WasmExplorer, which is available at https://mbebenita.github.io/WasmExplorer/ to get the compiled code as shown below −
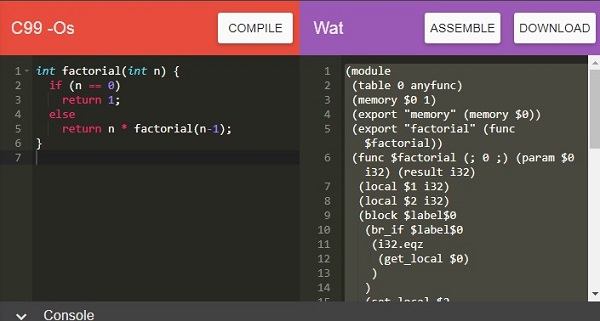
The WebAssembly text format for factorial program is as stated below −
(module (table 0 anyfunc) (memory $0 1) (export "memory" (memory $0)) (export "factorial" (func $factorial)) (func $factorial (; 0 ;) (param $0 i32) (result i32) (local $1 i32) (local $2 i32) (block $label$0 (br_if $label$0 (i32.eqz (get_local $0) ) ) (set_local $2 (i32.const 1) ) (loop $label$1 (set_local $2 (i32.mul (get_local $0) (get_local $2) ) ) (set_local $0 (tee_local $1 (i32.add (get_local $0) (i32.const -1) ) ) ) (br_if $label$1 (get_local $1) ) ) (return (get_local $2) ) ) (i32.const 1) ) )
Using the Wat2Wasm tool, you can view the WASM code, just like how it is mentioned below −
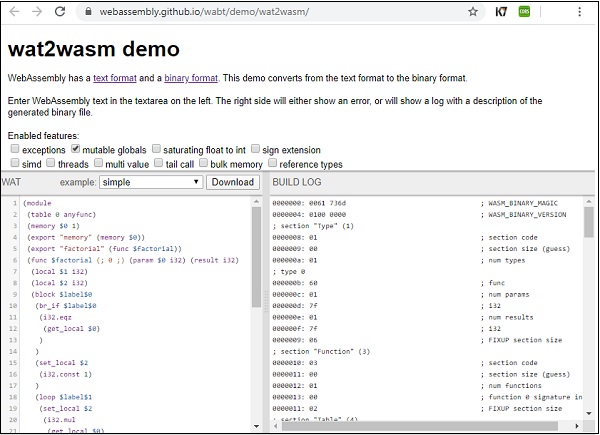
Developers are not supposed to write code in wasm or learn to code in it, as it is mostly generated when you compile high level languages.
Stack Machine Model
In WASM, all the instructions are pushed on to the stack. The arguments are popped and the result is pushed back to the stack.
Consider the following WebAssembly Text format that adds 2 numbers −
(module (func $add (param $a i32) (param $b i32) (result i32) get_local $a get_local $b i32.add ) (export "add" (func $add)) )
The name of the function is $add, it takes in 2 params $a and $b. The result is a type 32-bit integer. The local variables are accessed using get_local and the add operation is performed using i32.add.
The stack representation to add 2 numbers while execution will be as follows −
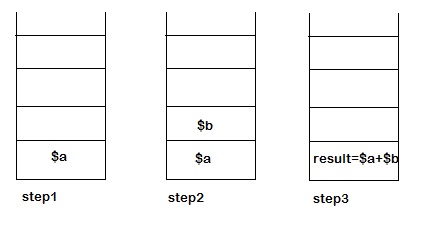
In step 1 − The execution of get_local $a instruction, the first parameters i.e., $a is pushed on the stack.
In step 2 − During execution of get_local $b instruction, the second parameters i.e., $b is pushed on the stack.
In step 3 − The execution of i32.add will pop the elements from the stack and will push the result back to the stack. The value that remains in the end inside the stack is the result of the function $add.