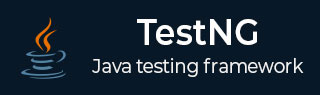
- TestNG Tutorial
- TestNG - Home
- TestNG - Overview
- TestNG - Environment
- TestNG - Writing Tests
- TestNG - Basic Annotations
- TestNG - Execution Procedure
- TestNG - Executing Tests
- TestNG - Suite Test
- TestNG - Ignore a Test
- TestNG - Group Test
- TestNG - Exception Test
- TestNG - Dependency Test
- TestNG - Parameterized Test
- TestNG - Run JUnit Tests
- TestNG - Test Results
- TestNG - Annotation Transformers
- TestNG - Asserts
- TestNG - Parallel Execution
- TestNG - Plug with ANT
- TestNG - Plug with Eclipse
- TestNG - TestNG - vs JUnit
- TestNG Useful Resources
- TestNG - Quick Guide
- TestNG - Useful Resources
- TestNG - Discussion
TestNG - Basic Annotations - Listeners
@Test annotation marks a class or a method as part of the test.
The following is a list of attributes supported by the @Test annotation:
Attribute | Description |
---|---|
alwaysRun |
If set to true, this test method will always be run even if it depends on a method that failed. |
dataProvider |
The name of the data provider for this test method. |
dataProviderClass |
The class where to look for the data provider. If not specified, the data provider will be looked on the class of the current test method or one of its base classes. If this attribute is specified, the data provider method needs to be static on the specified class. |
dependsOnGroups |
The list of groups this method depends on. |
dependsOnMethods |
The list of methods this method depends on. |
description |
The description for this method. |
enabled |
Whether methods on this class/method are enabled. |
expectedExceptions |
The list of exceptions that a test method is expected to throw. If no exception or a different than one on this list is thrown, this test will be marked a failure. |
groups |
The list of groups this class/method belongs to. |
invocationCount |
The number of times this method should be invoked. |
invocationTimeOut |
The maximum number of milliseconds this test should take for the cumulated time of all the invocationcounts. This attribute will be ignored if invocationCount is not specified. |
priority |
The priority for this test method. Lower priorities will be scheduled first. |
successPercentage |
The percentage of success expected from this method |
singleThreaded |
If set to true, all the methods on this test class are guaranteed to run in the same thread. This attribute can only be used at the class level and it will be ignored if used at the method level. |
timeOut |
The maximum number of milliseconds this test should take. |
threadPoolSize |
The size of the thread pool for this method. The method will be invoked from multiple threads as specified by invocationCount. |
Create Test Case Class
Let's see how to use @Test annotation.
Create a java test class, say, SimpleTestClass.java in /work/testng/src.
Add a test method sum() to your test class.
Add an Annotation @Test to method sum().
Following are the SimpleTestClass.java contents.
import org.testng.annotations.Test; public class SimpleTestClass { @Test public void sum() { int sum=0; int a=15; int b=27; sum=a+b; System.out.println("sum="+sum); } }
Create testng.xml
Next, let's create testng.xml file in /work/testng/src, to execute test case(s). This file captures your entire testing in XML. This file makes it easy to describe all your test suites and their parameters in one file, which you can check in your code repository or e-mail to coworkers. It also makes it easy to extract subsets of your tests or split several runtime configurations (e.g., testngdatabase.xml would run only tests that exercise your database).
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd"> <suite name="Suite"> <test thread-count="5" name="Test"> <classes> <class name="SimpleTestClass"/> </classes> </test> <!-- Test --> </suite> <!-- Suite -->
Compile the test case using javac.
/work/testng/src$ javac SimpleTestClass.java TestListener.java
Now, run the testng.xml, which will run the test case defined in <test> tag.
/work/testng/src$ java org.testng.TestNG testng.xml
Verify the output.
sum=42 =============================================== Suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 ===============================================