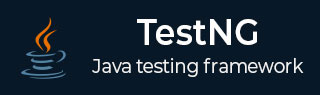
- TestNG Tutorial
- TestNG - Home
- TestNG - Overview
- TestNG - Environment
- TestNG - Writing Tests
- TestNG - Basic Annotations
- TestNG - Execution Procedure
- TestNG - Executing Tests
- TestNG - Suite Test
- TestNG - Ignore a Test
- TestNG - Group Test
- TestNG - Exception Test
- TestNG - Dependency Test
- TestNG - Parameterized Test
- TestNG - Run JUnit Tests
- TestNG - Test Results
- TestNG - Annotation Transformers
- TestNG - Asserts
- TestNG - Parallel Execution
- TestNG - Plug with ANT
- TestNG - Plug with Eclipse
- TestNG - TestNG - vs JUnit
- TestNG Useful Resources
- TestNG - Quick Guide
- TestNG - Useful Resources
- TestNG - Discussion
TestNG - Basic Annotations - Parameters
@Parameters describes how to pass parameters to a @Test method. There are mainly two ways through which we can provide parameter values to test-methods:
Through testng XML configuration file
Through DataProviders
The following is a list of attributes supported by the @Listeners annotation:
Attribute | Description |
---|---|
value |
The list of variables used to fill the parameters of this method. |
The @Parameters annotation can be placed at the following locations:
On any method that already has a @Test, @Before/After or @Factory annotation.
On at most one constructor of your test class. TestNG will invoke this particular constructor with the parameters initialized to the values specified in testng.xml whenever it needs to instantiate your test class. This feature can be used to initialize fields inside your classes to values that will then be used by your test methods.
Create Test Case Class
Let's see how to use @Parameters annotation
Create a java test class, say, TestAnnotationParameter.java in /work/testng/src.
Add a test method testparameter() to your test class.
Add an Annotation @Test to method testparameter().Specify that the parameter firstName of your Java method should receive the value of the XML parameter called first-name. This XML parameter is defined in testng.xml
Following are the TestAnnotationParameter.java contents.
import org.testng.annotations.Test; import org.testng.annotations.Parameters; public class TestAnnotationParameter { @Parameters({ "first-name" }) @Test public void testparameter(String firstName) { System.out.println("Invoked method testparameter with string " + firstName); } }
Create testng.xml
Next, let's create testng.xml file in /work/testng/src, to execute test case(s). This file captures your entire testing in XML. This file makes it easy to describe all your test suites and their parameters in one file, which you can check in your code repository or e-mail to coworkers. It also makes it easy to extract subsets of your tests or split several runtime configurations (e.g., testngdatabase.xml would run only tests that exercise your database).
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd"> <suite name="Suite"> <parameter name="first-name" value="TutorialsPoint"/> <test thread-count="5" name="Test"> <classes> <class name="TestAnnotationParameter"/> </classes> </test> <!-- Test --> </suite> <!-- Suite -->
Compile the test case using javac.
/work/testng/src$ javac TestAnnotationParameter.java
Now, run the testng.xml, which will run the test case defined in <test> tag.
/work/testng/src$ java org.testng.TestNG testng.xml
Verify the output.
Invoked method testparameter with string TutorialsPoint =============================================== Suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 ===============================================